在计算机编程中,循环用于重复代码块。例如,如果您想显示一条消息100次,而不是键入相同的代码100次,则可以使用循环。
在Java中,存在三种类型的循环。
- for循环
- while循环
- 做… while循环
本教程重点介绍for循环。您将在即将到来的教程中了解其他类型的循环。
Java for循环
Java for
循环用于运行代码块一定次数。 for
循环的语法为:
for (initialExpression; testExpression; updateExpression) {
// body of the loop
}
这里,
- initialExpression初始化和/或声明变量,并且仅执行一次。
- 条件被评估。如果条件为
true
,则执行for
循环的主体。 - 该updateExpression更新initialExpression的价值。
- 再次评估条件 。该过程一直持续到条件为
false
为止。
要了解有关条件的更多信息,请访问Java关系和逻辑运算符。
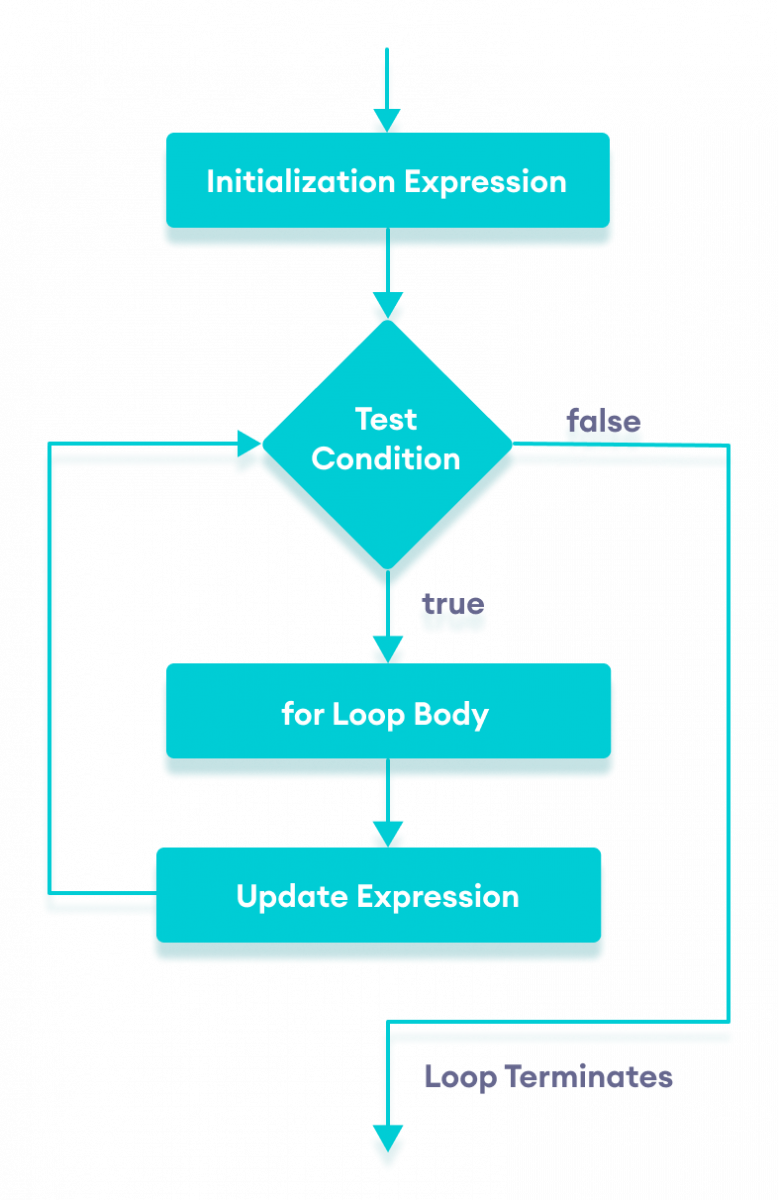
示例1:显示文本五次
// Program to print a text 5 times
class Main {
public static void main(String[] args) {
int n = 5;
// for loop
for (int i = 1; i <= n; ++i) {
System.out.println("Java is fun");
}
}
}
输出
Java is fun
Java is fun
Java is fun
Java is fun
Java is fun
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1 n = 5 |
true |
Java is fun is printed. i is increased to 2. |
2nd | i = 2 n = 5 |
true |
Java is fun is printed. i is increased to 3. |
3rd | i = 3 n = 5 |
true |
Java is fun is printed. i is increased to 4. |
4th | i = 4 n = 5 |
true |
Java is fun is printed. i is increased to 5. |
5th | i = 5 n = 5 |
true |
Java is fun is printed. i is increased to 6. |
6th | i = 6 n = 5 |
false |
The loop is terminated. |
示例2:显示从1到5的数字
// Program to print numbers from 1 to 5
class Main {
public static void main(String[] args) {
int n = 5;
// for loop
for (int i = 1; i <= n; ++i) {
System.out.println(i);
}
}
}
输出
1
2
3
4
5
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1 n = 5 |
true |
1 is printed. i is increased to 2. |
2nd | i = 2 n = 5 |
true |
2 is printed. i is increased to 3. |
3rd | i = 3 n = 5 |
true |
3 is printed. i is increased to 4. |
4th | i = 4 n = 5 |
true |
4 is printed. i is increased to 5. |
5th | i = 5 n = 5 |
true |
5 is printed. i is increased to 6. |
6th | i = 6 n = 5 |
false |
The loop is terminated. |
示例3:显示n个自然数的总和
// Program to find the sum of natural numbers from 1 to 1000.
class Main {
public static void main(String[] args) {
int sum = 0;
int n = 1000;
// for loop
for (int i = 1; i <= n; ++i) {
// body inside for loop
sum += i; // sum = sum + i
}
System.out.println("Sum = " + sum);
}
}
输出 :
Sum = 500500
在这里, sum的值最初为0 。然后,for循环从i = 1 to 1000
迭代i = 1 to 1000
。在每次迭代中,将i加到sum上,并将其值增加1 。
当i变为1001时 ,测试条件为false
, 总和等于0 + 1 + 2 + .... + 1000
。
上面的程序加上自然数的和也可以写成
// Program to find the sum of natural numbers from 1 to 1000.
class Main {
public static void main(String[] args) {
int sum = 0;
int n = 1000;
// for loop
for (int i = n; i >= 1; --i) {
// body inside for loop
sum += i; // sum = sum + i
}
System.out.println("Sum = " + sum);
}
}
该程序的输出与示例3相同。
Java for-each循环
Java for循环具有另一种语法,可以轻松地遍历数组和集合。例如,
// print array elements
class Main {
public static void main(String[] args) {
// create an array
int[] numbers = {3, 7, 5, -5};
// iterating through the array
for (int number: numbers) {
System.out.println(number);
}
}
}
输出
3
4
5
-5
在这里,我们使用了for-each循环来逐一打印数字数组的每个元素。
在循环的第一次迭代中, 数字将为3,在第二次迭代中, 数字将为7,依此类推。
要了解更多信息,请访问Java for-each循环。
Java无限循环
如果将测试表达式设置为永远不会为false
,则for
循环将永远运行。这称为无限循环。例如,
// Infinite for Loop
class Infinite {
public static void main(String[] args) {
int sum = 0;
for (int i = 1; i <= 10; --i) {
System.out.println("Hello");
}
}
}
在这里,测试表达式i <= 10
永远不会为false
并且Hello
会重复打印,直到内存用完。