在Python中使用 Turtle 创建一个贪吃蛇游戏
蛇类游戏是由 Gremlin Industries 开发、世嘉于 1976 年 10 月发行的街机迷宫游戏,被认为是一种技巧性游戏,并在几代人中普及。 Snake 游戏中的蛇是使用相对于它前进的方向的四个方向按钮来控制的。玩家在游戏中的目标是通过收集食物或水果来获得尽可能高的分数。一旦蛇撞到墙壁或撞到自己,玩家就输了。
对于Python初学者,那些有兴趣在您的领域中使事情变得更简单的人绝对可以尝试一下,而Turtle模块正是为此目的而制作的,供初学者尝试,也可以作为项目的一部分提交。该程序将在Python 3中完成。
因此,我们将使用以下模块创建一个基于 Python 的游戏:
- Turtle:它是一个预装的Python库,使用户能够通过为他们提供虚拟画布来创建形状和图片。
- 时间:此函数用于计算自纪元以来经过的秒数。
- Random:该函数用于在Python中使用random模块生成随机数。
支持
使用专为Python程序设计的PyCharm应用程序可以轻松完成以下代码。
此外,VSCode可用于此程序。从 VSCode 的扩展安装 Python3。然后,以your_filename.py的形式保存程序
以下是使用 Turtle 模块创建贪吃蛇游戏的分步方法:
第 1 步:我们将模块导入程序并为游戏提供默认值。
Python3
import turtle
import time
import random
delay = 0.1
score = 0
high_score = 0
Python3
# Creating a window screen
wn = turtle.Screen()
wn.title("Snake Game")
wn.bgcolor("blue")
# the width and height can be put as user's choice
wn.setup(width=600, height=600)
wn.tracer(0)
# head of the snake
head = turtle.Turtle()
head.shape("square")
head.color("white")
head.penup()
head.goto(0, 0)
head.direction = "Stop"
# food in the game
food = turtle.Turtle()
colors = random.choice(['red', 'green', 'black'])
shapes = random.choice(['square', 'triangle', 'circle'])
food.speed(0)
food.shape(shapes)
food.color(colors)
food.penup()
food.goto(0, 100)
pen = turtle.Turtle()
pen.speed(0)
pen.shape("square")
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Score : 0 High Score : 0", align="center",
font=("candara", 24, "bold"))
Python3
# assigning key directions
def goup():
if head.direction != "down":
head.direction = "up"
def godown():
if head.direction != "up":
head.direction = "down"
def goleft():
if head.direction != "right":
head.direction = "left"
def goright():
if head.direction != "left":
head.direction = "right"
def move():
if head.direction == "up":
y = head.ycor()
head.sety(y+20)
if head.direction == "down":
y = head.ycor()
head.sety(y-20)
if head.direction == "left":
x = head.xcor()
head.setx(x-20)
if head.direction == "right":
x = head.xcor()
head.setx(x+20)
wn.listen()
wn.onkeypress(goup, "w")
wn.onkeypress(godown, "s")
wn.onkeypress(goleft, "a")
wn.onkeypress(goright, "d")
Python3
segments = []
# Main Gameplay
while True:
wn.update()
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
time.sleep(1)
head.goto(0, 0)
head.direction = "Stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
if head.distance(food) < 20:
x = random.randint(-270, 270)
y = random.randint(-270, 270)
food.goto(x, y)
# Adding segment
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("orange") # tail colour
new_segment.penup()
segments.append(new_segment)
delay -= 0.001
score += 10
if score > high_score:
high_score = score
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
# Checking for head collisions with body segments
for index in range(len(segments)-1, 0, -1):
x = segments[index-1].xcor()
y = segments[index-1].ycor()
segments[index].goto(x, y)
if len(segments) > 0:
x = head.xcor()
y = head.ycor()
segments[0].goto(x, y)
move()
for segment in segments:
if segment.distance(head) < 20:
time.sleep(1)
head.goto(0, 0)
head.direction = "stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segment.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
time.sleep(delay)
wn.mainloop()
Python3
# import required modules
import turtle
import time
import random
delay = 0.1
score = 0
high_score = 0
# Creating a window screen
wn = turtle.Screen()
wn.title("Snake Game")
wn.bgcolor("blue")
# the width and height can be put as user's choice
wn.setup(width=600, height=600)
wn.tracer(0)
# head of the snake
head = turtle.Turtle()
head.shape("square")
head.color("white")
head.penup()
head.goto(0, 0)
head.direction = "Stop"
# food in the game
food = turtle.Turtle()
colors = random.choice(['red', 'green', 'black'])
shapes = random.choice(['square', 'triangle', 'circle'])
food.speed(0)
food.shape(shapes)
food.color(colors)
food.penup()
