React.js 神奇宝贝应用程序。
简介:在本文中,我们将了解如何使用 React.js 和 Pokémon API 构建 Pokémon 应用程序。
先决条件:
- JavaScript Es6
- 反应钩子
- 反应组件
- 获取 API 数据
- CSS
方法:这个网络应用程序将是一个单页网络应用程序,我们将向不同类型的神奇宝贝展示它们的一些功能,其中还将提到它们的一些功能,我们将使用免费的 API 资源 https:/ 获取所有这些数据/pokeapi.co/。我们将设置一个加载更多按钮,单击该按钮页面将在我们的网页上加载更多神奇宝贝(神奇宝贝的数量将取决于我们在 API 调用中设置的限制值)。
现在让我们看一下上述方法的逐步实现。
创建反应应用程序并安装所有必需的包:
第 1 步:使用以下命令创建一个 React 应用程序:
npx create-react-app foldername
第 2 步:创建项目文件夹(即文件夹名称)后,使用以下命令移动到该文件夹:
cd foldername
项目结构:它将如下所示。
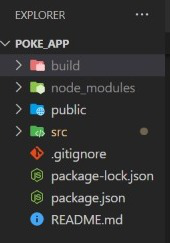
文件管理器
现在忽略 build 文件夹,当你完成制作这个应用程序时,如果你想在某个地方部署你的应用程序,那么你必须在终端中运行“ npm run build ”,这将创建一个build文件夹。然后,您只需将该构建文件夹拖放到您的部署网站 https://www.netlify.com/ 中。
第 3 步:现在在 src 文件夹中,编辑您的 App.js。对于loadPoke ,您可以设置任何您想要的限制,这里我将限制设置为 20。在此文件中,我们使用两个 useState 挂钩容器,一个用于使用https://pokeapi.co/api/获取所有数据及其名称v2/pokemon/${pokemon.name}在 useEffect 中,另一个用于在更多 Pokemons按钮的onClick事件上加载更多 Pokémon,获取API https://pokeapi.co/api/v2/pokemon?limit=20。然后在 Pokémon 应用程序容器中,我们正在构建具有所需特性的整个 Pokémon 卡片并为其他组件设置道具。
App.js
import React, { useEffect, useState } from "react";
import PokemonThumbnail from "./Components/PokemonThumbnail";
function App() {
const [allPokemons, setAllPokemons] = useState([]);
const [loadPoke, setLoadPoke] = useState(
"https://pokeapi.co/api/v2/pokemon?limit=20"
);
const getAllPokemons = async () => {
const res = await fetch(loadPoke);
const data = await res.json();
setLoadPoke(data.next);
function createPokemonObject(result) {
result.forEach(async (pokemon) => {
const res = await fetch(
`https://pokeapi.co/api/v2/pokemon/${pokemon.name}`
);
const data = await res.json();
setAllPokemons((currentList) => [...currentList, data]);
});
}
createPokemonObject(data.results);
await console.log(allPokemons);
};
useEffect(() => {
getAllPokemons();
}, []);
return (
Pokemon Kingdom .
{allPokemons.map((pokemon, index) => (
))}
);
}
export default App;
PokemonThumnail.js
import React, { useState } from "react";
import Description from "./Description";
const PokemonThumbnail = ({
id,
name,
image,
type,
height,
weight,
stat1,
stat2,
stat3,
stat4,
stat5,
stat6,
bs1,
bs2,
bs3,
bs4,
bs5,
bs6,
}) => {
const style = `thumb-container ${type}`;
const [show, setShow] = useState(false);
return (
#0{id}
{name.toUpperCase()}
Type : {type}
{show === true ? (
) : (
<>>
)}
);
};
export default PokemonThumbnail;
Description.js
import React from "react";
const Description = ({
heightpok,
weightpok,
pokstat1,
pokstat2,
pokstat3,
pokstat4,
pokstat5,
pokstat6,
posbs1,
posbs2,
posbs3,
posbs4,
posbs5,
posbs6,
}) => {
return (
Height is {heightpok * 10} cm.
