使用列表Python .docx 模块
先决条件:使用 .docx 模块
Word 文档包含包装在三个对象级别内的格式化文本。最低级-运行对象,中间级-段落对象,和最高级-文档对象。因此,我们无法使用普通文本编辑器处理这些文档。但是,我们可以使用 python-docx 模块在Python中操作这些 word 文档。安装这个模块的pip命令是:
pip install python-docx
Python docx 模块允许用户通过操作现有文档或创建新的空文档并对其进行操作来操作文档。它是一个强大的工具,因为它可以帮助您在非常大的范围内操作文档。有两种类型的列表:
- 有序列表
- 无序列表
要在 Word 文档中添加有序/无序列表,文档对象的 .add_paragraph() 方法中有可用的样式。
Syntax: doc.add_paragraph(String s, style=None)
Parameters:
- String s: It is the string data that is to be added as a paragraph. This string can contain newline character ‘\n’, tabs ‘\t’ or a carriage return character ‘\r’.
- Style: It is used to set style.
有序列表
添加有序列表的样式是: Sr. No. Style Name Description 1. List Number It adds an ordered list in the word document. 2. List Number 2 It adds an ordered list with a single tab indentation in the word document. 3. List Number 3 It adds an ordered list with double tab indentation in the word document.
注意:每个列表点都被视为列表中的一个段落,因此您必须将每个点添加为具有相同样式名称的新段落。
示例1:在Word文档中添加一个有序列表。
Python3
# Import docx NOT python-docx
import docx
# Create an instance of a word document
doc = docx.Document()
# Add a Title to the document
doc.add_heading('GeeksForGeeks', 0)
# Adding list of style name 'List Number'
doc.add_heading('Style: List Number', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an ordered list.',
style='List Number')
doc.add_paragraph('The second item in an ordered list.',
style='List Number')
doc.add_paragraph('The third item in an ordered list.',
style='List Number')
# Adding list of style name 'List Number 2'
doc.add_heading('Style: List Number 2', 3)
# Adding points to the list named 'List Number 2'
doc.add_paragraph('The first item in an ordered list.',
style='List Number 2')
doc.add_paragraph('The second item in an ordered list.',
style='List Number 2')
doc.add_paragraph('The third item in an ordered list.',
style='List Number 2')
# Adding list of style name 'List Number 3'
doc.add_heading('Style: List Number 3', 3)
# Adding points to the list named 'List Number 3'
doc.add_paragraph('The first item in an ordered list.',
style='List Number 3')
doc.add_paragraph('The second item in an ordered list.',
style='List Number 3')
doc.add_paragraph('The third item in an ordered list.',
style='List Number 3')
# Now save the document to a location
doc.save('gfg.docx')
Python3
# Import docx NOT python-docx
import docx
# Create an instance of a word document
doc = docx.Document()
# Add a Title to the document
doc.add_heading('GeeksForGeeks', 0)
# Adding list of style name 'List Bullet'
doc.add_heading('Style: List Bullet', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet')
# Adding list of style name 'List Bullet 2'
doc.add_heading('Style: List Bullet 2', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet 2')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet 2')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet 2')
# Adding list of style name 'List Bullet 3'
doc.add_heading('Style: List Bullet 3', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet 3')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet 3')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet 3')
# Now save the document to a location
doc.save('gfg.docx')
输出:
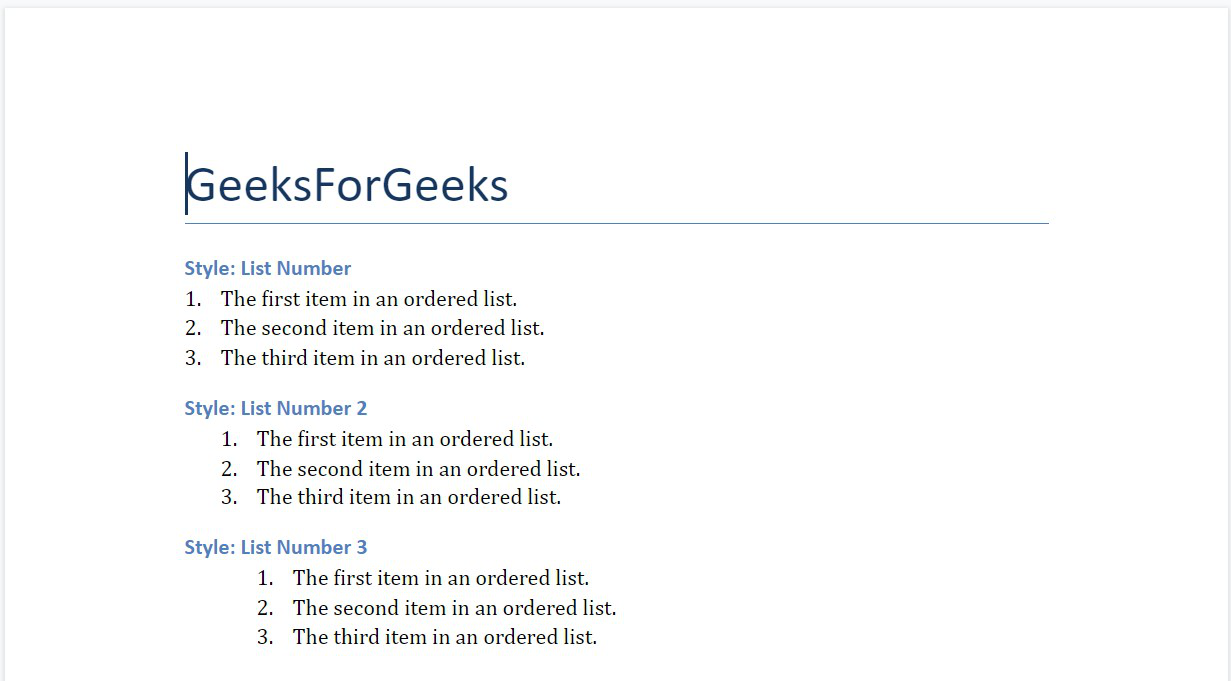
文档 gfg.docx
无序列表
添加无序列表的样式是: Sr. No. Style Name Description 1. List Bullet It adds an unordered list in the word document. 2. List Bullet 2 It adds an unordered list with a single tab indentation in the word document. 3. List Bullet 3 It adds an unordered list with a double tab indentation in the word document.
