使用Python的 JSON 漂亮打印
JSON(JavaScript Object Notation)是一种基于文本的数据格式,可与许多编程语言互换。它通常用于客户端-服务器应用程序之间的数据传输。通常,传输 JSON 文本的缩小版本以节省带宽。但是,为了调试和分析,需要美化版本或漂亮的 JSON 打印件。本质上,漂亮的打印 JSON 意味着具有适当的缩进、空格和分隔符。本文探讨了如何使用Python漂亮地打印 JSON。
方法
Python有一个名为 json 的内置模块,它允许使用 JSON 数据以及如何将其用于输出功能,如下步骤所示:
- 导入 JSON 包。
- 然后根据场景——
- 从 JSON 文件中读取并漂亮地打印出来,
- 将漂亮打印的 JSON 写入文件,或
- 只是漂亮地打印 JSON字符串数据。
以下是更好地理解这些案例的示例和步骤。
漂亮的打印 JSON 字符串
首先,使用 json.loads() 方法将 JSON String 转换为Python对象。要将此对象转换为漂亮的打印 JSON字符串,请使用 json.dumps() 方法。
句法:
json.dumps(indent,separator)
这个方法有一个参数 indent 来指定空格的数量和一个 separator 参数来指定键和值之间的分隔符。默认情况下,分隔符是键值对之间的逗号,键和值之间的冒号。如果 json.dumps() 的 indent 参数为负数、0 或空字符串,则没有缩进,只插入换行符。默认情况下,缩进为 None 并且数据在一行中表示。
例子:
Python3
# Import required libraries
import json
# Initialize JSON data
json_data = '[ {"studentid": 1, "name": "ABC", "subjects": ["Python", "Data Structures"]}, \
{"studentid": 2, "name": "PQR", "subjects": ["Java", "Operating System"]} ]'
# Create Python object from JSON string data
obj = json.loads(json_data)
# Pretty Print JSON
json_formatted_str = json.dumps(obj, indent=4)
print(json_formatted_str)
Python3
# Import required libraries
import json
data = [{"studentid": 1, "name": "ABC", "subjects": ["Python", "Data Structures"]},
{"studentid": 2, "name": "PQR", "subjects": ["Java", "Operating System"]}]
# Write pretty print JSON data to file
with open("filename.json", "w") as write_file:
json.dump(data, write_file, indent=4)
Python3
# Import required libraries
import json
# Read JSON data from file and pretty print it
with open("filename.json", "r") as read_file:
# Convert JSON file to Python Types
obj = json.load(read_file)
# Pretty print JSON data
pretty_json = json.dumps(obj, indent=4)
print(pretty_json)
输出:
[
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
},
{
"studentid": 2,
"name": "PQR",
"subjects": [
"Java",
"Operating System"
]
}
]
将漂亮的打印 JSON 数据写入文件
要将Python对象作为 JSON 格式的数据写入文件,请使用 json.dump() 方法。像 json.dumps() 方法一样,它有缩进和分隔符参数来编写美化的 JSON。
例子:
蟒蛇3
# Import required libraries
import json
data = [{"studentid": 1, "name": "ABC", "subjects": ["Python", "Data Structures"]},
{"studentid": 2, "name": "PQR", "subjects": ["Java", "Operating System"]}]
# Write pretty print JSON data to file
with open("filename.json", "w") as write_file:
json.dump(data, write_file, indent=4)
输出:
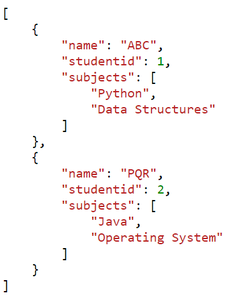
文件名.json
读取 JSON 数据并漂亮地打印出来
要从文件或 URL 读取 JSON,请使用 json.load()。然后使用 json.dumps() 将对象(从读取文件中获得)转换为漂亮的打印 JSON字符串。
例子:
蟒蛇3
# Import required libraries
import json
# Read JSON data from file and pretty print it
with open("filename.json", "r") as read_file:
# Convert JSON file to Python Types
obj = json.load(read_file)
# Pretty print JSON data
pretty_json = json.dumps(obj, indent=4)
print(pretty_json)
输出:
[
{
"studentid": 1,
"name": "ABC",
"subjects": [
"Python",
"Data Structures"
]
},
{
"studentid": 2,
"name": "PQR",
"subjects": [
"Java",
"Operating System"
]
}
]