Xstream 的春天
Xstream是一个简单的基于Java的序列化/反序列化库,用于将Java对象转换为其XML表示形式。它还可用于将XML字符串转换为等效的Java对象。它是对Java标准库的快速、高效的扩展。它也是高度可定制的。对于本教程,我们假设Java和环境变量已正确安装在您的本地环境中。
下载 XStream 档案
从此链接下载最新的 Xstream 存档。如果您下载了 jar,那么您必须手动或在 IDE 的帮助下设置 CLASSPATH 变量,(或)
使用 Maven:
如果您通过 maven 中央存储库将其添加到您的项目中,那么您不需要设置 CLASSPATH 变量,maven 会自动为您执行此操作。
com.thoughtworks.xstream
xstream
1.4.19
设置 CLASSPATH 变量
手动:
Linux:
export CLASSPATH=$CLASSPATH:$XStream_HOME/xstream-1.4.19.jar:
视窗:
将环境变量 CLASSPATH 设置为
%CLASSPATH%;%XStream_HOME%\xstream-1.4.19.jar;
苹果电脑:
export CLASSPATH=$CLASSPATH:$XStream_HOME/xstream-1.4.19.jar:
(或者)
在IDE的帮助下:在IntelliJ IDEA中执行以下步骤:
步骤:
右键单击项目->打开模块设置->库->单击“+” ->添加 Xstream Jar ->应用并确定
XStream 应用程序
确保您已经设置了 Spring 核心和 web jar 文件
Java
import lombok.Getter;
@Getter
public class Employee {
private String firstName;
private String lastName;
private int salary;
private int age;
private String gender;
public Employee(String firstName, String lastName,
int salary, int age, String gender)
{
this.firstName = firstName;
this.lastName = lastName;
this.salary = salary;
this.age = age;
this.gender = gender;
}
}
Java
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
import java.io.FileWriter;
import java.io.IOException;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class XStreamExampleApplication {
public static void main(String[] args)
throws IOException
{
// Initializing XStream with Dom driver
XStream xStream = new XStream(new DomDriver());
// Now, to make the XML outputted by XStream more
// concise, you can create aliases for your custom
// class names to XML element names. This is the
// only type of mapping required to use XStream and
// even this is optional.
xStream.alias("employee", Employee.class);
Employee e1 = new Employee("Sanyog", "Gautam", 1000,
19, "Male");
// Serializing a Java object into XML
String xml
= xStream.toXML(e1); // Converting it to XML
System.out.println(xml);
// Java Object to a file
try (FileWriter writer = new FileWriter(
"/home/anurag/xstreamExample.xml")) {
xStream.toXML(e1, writer);
}
catch (IOException e) {
e.printStackTrace();
}
}
}
Java
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
import java.io.IOException;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class XStreamExampleApplication {
public static void main(String[] args)
throws IOException
{
// Initializing XStream with Dom driver
XStream xStream = new XStream(new DomDriver());
// We need to configure the security framework in
// XStream, so it deserializes the object from the
// XML
xStream.allowTypes(new Class[] { Employee.class });
// Now, to make the XML outputted by XStream more
// concise, you can create aliases for your custom
// class names to XML element names. This is the
// only type of mapping required to use XStream and
// even this is optional.
xStream.alias("employee", Employee.class);
Employee e1 = new Employee("Sanyog", "Gautam", 1000,
19, "Male");
// Serializing a Java object into XML
String xml
= xStream.toXML(e1); // Converting it to XML
// Deserializing a Java object from XML
Employee employee = (Employee)xStream.fromXML(xml);
System.out.println("First name of Employee: "
+ employee.getFirstName());
System.out.println("Last name of Employee: "
+ employee.getLastName());
System.out.println("Employee's age: "
+ employee.getAge());
System.out.println("Employee's gender: "
+ employee.getGender());
System.out.println("Employee's salary: $"
+ employee.getSalary());
}
}
如您所见,我们得到了干净的 XML
Sanyog
Gautam
100
19
Male
我们可以从那个 XML 反序列化我们的对象
Java
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
import java.io.IOException;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class XStreamExampleApplication {
public static void main(String[] args)
throws IOException
{
// Initializing XStream with Dom driver
XStream xStream = new XStream(new DomDriver());
// We need to configure the security framework in
// XStream, so it deserializes the object from the
// XML
xStream.allowTypes(new Class[] { Employee.class });
// Now, to make the XML outputted by XStream more
// concise, you can create aliases for your custom
// class names to XML element names. This is the
// only type of mapping required to use XStream and
// even this is optional.
xStream.alias("employee", Employee.class);
Employee e1 = new Employee("Sanyog", "Gautam", 1000,
19, "Male");
// Serializing a Java object into XML
String xml
= xStream.toXML(e1); // Converting it to XML
// Deserializing a Java object from XML
Employee employee = (Employee)xStream.fromXML(xml);
System.out.println("First name of Employee: "
+ employee.getFirstName());
System.out.println("Last name of Employee: "
+ employee.getLastName());
System.out.println("Employee's age: "
+ employee.getAge());
System.out.println("Employee's gender: "
+ employee.getGender());
System.out.println("Employee's salary: $"
+ employee.getSalary());
}
}
我们取回我们的对象
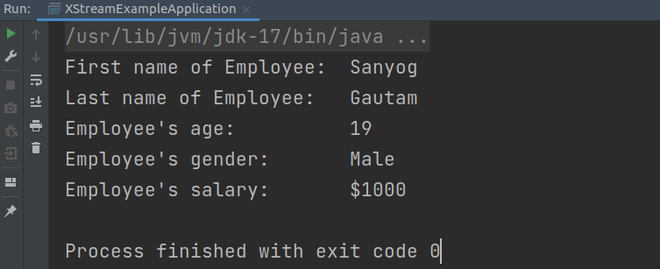
来自 XML 的反序列化对象