十六进制转十进制Java程序
给定一个十六进制数 N,将 N 转换成一个等价的十进制数,即将基数为 16 的数转换为基数 10。十进制数系统使用 10 位数字 0-9,十六进制数系统使用 0-9,AF 来表示任何数值。
插图:
Input : 1AB
Output: 427
Input : 1A
Output: 26
方法:
- 这个想法是提取a的数字 从最右边的数字开始给定的十六进制数。
- 保留一个变量“dec_value”。
- 在从十六进制数中提取数字时,将数字与适当的基数(16 的幂)相乘,并将其添加到上述变量中,即 'dec_value'。
- 最后,变量“dec_value”将存储所需的十进制数。
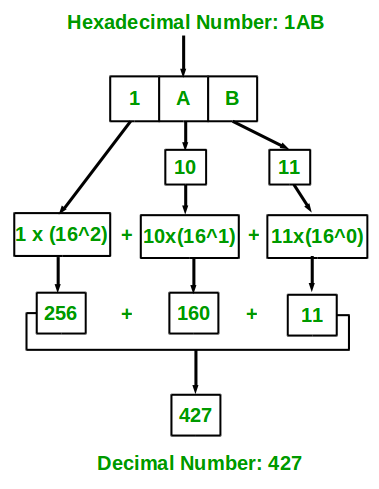
将十六进制数 ( 1AB ) 转换为等效的十进制值
执行:
例子
Java
// Java program to convert Hexadecimal to Decimal Number
// Importing input output classes
import java.io.*;
// Main class
class GFG {
// Method
// To convert hexadecimal to decimal
static int hexadecimalToDecimal(String hexVal)
{
// Storing the length of the
int len = hexVal.length();
// Initializing base value to 1, i.e 16^0
int base = 1;
// Initially declaring and initializing
// decimal value to zero
int dec_val = 0;
// Extracting characters as
// digits from last character
for (int i = len - 1; i >= 0; i--) {
// Condition check
// Case 1
// If character lies in '0'-'9', converting
// it to integral 0-9 by subtracting 48 from
// ASCII value
if (hexVal.charAt(i) >= '0'
&& hexVal.charAt(i) <= '9') {
dec_val += (hexVal.charAt(i) - 48) * base;
// Incrementing base by power
base = base * 16;
}
// Case 2
// if case 1 is bypassed
// Now, if character lies in 'A'-'F' ,
// converting it to integral 10 - 15 by
// subtracting 55 from ASCII value
else if (hexVal.charAt(i) >= 'A'
&& hexVal.charAt(i) <= 'F') {
dec_val += (hexVal.charAt(i) - 55) * base;
// Incrementing base by power
base = base * 16;
}
}
// Returning the decimal value
return dec_val;
}
// Method 2
// Main driver method
public static void main(String[] args)
{
// Custom input hexadecimal number to be
// converted into decimal number
String hexNum = "1A";
// Calling the above method to convert and
// alongside printing the hexadecimal number
System.out.println(hexadecimalToDecimal(hexNum));
}
}
输出
26