Python - 从父目录导入
在本文中,我们将学习如何从父目录导入模块。从Python 3.3 开始,不允许在父目录中引用或导入模块,从下面的示例中您可以清楚地了解这一点。
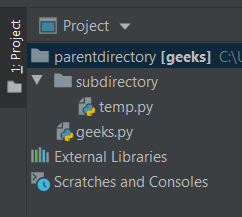
目录结构
在父目录下,我们有一个子目录geeks.py文件,在子目录下,我们有一个名为temp.py的Python文件,现在我们试试能否从temp.py文件中导入父目录下的geeks模块子目录。
geeks.py(父目录中的模块)
Python3
def geek_method():
print("This method in geeks module.......bye")
Python3
# importing the module in
# parent directory
from parentdirectory import geeks
# calling the func1() method
# from geeks module
geeks.geek_method()
Python3
import sys
# setting path
sys.path.append('../parentdirectory')
# importing
from parentdirectory.geeks import geek_method
# using
geek_method()
Python3
import path
import sys
# directory reach
directory = path.path(__file__).abspath()
# setting path
sys.path.append(directory.parent.parent)
# importing
from parentdirectory.geeks import geek_method
# using
geek_method()
Python3
import sys
import os
# getting the name of the directory
# where the this file is present.
current = os.path.dirname(os.path.realpath(__file__))
# Getting the parent directory name
# where the current directory is present.
parent = os.path.dirname(current)
# adding the parent directory to
# the sys.path.
sys.path.append(parent)
# now we can import the module in the parent
# directory.
import geeks
geeks.geek_method()
temp.py(子目录中的Python文件)
蟒蛇3
# importing the module in
# parent directory
from parentdirectory import geeks
# calling the func1() method
# from geeks module
geeks.geek_method()
正如我们之前讨论过的,不可能从父目录导入模块,所以这会导致类似这样的错误。
Traceback (most recent call last):
File “C:/Users/sai mohan pulamolu/Desktop/parentdirectory/subdirectory/temp.py”, line 2, in
from parentdirectory import geeks
ModuleNotFoundError: No module named ‘parentdirectory’
现在让我们学习如何从父目录导入模块:
为了导入模块,包含该模块的目录必须存在于 PYTHONPATH 中。它是一个环境变量,包含将由Python加载的包列表。 PYTHONPATH 中的软件包列表也存在于 sys.path 中,因此会将父目录路径添加到 sys.path 中。
在我们的工作中,我们使用三种不同的方法,下面通过示例进行解释。
方法一:这里我们会使用sys模块,直接设置需要的模块的路径。
使用append()方法将父目录添加到 sys.path。它是 sys 模块的内置函数,可以与路径变量一起使用来添加特定路径供解释器搜索。以下示例显示了如何做到这一点。
代码:
蟒蛇3
import sys
# setting path
sys.path.append('../parentdirectory')
# importing
from parentdirectory.geeks import geek_method
# using
geek_method()
输出:
This method in geeks module.......bye
方法二:这里我们将使用sys模块以及path模块来获取目录,并直接设置需要的模块的路径。
Syntax: os.path.abspath(path)
Parameter:
Path: A path-like object representing a file system path.
Return Type: This method returns a normalized version of the pathname path.
首先我们将使用path.path(__file__).abspath()获取temp.py文件当前所在目录的名称,然后将该目录添加到sys.path.append进行检查,我们将使用它的方法。
代码:
蟒蛇3
import path
import sys
# directory reach
directory = path.path(__file__).abspath()
# setting path
sys.path.append(directory.parent.parent)
# importing
from parentdirectory.geeks import geek_method
# using
geek_method()
输出:
This method in geeks module.......bye
方法三:这里我们将使用 sys 模块和 os 模块来获取目录(当前和父目录)并直接设置所需模块的路径。
Syntax: os.path.dirname(path)
Parameter:
path: A path-like object representing a file system path.
Return Type: This method returns a string value which represents the directory name from the specified path.
首先我们将使用 os.path.dirname(os.path.realpath(__file__)) 获取当前目录,其次我们将使用 os.path.dirname() 获取父目录,最后,添加父目录目录到 sys.path 来检查,我们将使用它的方法。
代码:
蟒蛇3
import sys
import os
# getting the name of the directory
# where the this file is present.
current = os.path.dirname(os.path.realpath(__file__))
# Getting the parent directory name
# where the current directory is present.
parent = os.path.dirname(current)
# adding the parent directory to
# the sys.path.
sys.path.append(parent)
# now we can import the module in the parent
# directory.
import geeks
geeks.geek_method()
输出: