Spring Boot – 入门网站
今天,大多数应用程序都需要模型-视图-控制器 (MVC) 架构来满足各种需求,例如处理用户数据、提高应用程序效率、为应用程序提供动态特性。它主要用于构建桌面图形用户界面 (GUI),但现在越来越流行于构建基于 Web 的应用程序。 MVC 不是一个技术栈,而是一种架构模式,它提供了三个重要的逻辑组件模型、视图和控制器。
- 模型:模型是处理数据的数据和逻辑。它表示在控制器或任何其他逻辑之间传输的数据。控制器可以从数据库和/或用户检索数据(模型)。
- 视图:视图是呈现模型数据的应用程序的一部分。数据是数据库形式或来自用户输入。它是从操纵数据(如表格、图表、图表等)向用户输出的演示文稿。
- 控制器:控制器负责处理用户与应用程序的交互。它从用户那里获取鼠标和键盘输入,并根据输入更改模型和视图。
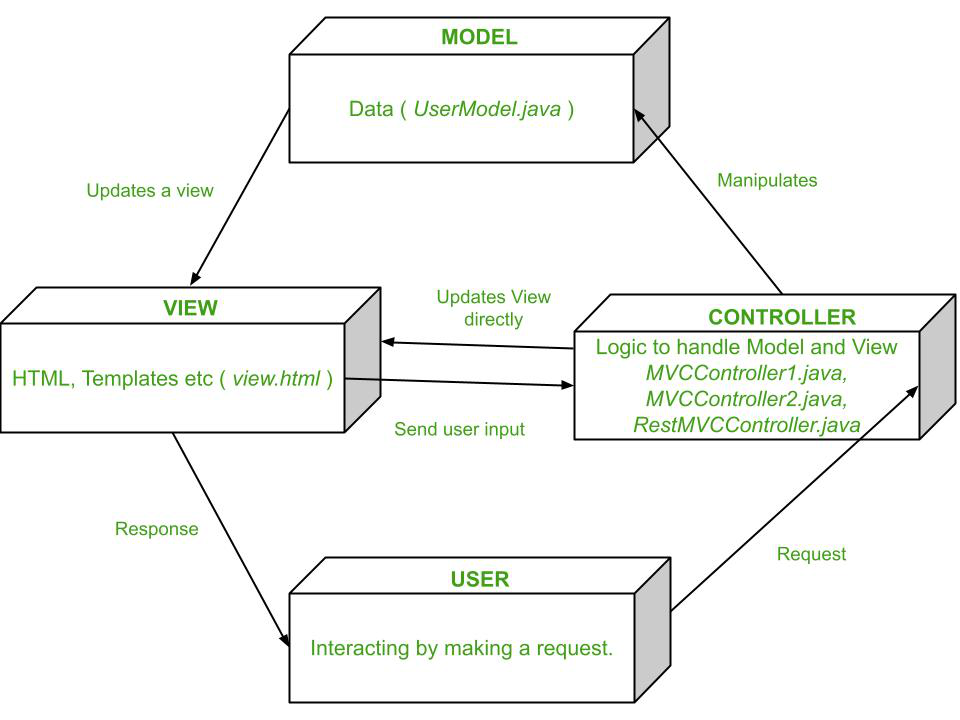
MVC 架构
MVC 架构的优点
- 简单的代码维护简化了应用程序的扩展。
- 测试可以与用户分开进行。
- 模型-视图-控制器降低了复杂性。
- 它对搜索引擎优化 (SEO) 友好。
- 控制器本身允许将相关动作逻辑分组在一起。
Spring 基本特性的核心是 Spring MVC——Spring 的 Web 框架。它被打包在“Spring Web”启动器中。 Spring MVC 也可用于创建产生非 HTML 输出(例如 JSON、XML 等)的 REST API。
在 Spring 应用程序中嵌入 Started Web:
Spring Tool Suite (STS) -> Go To File -> New -> Spring Starter Project -> Next -> Spring Web -> Next -> Finish
您还可以添加 Starter Web 依赖项。
Maven -> pom.xml
org.springframework.boot
spring-boot-starter-web
Gradle -> build.gradle
dependencies {
compile("org.springframework.boot:spring-boot-starter-web")
}
Spring MVC 工作原理
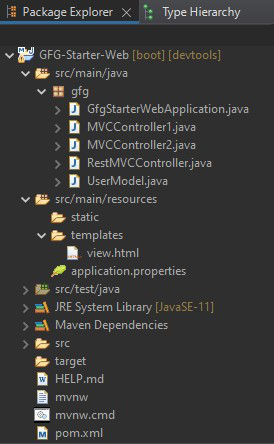
项目结构——Maven
pom.xml (项目配置)
XML
4.0.0
org.springframework.boot
spring-boot-starter-parent
2.6.3
sia
GFG-Starter-Web
0.0.1-SNAPSHOT
GFG-Starter-Web
Spring Boot Starter Web
11
org.springframework.boot
spring-boot-starter-thymeleaf
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-devtools
runtime
true
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-starter
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-maven-plugin
org.projectlombok
lombok
Java
package gfg;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GfgStarterWebApplication {
public static void main(String[] args) {
SpringApplication.run(GfgStarterWebApplication.class, args);
}
}
Java
package gfg;
import lombok.Data;
@Data
public class UserModel {
public String userText;
}
HTML
GeeksforGeeks
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