Weight is {weightpok * 0.1} kg
Stat
{pokstat1} : {posbs1}
{pokstat2} : {posbs2}
{pokstat3} : {posbs3}
{pokstat4} : {posbs4}
{pokstat5} : {posbs5}
{pokstat6} : {posbs6}
);
};
export default Description;
src/index.css
@import url("https://fonts.googleapis.com/css2?family=Satisfy&display=swap");
body {
margin: 0;
font-family: "Satisfy", cursive;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
background-image: linear-gradient(
to right,
#eea2a2 0%,
#bbc1bf 19%,
#57c6e1 42%,
#b49fda 79%,
#7ac5d8 100%
);
}
code {
font-family: "Satisfy", cursive;
}
.rock {
background-image: linear-gradient(
to top, #c79081 0%, #dfa579 100%);
}
.ghost {
background-image: linear-gradient(
to top, #cfd9df 0%, #e2ebf0 100%);
}
.electric {
background-image: linear-gradient(
to right, #f83600 0%, #f9d423 100%);
}
.bug {
background-image: linear-gradient(
to top, #e6b980 0%, #eacda3 100%);
}
.poison {
background-image: linear-gradient(
to top, #df89b5 0%, #bfd9fe 100%);
}
.normal {
background-image: linear-gradient(
-225deg, #e3fdf5 0%, #ffe6fa 100%);
}
.fairy {
background-image: linear-gradient(
to top,
#ff9a9e 0%,
#fecfef 99%,
#fecfef 100%
);
}
.fire {
background-image: linear-gradient(
120deg, #f6d365 0%, #fda085 100%);
}
.grass {
background-image: linear-gradient(
120deg, #d4fc79 0%, #96e6a1 100%);
}
.water {
background-image: linear-gradient(
120deg, #89f7fe 0%, #66a6ff 100%);
}
.ground {
background-image: linear-gradient(
315deg, #772f1a 0%, #f2a65a 74%);
}
.app-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 100vh;
padding: 3rem 1rem;
}
.app-container h1 {
width: 0ch;
color: rgb(16, 166, 236);
overflow: hidden;
white-space: nowrap;
animation: text 4s steps(15) infinite alternate;
}
@keyframes text {
0% {
width: 0ch;
}
50% {
width: 15ch;
color: rgb(218, 55, 5);
}
}
.pokemon-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.all-container {
display: flex;
flex-wrap: wrap;
align-items: center;
justify-content: center;
}
.thumb-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
padding: 1.5rem 0.2rem;
margin: 0.3rem;
border: 1px solid #efefef;
border-radius: 2rem;
min-width: 160px;
text-align: center;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.089);
}
.thumb-container :hover {
margin: 0.7rem 0;
}
h3 {
margin-bottom: 0.2rem;
}
.thumb-container .number {
border-radius: 1rem;
padding: 0.2rem 0.4rem;
background-color: rgba(255, 255, 255, 0.3);
}
.thumb-container img {
width: 120px;
height: 120px;
}
.thumb-container small {
text-transform: capitalize;
}
.detail-wrapper {
display: flex;
flex-direction: column;
width: 100%;
}
.detail-wrapper button {
color: rgb(22, 22, 22);
padding: 0.5rem;
margin-top: 1rem;
border: none;
border-radius: 0.2rem;
cursor: pointer;
background-color: rgba(0, 0, 0, 0.185);
}
.load-more {
background-color: #76daff;
background-image: linear-gradient(
315deg, #76daff 0%, #fcd000 74%);
border-radius: 6px;
border: 1px solid #c6c6c6;
color: #444;
padding: 0.5rem 1.5rem;
min-width: 20%;
margin-top: 1rem;
font-family: "Satisfy", cursive;
}
button:hover {
background-color: #ff0000;
background-image: linear-gradient(
315deg, #ff0000 0%, #ffed00 74%);
}
.pokeinfo {
margin: 0 25px;
font-family: "Satisfy", cursive;
background-image: linear-gradient(
180deg, #2af598 0%, #009efd 100%);
}
.pokeinfo:hover {
background-image: linear-gradient(
-20deg,
#ddd6f3 0%,
#faaca8 100%,
#faaca8 100%
);
}
h3 {
text-decoration: underline;
}
第 4 步:现在在src文件夹中,创建一个 Components 文件夹,在此文件夹中创建两个文件,一个用于 Pokémon 缩略图,另一个用于描述。在您的src/Components/PokemonThumnail.js (或您喜欢的任何名称)中粘贴下面给出的代码。在这个文件中,我们使用了 App.js 传递的所有必需的道具,并创建了整个 Pokémon 缩略图结构。在这里,我们使用 useState 来显示在单击加载更多按钮时的 Description.js 组件,默认情况下它已设置为 false 。
PokemonThumnail.js
import React, { useState } from "react";
import Description from "./Description";
const PokemonThumbnail = ({
id,
name,
image,
type,
height,
weight,
stat1,
stat2,
stat3,
stat4,
stat5,
stat6,
bs1,
bs2,
bs3,
bs4,
bs5,
bs6,
}) => {
const style = `thumb-container ${type}`;
const [show, setShow] = useState(false);
return (
#0{id}
{name.toUpperCase()}
Type : {type}
{show === true ? (
) : (
<>>
)}
);
};
export default PokemonThumbnail;
第 5 步:将下面给出的代码粘贴到您的src/Components/Description.js (或您将提供的任何名称)中。在这个文件中,我们正在制作一个用于描述神奇宝贝的组件,这里我们也使用了 App.js 传递的道具。我们的神奇宝贝缩略图中有一个了解更多按钮,单击该特定按钮时,此描述将显示在我们的缩略图 UI 中,单击了解更少缩略图将显示其初始状态。
描述.js
import React from "react";
const Description = ({
heightpok,
weightpok,
pokstat1,
pokstat2,
pokstat3,
pokstat4,
pokstat5,
pokstat6,
posbs1,
posbs2,
posbs3,
posbs4,
posbs5,
posbs6,
}) => {
return (
Height is {heightpok * 10} cm.