food.goto(0, 100)
pen = turtle.Turtle()
pen.speed(0)
pen.shape("square")
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Score : 0 High Score : 0", align="center",
font=("candara", 24, "bold"))
# assigning key directions
def goup():
if head.direction != "down":
head.direction = "up"
def godown():
if head.direction != "up":
head.direction = "down"
def goleft():
if head.direction != "right":
head.direction = "left"
def goright():
if head.direction != "left":
head.direction = "right"
def move():
if head.direction == "up":
y = head.ycor()
head.sety(y+20)
if head.direction == "down":
y = head.ycor()
head.sety(y-20)
if head.direction == "left":
x = head.xcor()
head.setx(x-20)
if head.direction == "right":
x = head.xcor()
head.setx(x+20)
wn.listen()
wn.onkeypress(goup, "w")
wn.onkeypress(godown, "s")
wn.onkeypress(goleft, "a")
wn.onkeypress(goright, "d")
segments = []
# Main Gameplay
while True:
wn.update()
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
time.sleep(1)
head.goto(0, 0)
head.direction = "Stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
if head.distance(food) < 20:
x = random.randint(-270, 270)
y = random.randint(-270, 270)
food.goto(x, y)
# Adding segment
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("orange") # tail colour
new_segment.penup()
segments.append(new_segment)
delay -= 0.001
score += 10
if score > high_score:
high_score = score
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
# Checking for head collisions with body segments
for index in range(len(segments)-1, 0, -1):
x = segments[index-1].xcor()
y = segments[index-1].ycor()
segments[index].goto(x, y)
if len(segments) > 0:
x = head.xcor()
y = head.ycor()
segments[0].goto(x, y)
move()
for segment in segments:
if segment.distance(head) < 20:
time.sleep(1)
head.goto(0, 0)
head.direction = "stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segment.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
time.sleep(delay)
wn.mainloop()
第 2 步:现在,我们将创建游戏的显示,即游戏的窗口屏幕,我们将在游戏中创建蛇的头部和食物,并在游戏的标题处显示分数.
蟒蛇3
# Creating a window screen
wn = turtle.Screen()
wn.title("Snake Game")
wn.bgcolor("blue")
# the width and height can be put as user's choice
wn.setup(width=600, height=600)
wn.tracer(0)
# head of the snake
head = turtle.Turtle()
head.shape("square")
head.color("white")
head.penup()
head.goto(0, 0)
head.direction = "Stop"
# food in the game
food = turtle.Turtle()
colors = random.choice(['red', 'green', 'black'])
shapes = random.choice(['square', 'triangle', 'circle'])
food.speed(0)
food.shape(shapes)
food.color(colors)
food.penup()
food.goto(0, 100)
pen = turtle.Turtle()
pen.speed(0)
pen.shape("square")
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Score : 0 High Score : 0", align="center",
font=("candara", 24, "bold"))
输出:

初始分数为header,白色:蛇头,红色:水果
第 3 步:现在,我们将验证蛇运动的关键。通过点击通常用于游戏的关键字“w”、“a”、“s”和“d”,我们可以操作蛇在屏幕上的动作。
蟒蛇3
# assigning key directions
def goup():
if head.direction != "down":
head.direction = "up"
def godown():
if head.direction != "up":
head.direction = "down"
def goleft():
if head.direction != "right":
head.direction = "left"
def goright():
if head.direction != "left":
head.direction = "right"
def move():
if head.direction == "up":
y = head.ycor()
head.sety(y+20)
if head.direction == "down":
y = head.ycor()
head.sety(y-20)
if head.direction == "left":
x = head.xcor()
head.setx(x-20)
if head.direction == "right":
x = head.xcor()
head.setx(x+20)
wn.listen()
wn.onkeypress(goup, "w")
wn.onkeypress(godown, "s")
wn.onkeypress(goleft, "a")
wn.onkeypress(goright, "d")
第 4 步:现在,最后,我们将创建将发生以下情况的游戏玩法:
- 当蛇吃水果时,蛇会长出它的身体。
- 给蛇的尾巴上色。
- 水果吃完后,会计算分数。
- 检查蛇的头部是否与身体或窗纱的墙壁发生碰撞。
- 碰撞后自动重新开始游戏。
- 每次重新启动窗口时都会引入水果的新形状和颜色。
- 分数将归零并保留一个高分,直到窗口未关闭。
蟒蛇3
segments = []
# Main Gameplay
while True:
wn.update()
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
time.sleep(1)
head.goto(0, 0)