Java
package gfg;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("userModel")
@RequestMapping("MVC-1")
public class MVCController1 {
@GetMapping
public String get(Model model) {
model.addAttribute("Controller1","You are in Controller-1");
model.addAttribute("userModel", new UserModel());
return "view";
}
@PostMapping
public String post(@ModelAttribute("userModel") UserModel userModel, Model model,RedirectAttributes redirectAttributes) {
redirectAttributes.addFlashAttribute("user", userModel);
return "redirect:/MVC-2";
}
}
Java
package gfg;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("userModel")
@RequestMapping("/MVC-2")
public class MVCController2 {
@GetMapping
public String get(@ModelAttribute("user") UserModel message, Model model) {
model.addAttribute("Controller2","You are in Controller-2");
model.addAttribute("message", message.getUserText());
model.addAttribute("userModel", new UserModel());
return "view";
}
@PostMapping
public String post(@ModelAttribute("userModel") UserModel userModel, Model model,RedirectAttributes redirectAttributes) {
redirectAttributes.addFlashAttribute("message", userModel);
return "redirect:/Rest";
}
}
Java
package gfg;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.support.SessionStatus;
import org.springframework.web.bind.annotation.SessionAttributes;
@RestController
@SessionAttributes("userModel")
@RequestMapping(path="/Rest", produces="application/json")
@CrossOrigin(origins="*")
public class RestMVCController {
@GetMapping
public UserModel get(@ModelAttribute("message") UserModel user, SessionStatus sessionStatus) {
// cleans up the stored
// session attributes (data)
sessionStatus.setComplete();
return user;
}
}
GfgStarterWeb 应用程序。 Java (应用程序的引导)
Java
package gfg;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GfgStarterWebApplication {