Weight is {weightpok * 0.1} kg
Stat
{pokstat1} : {posbs1}
{pokstat2} : {posbs2}
{pokstat3} : {posbs3}
{pokstat4} : {posbs4}
{pokstat5} : {posbs5}
{pokstat6} : {posbs6}
);
};
export default Description;
第 6 步:转到您的src/index.css ,粘贴下面给出的代码,这将为您的 Pokémon Web 应用程序添加所有组件的样式。
src/index.css
@import url("https://fonts.googleapis.com/css2?family=Satisfy&display=swap");
body {
margin: 0;
font-family: "Satisfy", cursive;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
background-image: linear-gradient(
to right,
#eea2a2 0%,
#bbc1bf 19%,
#57c6e1 42%,
#b49fda 79%,
#7ac5d8 100%
);
}
code {
font-family: "Satisfy", cursive;
}
.rock {
background-image: linear-gradient(
to top, #c79081 0%, #dfa579 100%);
}
.ghost {
background-image: linear-gradient(
to top, #cfd9df 0%, #e2ebf0 100%);
}
.electric {
background-image: linear-gradient(
to right, #f83600 0%, #f9d423 100%);
}
.bug {
background-image: linear-gradient(
to top, #e6b980 0%, #eacda3 100%);
}
.poison {
background-image: linear-gradient(
to top, #df89b5 0%, #bfd9fe 100%);
}
.normal {
background-image: linear-gradient(
-225deg, #e3fdf5 0%, #ffe6fa 100%);
}
.fairy {
background-image: linear-gradient(
to top,
#ff9a9e 0%,
#fecfef 99%,
#fecfef 100%
);
}
.fire {
background-image: linear-gradient(
120deg, #f6d365 0%, #fda085 100%);
}
.grass {
background-image: linear-gradient(
120deg, #d4fc79 0%, #96e6a1 100%);
}
.water {
background-image: linear-gradient(
120deg, #89f7fe 0%, #66a6ff 100%);
}
.ground {
background-image: linear-gradient(
315deg, #772f1a 0%, #f2a65a 74%);
}
.app-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 100vh;
padding: 3rem 1rem;
}
.app-container h1 {
width: 0ch;
color: rgb(16, 166, 236);
overflow: hidden;
white-space: nowrap;
animation: text 4s steps(15) infinite alternate;
}
@keyframes text {
0% {
width: 0ch;
}
50% {
width: 15ch;
color: rgb(218, 55, 5);
}
}
.pokemon-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.all-container {
display: flex;
flex-wrap: wrap;
align-items: center;
justify-content: center;
}
.thumb-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
padding: 1.5rem 0.2rem;
margin: 0.3rem;
border: 1px solid #efefef;
border-radius: 2rem;
min-width: 160px;
text-align: center;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.089);
}
.thumb-container :hover {
margin: 0.7rem 0;
}
h3 {
margin-bottom: 0.2rem;
}
.thumb-container .number {
border-radius: 1rem;
padding: 0.2rem 0.4rem;
background-color: rgba(255, 255, 255, 0.3);
}
.thumb-container img {
width: 120px;
height: 120px;
}
.thumb-container small {
text-transform: capitalize;
}
.detail-wrapper {
display: flex;
flex-direction: column;
width: 100%;
}
.detail-wrapper button {
color: rgb(22, 22, 22);
padding: 0.5rem;
margin-top: 1rem;
border: none;
border-radius: 0.2rem;
cursor: pointer;
background-color: rgba(0, 0, 0, 0.185);
}
.load-more {
background-color: #76daff;
background-image: linear-gradient(
315deg, #76daff 0%, #fcd000 74%);
border-radius: 6px;
border: 1px solid #c6c6c6;
color: #444;
padding: 0.5rem 1.5rem;
min-width: 20%;
margin-top: 1rem;
font-family: "Satisfy", cursive;
}
button:hover {
background-color: #ff0000;
background-image: linear-gradient(
315deg, #ff0000 0%, #ffed00 74%);
}
.pokeinfo {
margin: 0 25px;
font-family: "Satisfy", cursive;
background-image: linear-gradient(
180deg, #2af598 0%, #009efd 100%);
}
.pokeinfo:hover {
background-image: linear-gradient(
-20deg,
#ddd6f3 0%,
#faaca8 100%,
#faaca8 100%
);
}
h3 {
text-decoration: underline;
}
运行应用程序的步骤:从项目的根目录使用以下命令运行应用程序:
npm start
输出:现在打开浏览器并转到http://localhost:3000/ ,您将看到以下输出:
在评论中写代码?请使用 ide.geeksforgeeks.org,生成链接并在此处分享链接。