head.direction = "Stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
if head.distance(food) < 20:
x = random.randint(-270, 270)
y = random.randint(-270, 270)
food.goto(x, y)
# Adding segment
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("orange") # tail colour
new_segment.penup()
segments.append(new_segment)
delay -= 0.001
score += 10
if score > high_score:
high_score = score
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
# Checking for head collisions with body segments
for index in range(len(segments)-1, 0, -1):
x = segments[index-1].xcor()
y = segments[index-1].ycor()
segments[index].goto(x, y)
if len(segments) > 0:
x = head.xcor()
y = head.ycor()
segments[0].goto(x, y)
move()
for segment in segments:
if segment.distance(head) < 20:
time.sleep(1)
head.goto(0, 0)
head.direction = "stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segment.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
time.sleep(delay)
wn.mainloop()
下面是基于上述方法的完整程序:
蟒蛇3
# import required modules
import turtle
import time
import random
delay = 0.1
score = 0
high_score = 0
# Creating a window screen
wn = turtle.Screen()
wn.title("Snake Game")
wn.bgcolor("blue")
# the width and height can be put as user's choice
wn.setup(width=600, height=600)
wn.tracer(0)
# head of the snake
head = turtle.Turtle()
head.shape("square")
head.color("white")
head.penup()
head.goto(0, 0)
head.direction = "Stop"
# food in the game
food = turtle.Turtle()
colors = random.choice(['red', 'green', 'black'])
shapes = random.choice(['square', 'triangle', 'circle'])
food.speed(0)
food.shape(shapes)
food.color(colors)
food.penup()
food.goto(0, 100)
pen = turtle.Turtle()
pen.speed(0)
pen.shape("square")
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Score : 0 High Score : 0", align="center",
font=("candara", 24, "bold"))
# assigning key directions
def goup():
if head.direction != "down":
head.direction = "up"
def godown():
if head.direction != "up":
head.direction = "down"
def goleft():
if head.direction != "right":
head.direction = "left"
def goright():
if head.direction != "left":
head.direction = "right"
def move():
if head.direction == "up":
y = head.ycor()
head.sety(y+20)
if head.direction == "down":
y = head.ycor()
head.sety(y-20)
if head.direction == "left":
x = head.xcor()
head.setx(x-20)
if head.direction == "right":
x = head.xcor()
head.setx(x+20)
wn.listen()
wn.onkeypress(goup, "w")
wn.onkeypress(godown, "s")
wn.onkeypress(goleft, "a")
wn.onkeypress(goright, "d")
segments = []
# Main Gameplay
while True:
wn.update()
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
time.sleep(1)
head.goto(0, 0)
head.direction = "Stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
if head.distance(food) < 20:
x = random.randint(-270, 270)
y = random.randint(-270, 270)
food.goto(x, y)
# Adding segment
new_segment = turtle.Turtle()
new_segment.speed(0)
new_segment.shape("square")
new_segment.color("orange") # tail colour
new_segment.penup()
segments.append(new_segment)
delay -= 0.001
score += 10
if score > high_score:
high_score = score
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
# Checking for head collisions with body segments
for index in range(len(segments)-1, 0, -1):
x = segments[index-1].xcor()
y = segments[index-1].ycor()
segments[index].goto(x, y)
if len(segments) > 0:
x = head.xcor()
y = head.ycor()
segments[0].goto(x, y)
move()
for segment in segments:
if segment.distance(head) < 20:
time.sleep(1)
head.goto(0, 0)
head.direction = "stop"
colors = random.choice(['red', 'blue', 'green'])
shapes = random.choice(['square', 'circle'])
for segment in segments:
segment.goto(1000, 1000)
segment.clear()
score = 0
delay = 0.1
pen.clear()
pen.write("Score : {} High Score : {} ".format(
score, high_score), align="center", font=("candara", 24, "bold"))
time.sleep(delay)
wn.mainloop()
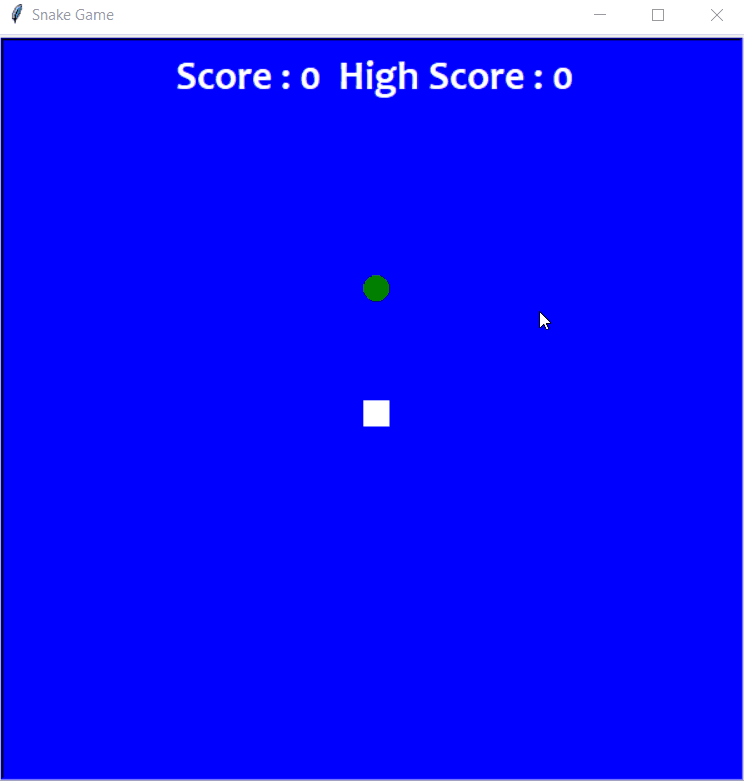
演示最终游戏,显示所有用代码编写的内容