public static void main(String[] args) {
SpringApplication.run(GfgStarterWebApplication.class, args);
}
}
用户模型。 Java (模型)
- Java bean 需要 getter 和 setter 方法来更新和访问变量。
- 'Lombok' Java库使用'@Data' 注释自动生成 Getter 和 Setter 方法的过程。
- 要在项目中包含 Lombok,请添加以下依赖项:
Maven -> pom.xml
org.projectlombok
lombok
true
Java
package gfg;
import lombok.Data;
@Data
public class UserModel {
public String userText;
}
view.html (视图 - Thymeleaf 模板)
读取绑定到 UserModel 的用户输入,从而创建模型数据。提交后,将Model数据传递给Controller。
HTML
GeeksforGeeks
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
MVCController1。 Java (控制器)
用于控制器的一些有用的注释是:
- @Controller – 它是 @Component 注释的特殊版本,表示一个类是一个“控制器”,在类路径扫描时会自动检测到它。它与@RequestMapping 等注解、@GetMapping、@PostMapping 等处理方法注解同时工作。
- @RequestMapping - 用于将 Web 请求映射到请求处理类的相应方法上。它既可以用于类级别,也可以用于方法级别。在方法级别的 HTTP 上,应该使用特定的注释。
- @GetMapping – 它将 HTTP GET Web 请求映射到特定的处理程序方法。它的替代方法是'@RequestMapping(method=RequestMethod.GET)'。
- @PostMapping – 它将 HTTP POST Web 请求映射到特定的处理程序方法。它的替代方法是'@RequestMapping(method=RequestMethod.POST)'。
- @SessionAttributes - 它列出了应该存储在会话中并由特定注释处理程序方法使用的模型属性(数据)。
- @ModelAtrribute - 它将方法参数或方法返回值绑定到暴露给 Web 视图的命名模型属性。
这个控制器有两种方法:
- get() – 此方法在 GET 请求上调用,该请求绑定模型数据并返回视图。
- post() - 此方法从用户的 POST 请求中获取模型数据,这些数据将被其他控制器使用并重定向到 MVCController2。Java
Java
package gfg;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("userModel")
@RequestMapping("MVC-1")
public class MVCController1 {
@GetMapping
public String get(Model model) {
model.addAttribute("Controller1","You are in Controller-1");
model.addAttribute("userModel", new UserModel());
return "view";
}
@PostMapping
public String post(@ModelAttribute("userModel") UserModel userModel, Model model,RedirectAttributes redirectAttributes) {
redirectAttributes.addFlashAttribute("user", userModel);
return "redirect:/MVC-2";
}
}
输出:view.html
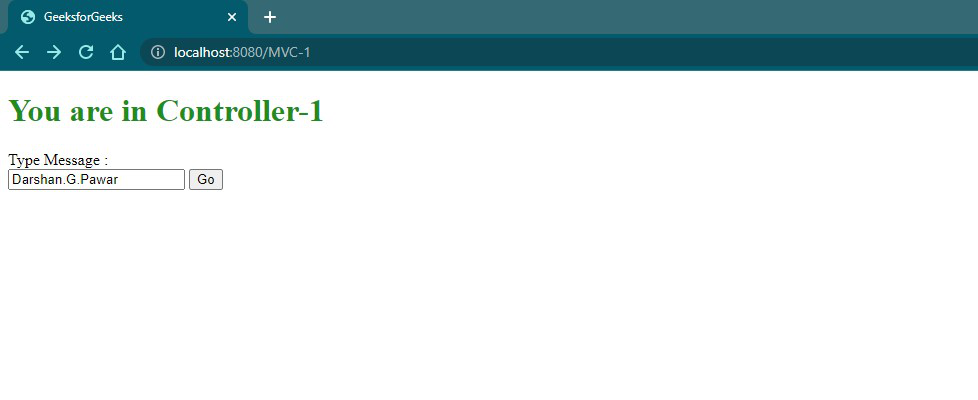
在 MVCController1 上的“GET”请求后返回的视图。Java