注意:每个列表点都被视为列表中的一个段落,因此您必须将每个点添加为具有相同样式名称的新段落。
示例2:在Word文档中添加一个无序列表。
蟒蛇3
# Import docx NOT python-docx
import docx
# Create an instance of a word document
doc = docx.Document()
# Add a Title to the document
doc.add_heading('GeeksForGeeks', 0)
# Adding list of style name 'List Bullet'
doc.add_heading('Style: List Bullet', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet')
# Adding list of style name 'List Bullet 2'
doc.add_heading('Style: List Bullet 2', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet 2')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet 2')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet 2')
# Adding list of style name 'List Bullet 3'
doc.add_heading('Style: List Bullet 3', 3)
# Adding points to the list named 'List Number'
doc.add_paragraph('The first item in an unordered list.',
style='List Bullet 3')
doc.add_paragraph('The second item in an unordered list.',
style='List Bullet 3')
doc.add_paragraph('The third item in an unordered list.',
style='List Bullet 3')
# Now save the document to a location
doc.save('gfg.docx')
输出:
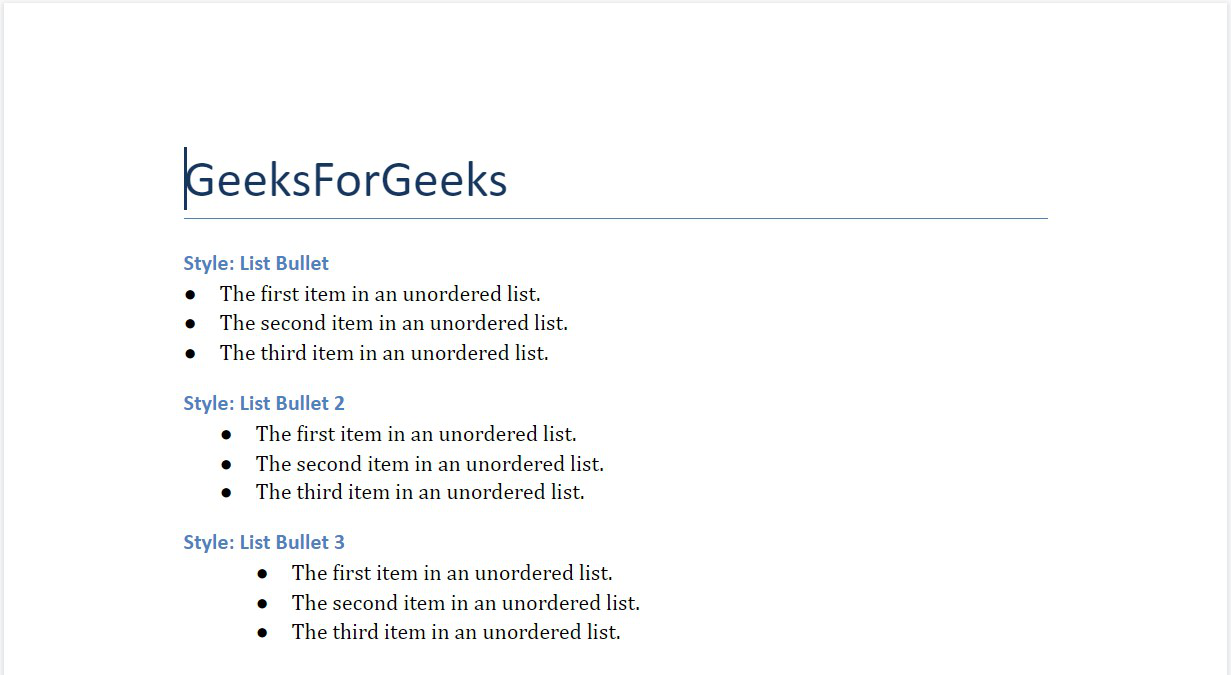
文档 gfg.docx