在Python中使用 Turtle 绘制带有月亮的星空
先决条件: Python中的海龟编程
在Python中,Turtle 是一个内置库,它使我们可以绘制不同的图案、形状或任何我们想要的东西,但为此,我们必须了解 Turtle 库提供的不同功能的用途。
在这篇文章中,我们将学习如何绘制星空。也许这篇文章很长,但在阅读整篇文章后,您可以轻松理解每一步。
为此,我们使用了海龟库和随机模块来生成随机位置来绘制星星。
下面列出了我们用来绘制星空的方法。METHOD PARAMETER DESCRIPTION Turtle() None It creates and returns a new turtle object. bgcolor color name fills the color in the background of the window circle() radius, extent, steps Draw a circle with given radius. title() name Gives name to the turtle window forward() / fd() amount It moves the turtle forward by the specified amount. Screen() None After passing this method turtle screen will be active speed value Decides the speed of the pen up() None Picks up the turtle’s Pen. down() None Picks down the turtle’s Pen. left() angle It turns the turtle counter clockwise. right() angle It turns the turtle clockwise. goto() x, y It moves the turtle to position x, y. begin_fill() None Call just before drawing a shape to be filled. Equivalent to fill(True). end_fill() None Fill the shape drawn after the last call to begin_fill(). Equivalent to fill(False). hideturtle() None Hides the turtle after finishing the drawing. exitonclick() None On clicking exit the turtle screen. randint start, end value Generates random values with in the given range.
我们的星空是由许多星星和一个圆形的月亮组成的。所以,我们必须首先学习如何绘制星星和圆圈。
让我们首先学习如何通过我们的代码绘制星星。
Python
# importing turtle library
import turtle
# creating turtle object
t = turtle.Turtle()
# to activate turtle graphics screen
w = turtle.Screen()
# speed of turtle's pen
t.speed(0)
# creating star
for i in range(0, 5):
t.fd(200)
t.right(144)
# after clicking turtle graphics screen
# will be terminated
w.exitonclick()
Python
# importing turtle library
import turtle
# creating turtle object
t = turtle.Turtle()
# to activate turtle graphics Screen
w = turtle.Screen()
# speed of turtle's pen
t.speed()
# Creating circle
t.circle(100)
# after clicking turtle graphics screen
# will be terminated
w.exitonclick()
Python
# importing libraries
import turtle
import random
# creating turtle object
t = turtle.Turtle()
# to activate turtle graphics Screen
w = turtle.Screen()
# setting speed of turtle
t.speed(0)
# giving the background color of turtle
# graphics screen
w.bgcolor("black")
# giving the color of pen to our turtle
# for drawing
t.color("white")
# giving title to our turtle graphics window
w.title("Starry Sky")
# making function to draw the stars
def stars():
for i in range(5):
t.fd(10)
t.right(144)
# loop for making number of stars
for i in range(100):
# generating random integer values for x and y
x = random.randint(-640, 640)
y = random.randint(-330, 330)
# calling the function stars to draw the
# stars at random x,y value
stars()
# took up the turtle's pen
t.up()
# go at the x,y coordinate generated above
t.goto(x, y)
# took down the pen to draw
t.down()
# for making our moon tooking up the pen
t.up()
# going at the specific coordinated
t.goto(0, 170)
# took down the pen to start drawing
t.down()
# giving color to turtle's pen
t.color("white")
# start filling the color
t.begin_fill()
# making our moon
t.circle(80)
# stop filling the color
t.end_fill()
# after drawing hidding the turtle from
# the window
t.hideturtle()
# terminated the window after clicking
w.exitonclick()
输出:
在上面的代码中,我们从 0 到 5 的范围内运行 for 循环,因为我们都知道星星有 5 条边,两条边之间的角度将是 144 度,以制作完美的星星,这就是我们在 t.right 行中所做的(144) 我们以 144 度角移动海龟的头部,线 t.fd(200) 决定了我们星星的大小(值越小=星星越小)
现在,我们将学习如何通过我们的代码绘制圆。
Python
# importing turtle library
import turtle
# creating turtle object
t = turtle.Turtle()
# to activate turtle graphics Screen
w = turtle.Screen()
# speed of turtle's pen
t.speed()
# Creating circle
t.circle(100)
# after clicking turtle graphics screen
# will be terminated
w.exitonclick()
输出:
在上面的代码中,为了制作一个圆,我们使用了海龟库提供的 circle函数,只传递了定义圆半径的参数 100。运行我们的代码后,我们得到了我们的圈子。
所以,最后,我们学会了如何画星星和圆圈。
现在我们要画我们的星空。
Python
# importing libraries
import turtle
import random
# creating turtle object
t = turtle.Turtle()
# to activate turtle graphics Screen
w = turtle.Screen()
# setting speed of turtle
t.speed(0)
# giving the background color of turtle
# graphics screen
w.bgcolor("black")
# giving the color of pen to our turtle
# for drawing
t.color("white")
# giving title to our turtle graphics window
w.title("Starry Sky")
# making function to draw the stars
def stars():
for i in range(5):
t.fd(10)
t.right(144)
# loop for making number of stars
for i in range(100):
# generating random integer values for x and y
x = random.randint(-640, 640)
y = random.randint(-330, 330)
# calling the function stars to draw the
# stars at random x,y value
stars()
# took up the turtle's pen
t.up()
# go at the x,y coordinate generated above
t.goto(x, y)
# took down the pen to draw
t.down()
# for making our moon tooking up the pen
t.up()
# going at the specific coordinated
t.goto(0, 170)
# took down the pen to start drawing
t.down()
# giving color to turtle's pen
t.color("white")
# start filling the color
t.begin_fill()
# making our moon
t.circle(80)
# stop filling the color
t.end_fill()
# after drawing hidding the turtle from
# the window
t.hideturtle()
# terminated the window after clicking
w.exitonclick()
输出:

星空
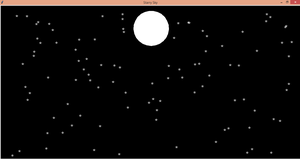
在上面的代码中,您将在阅读每行代码之前的注释后了解我们的代码如何工作并绘制星空的清晰概念。