给定两个整数A和B,它们表示椭圆的半长轴和半短轴的长度,且通式为(x 2 / A 2 )+(y 2 / B 2 )= 1 ,因此任务是求出长度椭圆的直肠
例子:
Input: A = 3, B = 2
Output: 2.66666
Input: A = 6, B = 3
Output: 3
方法:可以根据以下观察结果解决给定问题:
- The Latus Rectum of an Ellipse is the focal chord perpendicular to the major axis whose length is equal to:
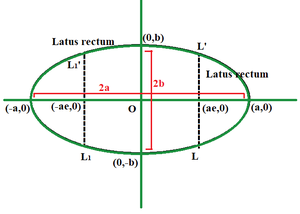
Ellipse
- Length of major axis is 2A.
- Length of minor axis is 2B.
- Therefore, the length of the latus rectum is:
请按照以下步骤解决给定的问题:
- 初始化两个变量,说主要和次要,以分别存储长轴(= 2A)和短轴(= 2B)的椭圆的长度的长度。
- 计算小平方 并按专业划分。将结果存储在一个双变量中,例如latus_rectum 。
- 打印latus_rectum的值作为最终结果。
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function to calculate the length
// of the latus rectum of an ellipse
double lengthOfLatusRectum(double A,
double B)
{
// Length of major axis
double major = 2.0 * A;
// Length of minor axis
double minor = 2.0 * B;
// Length of the latus rectum
double latus_rectum = (minor*minor)/major;
return latus_rectum;
}
// Driver Code
int main()
{
// Given lengths of semi-major
// and semi-minor axis
double A = 3.0, B = 2.0;
// Function call to calculate length
// of the latus rectum of a ellipse
cout << lengthOfLatusRectum(A, B);
return 0;
}
Java
// Java program for the above approach
import java.util.*;
class GFG{
// Function to calculate the length
// of the latus rectum of an ellipse
static double lengthOfLatusRectum(double A,
double B)
{
// Length of major axis
double major = 2.0 * A;
// Length of minor axis
double minor = 2.0 * B;
// Length of the latus rectum
double latus_rectum = (minor * minor) / major;
return latus_rectum;
}
// Driver code
public static void main(String[] args)
{
// Given lengths of semi-major
// and semi-minor axis
double A = 3.0, B = 2.0;
// Function call to calculate length
// of the latus rectum of a ellipse
System.out.print(lengthOfLatusRectum(A, B));
}
}
// This code is contributed by susmitakundugoaldanga
Python3
# Python3 program for the above approach
# Function to calculate the length
# of the latus rectum of an ellipse
def lengthOfLatusRectum(A, B):
# Length of major axis
major = 2.0 * A
# Length of minor axis
minor = 2.0 * B
# Length of the latus rectum
latus_rectum = (minor*minor)/major
return latus_rectum
# Driver Code
if __name__ == "__main__":
# Given lengths of semi-major
# and semi-minor axis
A = 3.0
B = 2.0
# Function call to calculate length
# of the latus rectum of a ellipse
print('%.5f' % lengthOfLatusRectum(A, B))
# This code is contributed by ukasp.
C#
// C# program for the above approach
using System;
class GFG
{
// Function to calculate the length
// of the latus rectum of an ellipse
static double lengthOfLatusRectum(double A,
double B)
{
// Length of major axis
double major = 2.0 * A;
// Length of minor axis
double minor = 2.0 * B;
// Length of the latus rectum
double latus_rectum = (minor*minor)/major;
return latus_rectum;
}
// Driver Code
public static void Main()
{
// Given lengths of semi-major
// and semi-minor axis
double A = 3.0, B = 2.0;
// Function call to calculate length
// of the latus rectum of a ellipse
Console.WriteLine(lengthOfLatusRectum(A, B));
}
}
// This code is contributed by souravghosh0416.
Javascript
输出:
2.66667
时间复杂度: O(1)
辅助空间: O(1)