1. 堆:
堆是一种基于树的数据结构,其中树应该几乎是完整的。它有两种类型,即最大堆和最小堆。
- 最大堆在最大堆中,如果 p 是父级,c 是其子级,那么对于每个父级 p,它的值大于或等于 c 的值
- 最小堆在最小堆中,如果 p 是父级,c 是其子级,则对于每个父级,p 值都小于或等于 c 的值。
堆也作为优先队列。其中最高(在最大堆的情况下)或最低(在最小堆的情况下)元素存在于根处。
堆用于我们需要删除最高或最低优先级元素的问题。堆的一个常见实现是二叉堆。
执行
- 由于堆可以实现为树,但是在这些大量存储空间中浪费了存储指针。由于堆的特性,因为它几乎是完整的二叉树,所以可以很容易地存储在数组中。
- 其中根元素存储在第一个索引处,其子索引可以计算为
- 左子索引 = 2×r 其中 r 是索引是根,数组起始索引是 0。
- 右子索引 = 2×r+1。
- 并且父索引可以计算为 floor(i/2) ,其中 i 是其左孩子或右孩子的索引。
例子 :
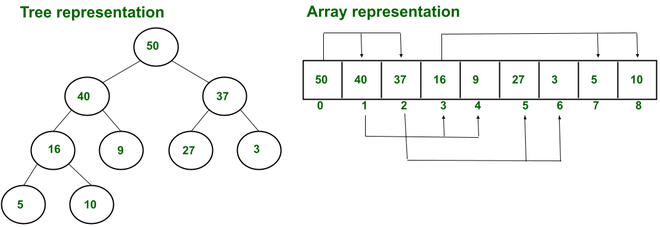
最大堆示例
2. 排序数组:
排序数组是一种数据结构,其中元素按数字、字母或其他顺序排序并存储在连续的内存位置。
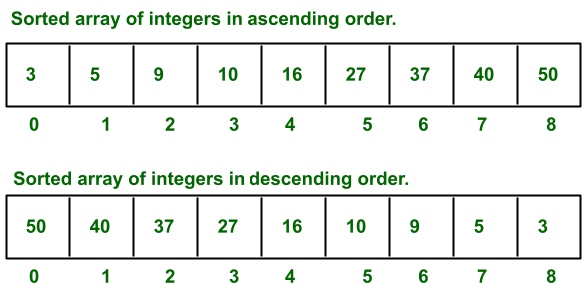
排序数组示例
- 所有数据结构都有自己的优缺点,具体取决于它们使用的问题或算法。例如,在我们需要寻找(最大或最小)、删除(最大或最小)或插入(最大或最小)元素的最优性的情况下,堆是最好的数据结构。
- 排序数组用于需要按升序或降序存储项目的情况。例如,在最短作业调度优先算法中,需要根据进程的突发时间对进程(存储在数组中)进行排序。因此,如果需要排序数组。
Data structure | Insert | Search | Find min | Delete min |
---|---|---|---|---|
Sorted array | O(n) | O(log n) | O(1) | O(n) |
Min heap | O(log n) | O(n) | O(1) | O(log n) |
堆和排序数组的区别:
Sorted array |
Heaps |
---|---|
In sorted array, elements are sorted in numerical, alphabetical, or by some other order and stored at contiguous memory locations. | A heap is almost complete binary tree, In case of max heap if p is the parent and c is its child, then the value of p is greater than or equal to the value of c and in min heap if p is the parent and c is its child, then the value p is less than or equal to the value of c. |
A sorted array can be act as heap when using array based heap implementation. | Heap may or not be a sorted array when using array based heap implementation. |
For a given set of integers there can be two arrangements(i.e. ascending or descending) possible after sorting . |
For a given set of n integers there can be multiple possible heaps(max or min) can be formed. Refer this article for more details |
Here the next element address can be accessed by incrementing the index of current element. | Here the left child index can be accessed by calculating 2×r and right element index can be accessed by calculating 2×r+1, where r is the index of root and array is 0 index based. |
Searching can be performed (log n) time complexity in sorted array by using binary search. | Heap is not optimal for searching operation but searching can be performed in O(n) complexity. |
Heap sort can be used for sorting an array, but for this first heap is build with array of n integers and then heap sort is applied Here O(n) time complexity is needed for building heap and O(n log n) is required for removing n (min or max) element from heap and placing at the end of array and decreasing the size of array) i.e. heap sort. | Heap sort which is applied on heaps(min or max) and it is in-place sorting algorithm for performing sorting in O(n log n) time complexity. |
Sorting an array requires O(n log n) complexity which is best time complexity for sorting an array of n items in comparison based sorting algorithm. | Building heap takes O(n) time complexity. |
Sorted array are used for performing efficient searching (i.e. binary search), SJFS scheduling algorithm and in the organizations where data is needed in sorted order etc. | The heaps are used in heap sort, priority queue, in graph algorithms and K way merge etc. |