MVCController2。 Java (第二个控制器)
这个控制器有两种方法:
- get() - 此方法使用由 MVCController 的 post() 方法转发的模型数据,并与其他模型数据一起返回一个视图。
- post() - 此方法获取模型用户数据,转发它,并重定向到 RestMVCController。
Java
package gfg;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("userModel")
@RequestMapping("/MVC-2")
public class MVCController2 {
@GetMapping
public String get(@ModelAttribute("user") UserModel message, Model model) {
model.addAttribute("Controller2","You are in Controller-2");
model.addAttribute("message", message.getUserText());
model.addAttribute("userModel", new UserModel());
return "view";
}
@PostMapping
public String post(@ModelAttribute("userModel") UserModel userModel, Model model,RedirectAttributes redirectAttributes) {
redirectAttributes.addFlashAttribute("message", userModel);
return "redirect:/Rest";
}
}
输出:view.html
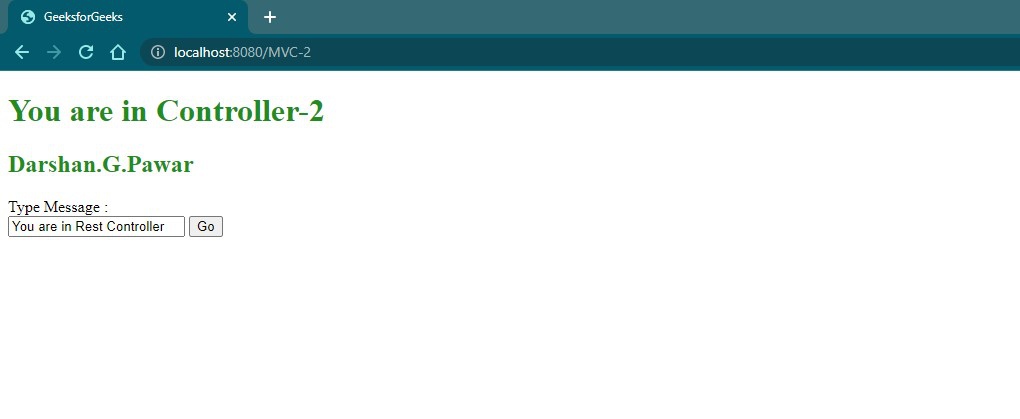
MVCController2 返回的视图。Java
Spring MVC 与 REST API 一起工作
RestMVC控制器。Java(REST API)
使用的一些重要注释是:
- @RestController – 它是 @RequestMapping 和 @ResponseBody 注释的组合,它们在响应正文中而不是作为视图返回数据。
- @CrossOrigin - 它用于允许处理程序类和/或处理程序方法上的跨域请求来使用数据。
Java
package gfg;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.support.SessionStatus;
import org.springframework.web.bind.annotation.SessionAttributes;
@RestController
@SessionAttributes("userModel")
@RequestMapping(path="/Rest", produces="application/json")
@CrossOrigin(origins="*")
public class RestMVCController {
@GetMapping
public UserModel get(@ModelAttribute("message") UserModel user, SessionStatus sessionStatus) {
// cleans up the stored
// session attributes (data)
sessionStatus.setComplete();
return user;
}
}
输出:
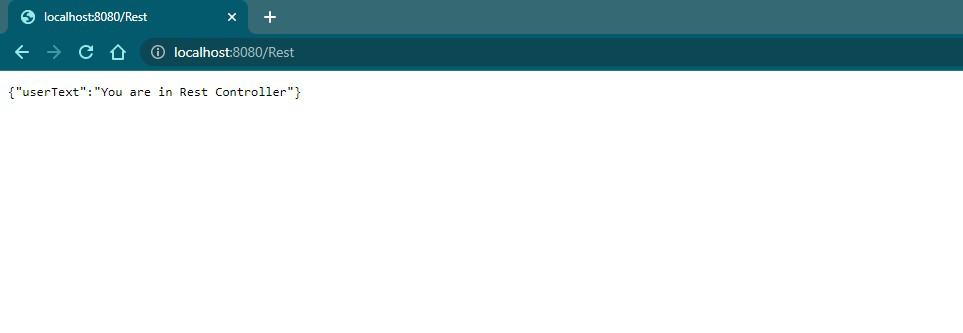
在响应中返回模型数据(JSON字面量)
Note: Spring framework’s back end REST API can work in combination with front end framework technologies like Angular, React, etc which can request for data and also provides the data.