Python Psycopg2 - 获取刚刚插入的行的 ID
在本文中,我们将了解如何在Python中使用 pyscopg2 获取刚刚插入的行的 ID
建立与 PostgreSQL 服务器的连接
为了建立到 PostgreSQL 服务器的连接,我们将使用Python中的 pscopg2 库。您可以使用以下命令安装 psycopg2:
pip install psycopg2
安装库后,可以使用以下代码创建与数据库服务器的连接:
Python3
import psycopg2
def get_connection():
try:
return psycopg2.connect(
database="postgres",
user="postgres",
password="password",
host="127.0.0.1",
port=5432,
)
except:
return False
conn = get_connection()
if conn:
print("Connection to the PostgreSQL established successfully.")
else:
print("Connection to the PostgreSQL encountered and error.")
Python3
# This program uses fetchone()
# to get the row ID of the latest
# entry in the table
import psycopg2
def get_connection():
try:
return psycopg2.connect(
database="postgres",
user="postgres",
password="password",
host="127.0.0.1",
port=5432,
)
except:
return False
# GET THE CONNECTION OBJECT
conn = get_connection()
# CREATE A CURSOR USING THE CONNECTION OBJECT
curr = conn.cursor()
# EXECUTE THE SQL QUERY
curr.execute('''
INSERT INTO public.USER(user_name, email)
VALUES ('Sudha Tiwari', 'sudha@xyz.com')
RETURNING user_id;
''')
# FETCH THE LATEST USER ID USING THE CURSOR
data = curr.fetchone()
print("User ID of latest entry:", data[0])
# CLOSE THE CONNECTION
conn.close()
如果提供的数据库凭据正确并且成功建立连接,则运行上述代码将产生以下结果:
Connection to the PostgreSQL established successfully.
为演示创建表
我们将创建一个演示表 `user` 用于理解本文。您可以使用以下查询生成示例“用户”表。
CREATE TABLE public.user (
user_id SERIAL PRIMARY KEY,
user_name VARCHAR(50) NOT NULL,
email VARCHAR(50) NOT NULL
);
INSERT INTO public.USER(user_name, email) VALUES (‘Amit Pathak’, ‘amit@xyz.com’);
INSERT INTO public.USER(user_name, email) VALUES (‘Ashish Mysterio’, ‘ashish@xyz.com’);
INSERT INTO public.USER(user_name, email) VALUES (‘Priyanka Pandey’, ‘priyanka@xyz.com’);
执行上述查询后,`user` 表如下所示:
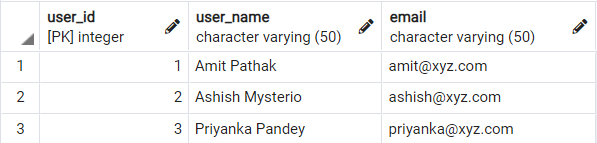
用户表(代码运行前)
下面是实现
Python3
# This program uses fetchone()
# to get the row ID of the latest
# entry in the table
import psycopg2
def get_connection():
try:
return psycopg2.connect(
database="postgres",
user="postgres",
password="password",
host="127.0.0.1",
port=5432,
)
except:
return False
# GET THE CONNECTION OBJECT
conn = get_connection()
# CREATE A CURSOR USING THE CONNECTION OBJECT
curr = conn.cursor()
# EXECUTE THE SQL QUERY
curr.execute('''
INSERT INTO public.USER(user_name, email)
VALUES ('Sudha Tiwari', 'sudha@xyz.com')
RETURNING user_id;
''')
# FETCH THE LATEST USER ID USING THE CURSOR
data = curr.fetchone()
print("User ID of latest entry:", data[0])
# CLOSE THE CONNECTION
conn.close()
输出:
User ID of latest entry: 4
在上面的例子中,我们首先创建了一个 psycopg2 连接对象,并且使用这个对象,我们创建了一个游标来提交我们的查询。表中已经有 3 个条目,其中 ` user_id`分别为 1、2 和 3。对于表中的任何新条目,` user_id`字段将递增,因为默认情况下它是一个自动递增字段。因此,新记录的 ` user_id`应该是 4。这就是我们可以从输出中看到的。该查询使用一个额外的 RETURNING 子句,它返回所需的列值,就像任何回调一样工作。然后我们使用fetchone()方法来获取最新执行的查询的响应,该查询给出了所需的行 ID。 `user` 表现在有以下记录——
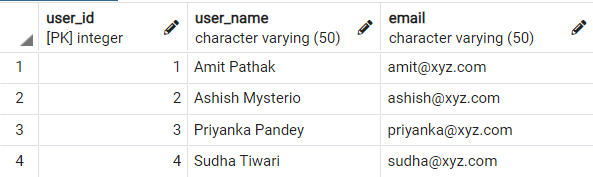
用户表(代码运行后)