Kotlin 中的文本切换器
Android TextSwitcher是一个用户界面小部件,包含多个 textView 并一次显示一个。 Textswitcher 是 View Switcher 的子类,用于为一个文本设置动画并显示下一个。
通常,我们在 XML 布局中手动使用 TextSwitcher 和在 Kotlin 文件中以编程方式使用两种方式。
我们应该定义一个 XML 组件,以便在我们的 android 应用程序中使用TextSwitcher 。
XML
XML
XML
TextSwitcherInKotlin
Next
Prev
Kotlin
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.Gravity
import android.view.animation.AnimationUtils
import android.widget.Button
import android.widget.TextSwitcher
import android.widget.TextView
import android.graphics.Color
class MainActivity : AppCompatActivity() {
private val languages = arrayOf("Java","Python","Kotlin","Scala","C++")
private var index = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// accessing the TextSwitcher from XML layout
val textSwitcher = findViewById(R.id.textSwitcher)
textSwitcher.setFactory {
val textView = TextView(this@MainActivity)
textView.gravity = Gravity.TOP or Gravity.CENTER_HORIZONTAL
textView.textSize = 32f
textView.setTextColor(Color.BLUE)
textView
}
textSwitcher.setText(languages[index])
val textIn = AnimationUtils.loadAnimation(
this, android.R.anim.slide_in_left)
textSwitcher.inAnimation = textIn
val textOut = AnimationUtils.loadAnimation(
this, android.R.anim.slide_out_right)
textSwitcher.outAnimation = textOut
// previous button functionality
val prev = findViewById
XML
首先,我们按照以下步骤创建一个新项目:
- 单击File ,然后单击New => New Project 。
- 之后包括 Kotlin 支持,然后单击下一步。
- 根据方便选择最小的 SDK,然后单击下一步按钮。
- 然后选择Empty activity => next => finish 。
TextSwitcher 小部件的不同属性
XML attributes | Description |
---|---|
android:id | Used to specify the id of the view. |
android:onClick | Used to specify the action when this view is clicked. |
android:background | Used to set the background of the view. |
android:padding | Used to set the padding of the view. |
android:visibilty | Used to set the visibility of the view. |
android:inAnimation | Used to define the animation to use when view is shown. |
android:outAnimation | Used to define the animation to use when view is hidden. |
android:animateFirstView | Used to define whether to animate the current view when the view animation is first displayed. |
修改activity_main.xml文件
在这个文件中,我们使用了 TextSwitcher、Buttons 并设置了它们的属性。
XML
更新字符串.xml 文件
在这里,我们使用字符串标签更新应用程序的名称。
XML
TextSwitcherInKotlin
Next
Prev
在 MainActivity.kt 文件中访问 TextSwitcher
首先,我们声明一个数组语言,其中包含用于 textView 的语言列表。
private val textList = arrayOf("Java","Python","Kotlin","Scala","C++")
然后,我们从 XML 布局中访问TextSwitcher并设置文本颜色、文本大小等属性。
val textSwitcher = findViewById(R.id.textSwitcher)
科特林
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.Gravity
import android.view.animation.AnimationUtils
import android.widget.Button
import android.widget.TextSwitcher
import android.widget.TextView
import android.graphics.Color
class MainActivity : AppCompatActivity() {
private val languages = arrayOf("Java","Python","Kotlin","Scala","C++")
private var index = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// accessing the TextSwitcher from XML layout
val textSwitcher = findViewById(R.id.textSwitcher)
textSwitcher.setFactory {
val textView = TextView(this@MainActivity)
textView.gravity = Gravity.TOP or Gravity.CENTER_HORIZONTAL
textView.textSize = 32f
textView.setTextColor(Color.BLUE)
textView
}
textSwitcher.setText(languages[index])
val textIn = AnimationUtils.loadAnimation(
this, android.R.anim.slide_in_left)
textSwitcher.inAnimation = textIn
val textOut = AnimationUtils.loadAnimation(
this, android.R.anim.slide_out_right)
textSwitcher.outAnimation = textOut
// previous button functionality
val prev = findViewById(R.id.prev)
prev.setOnClickListener {
index = if (index - 1 >= 0) index - 1 else 4
textSwitcher.setText(languages[index])
}
// next button functionality
val button = findViewById(R.id.next)
button.setOnClickListener {
index = if (index + 1 < languages.size) index + 1 else 0
textSwitcher.setText(languages[index])
}
}
}
AndroidManifest.xml 文件
XML
作为模拟器运行:
单击下一步按钮,然后我们在 TextView 中获取其他文本。
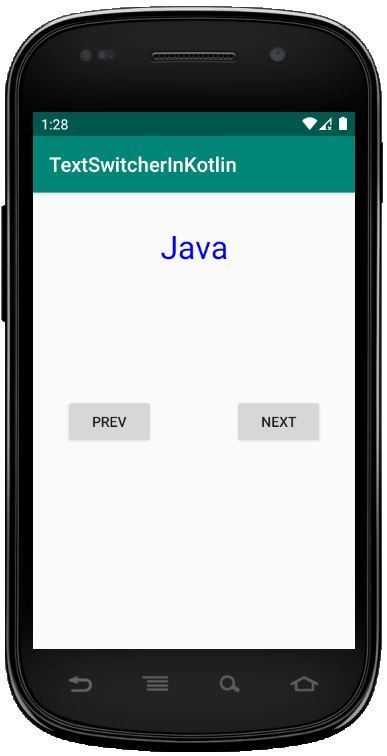
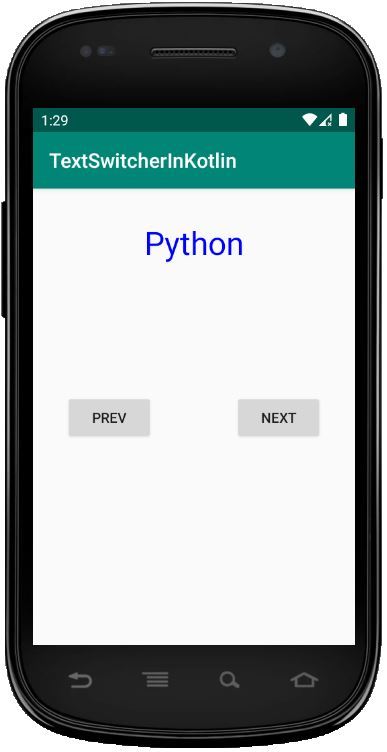
在评论中写代码?请使用 ide.geeksforgeeks.org,生成链接并在此处分享链接。