JSDoc 中的文档注释
在 JSDoc 中,我们需要在代码中包含文档注释,JSDoc 将通过这些注释生成 HTML 文档网站。让我们看看如何为不同类型的代码创建文档注释。
字符串、数字和数组:
Javascript
/**
* Site Name
* @type {string}
*/
const siteName = "GeeksForGeeks";
/**
* Number
* @type {number}
*/
const number = 1000;
/**
* Array
* @type {Array}
*/
const myArray = [10, 20, 30];
Javascript
/**
* Site object
* @type {{id: number, name: string, rank: number|string}}
*/
const site = {
id: 1,
name: "gfg",
rank: 1,
};
Javascript
/**
* Calculate age
* @param {number} current Current year
* @param {number} yearOfBirth Year of Birth
* @returns {string} your calculated age
*/
const calcAge = (current, yearOfBirth) => {
return `${current - yearOfBirth}`;
};
Javascript
/**
* Class to create new owner
*/
class Owner {
/**
* Owner details
* @param {Object} ownerDetail
*/
constructor(ownerDetail) {
/**
* @property {string} name Owner name
*/
this.name = ownerDetail.name;
/**
* @property {number} age Owner's age
*/
this.age = ownerDetail.age;
}
/**
* @property {Function} printOwner Prints Owner's details
* @returns {void}
*/
printOwner() {
console.log(`Owner's name is ${this.name}
and his age is ${this.age}`);
}
}
Javascript
/**
* Link to Owner Class
* See {@link Owner}
*/
const gfg = new Owner({
name: "GeeksForGeeks",
age: 13,
});
Javascript
// @ts-check
/**
* Site Name
* @type {string}
*/
const siteName = "GeeksForGeeks";
/**
* Number
* @type {number}
*/
const number = 1000;
/**
* Array
* @type {Array}
*/
const myArray = [10, 20, 30];
/**
* Site object
* @type {{id: number, name: string, rank: number|string}}
*/
const site = {
id: 1,
name: "gfg",
rank: 1,
};
/**
* Calculate age
* @param {number} current Current year
* @param {number} yearOfBirth Year of Birth
* @returns {string} your calculated age
*/
const calcAge = (current, yearOfBirth) => {
return `${current - yearOfBirth}`;
};
/**
* Class to create new owner
*/
class Owner {
/**
* Owner details
* @param {Object} ownerDetail
*/
constructor(ownerDetail) {
/**
* @property {string} name Owner name
*/
this.name = ownerDetail.name;
/**
* @property {number} age Owner's age
*/
this.age = ownerDetail.age;
}
/**
* @property {Function} printOwner Prints Owner's details
* @returns {void}
*/
printOwner() {
console.log(`Owner's name is ${this.name} and his age is ${this.age}`);
}
}
/**
* Link to Owner Class
* See {@link Owner}
*/
const gfg = new Owner({
name: "GeeksForGeeks",
age: 13,
});
在这里,我们生成了简单的 JSDoc 注释,其中包含变量的描述及其数据类型,这些数据类型是在@type标记的帮助下表示的。
对象:
Javascript
/**
* Site object
* @type {{id: number, name: string, rank: number|string}}
*/
const site = {
id: 1,
name: "gfg",
rank: 1,
};
在上面的注释中,我们已经指定了一个对象的所有属性的类型。
函数/方法:
Javascript
/**
* Calculate age
* @param {number} current Current year
* @param {number} yearOfBirth Year of Birth
* @returns {string} your calculated age
*/
const calcAge = (current, yearOfBirth) => {
return `${current - yearOfBirth}`;
};
这里, @param标记用于记录函数的不同参数,而@returns标记记录函数的返回值
班级:
Javascript
/**
* Class to create new owner
*/
class Owner {
/**
* Owner details
* @param {Object} ownerDetail
*/
constructor(ownerDetail) {
/**
* @property {string} name Owner name
*/
this.name = ownerDetail.name;
/**
* @property {number} age Owner's age
*/
this.age = ownerDetail.age;
}
/**
* @property {Function} printOwner Prints Owner's details
* @returns {void}
*/
printOwner() {
console.log(`Owner's name is ${this.name}
and his age is ${this.age}`);
}
}
解释:
- 在类的声明之前,我们有 JSDoc 注释来描述类
- 对于 Constructor,使用类似于函数的 JSDoc 注释
- 在构造函数内部,为了记录变量,使用了@property标签
将类的实例链接到其类
由于我们已经创建了一个名为“Owner”的类,所以让我们创建该类的一个实例并在@link标签的帮助下将其链接到该类
Javascript
/**
* Link to Owner Class
* See {@link Owner}
*/
const gfg = new Owner({
name: "GeeksForGeeks",
age: 13,
});
最终index.js文件:
Javascript
// @ts-check
/**
* Site Name
* @type {string}
*/
const siteName = "GeeksForGeeks";
/**
* Number
* @type {number}
*/
const number = 1000;
/**
* Array
* @type {Array}
*/
const myArray = [10, 20, 30];
/**
* Site object
* @type {{id: number, name: string, rank: number|string}}
*/
const site = {
id: 1,
name: "gfg",
rank: 1,
};
/**
* Calculate age
* @param {number} current Current year
* @param {number} yearOfBirth Year of Birth
* @returns {string} your calculated age
*/
const calcAge = (current, yearOfBirth) => {
return `${current - yearOfBirth}`;
};
/**
* Class to create new owner
*/
class Owner {
/**
* Owner details
* @param {Object} ownerDetail
*/
constructor(ownerDetail) {
/**
* @property {string} name Owner name
*/
this.name = ownerDetail.name;
/**
* @property {number} age Owner's age
*/
this.age = ownerDetail.age;
}
/**
* @property {Function} printOwner Prints Owner's details
* @returns {void}
*/
printOwner() {
console.log(`Owner's name is ${this.name} and his age is ${this.age}`);
}
}
/**
* Link to Owner Class
* See {@link Owner}
*/
const gfg = new Owner({
name: "GeeksForGeeks",
age: 13,
});
输出:运行以下 jsdoc 命令:
npm run jsdoc
执行命令后,在 jsdoc 文件夹中会创建一个 index.html 页面,在浏览器中打开即可查看 jsdoc 生成的文档站点。
文档站点输出:
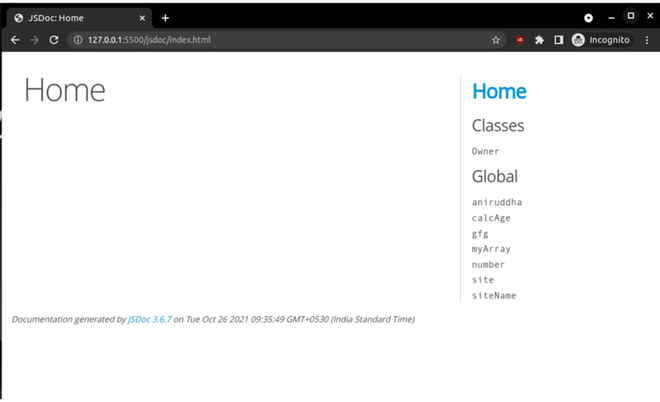
由 JSDoc 生成的文档站点的主页
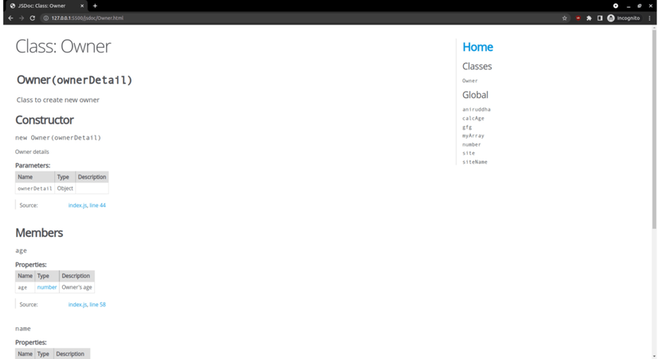
所有者类文档
JSDoc 注释中使用的一些常用标签:
- @author –记录代码的作者。
- @constant –记录常量。
- @default –允许记录赋予某些东西的默认值。
- @ 函数这个标签用来描述函数或方法。
- @global –记录全局对象。
- @implements – 记录接口的实现。
- 成员 –记录函数的成员。
- @module –用于记录 JavaScript 模块。
- @param –此标签用于记录函数的参数/参数。
- @returns –用于记录函数的返回值。
- @type –记录变量的类型