锈——铸造
Rust 中的类型转换或强制转换是一种将一种类型转换为另一种类型的方法。顾名思义,类型转换是将变量从一种数据类型转换为另一种数据类型的过程。因此,编译器将变量视为新的数据类型。 Rust 不允许我们在原始类型之间隐式转换数据类型。
原始类型是 i8、i16、i32、i64、u8、u16、u32、u64、f32、f64、char 和 bool。这里 i8、i16、i32、i64 是有符号整数类型,u8、u16、u32、u64 是无符号整数类型。 f32 和 f64 是浮点类型。 char 是单字符类型,bool 是布尔类型。
该术语隐含意味着编译器不需要我们编写代码来转换数据类型。编译器会自动执行此操作。但是 rust 不允许我们在原始类型之间隐式转换数据类型。我们必须使用as关键字显式转换数据类型。
铸件类型:
- 隐式类型转换
- 显式类型转换
1.隐式类型转换:
隐式类型转换是将一种数据类型转换为另一种数据类型的过程。编译器会自动执行此操作。
Rust
// Implicit type casting results in an error
fn main() { // main function
// declaring the variable with float datatype
let x = 1000.456456154651;
// converting the float datatype to integer datatype
// results in an error
let y: i32 = x;
println!("The value of y is: {}", y);
}
Rust
// Rust program to convert the datatype from i32 to u32
// function to print the type of a variable
fn print_type_of(_: &T) {
println!("{}", std::any::type_name::())
}
fn main() { // main function
// declare x as i32
let x: i32 = 100000;
// explicitly convert the datatype from i32 to u32
let y: u32 = x as u32;
// print the type of x
println!("Before Type Conversion of x ");
print_type_of(&x);
// print the type of y
println!("After Type Conversion of x ");
print_type_of(&y);
}
Rust
// Rust program to convert the datatype from i32 to u32
// function to print the type of a variable
fn print_type_of(_: &T) {
println!("{}", std::any::type_name::())
}
// type casting
fn main() {
// declare x as i32
let decimal_value = 65.4321_f32;
// converting to integer and truncating
let integer_value = decimal_value as u8;
// converting integer to character using ascii table
let character_value = integer_value as char;
// printing the values and their datatype
println!("The value of decimal_value is: {} and its datatype is ", decimal_value);
print_type_of(&decimal_value);
println!("The value of integer_value is: {} and its datatype is ", integer_value);
print_type_of(&integer_value);
println!("The value of character_value is: {} and its datatype is", character_value);
print_type_of(&character_value);
// printing the type of the variables
println!("Casting from {} -> {} -> {}", decimal_value, integer_value, character_value);
}
输出:
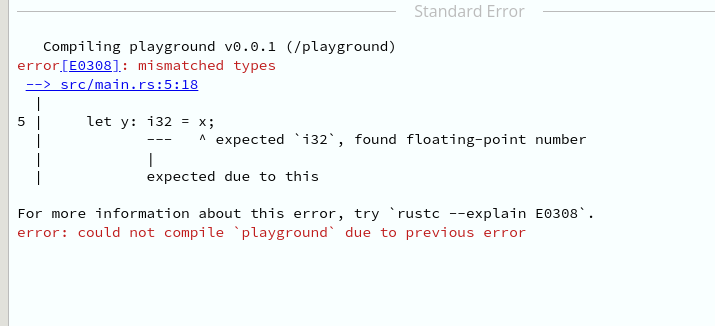
Rust 不允许隐式类型转换
Rust 编译器不能简单地将浮点数据类型转换为整数数据类型,我们必须显式转换数据类型。因此,如果我们尝试运行上面的代码,就会报错。
2.显式类型转换:
显式类型转换可以通过使用as关键字来完成。 as关键字用于安全地转换数据类型。编译器检查数据类型是否正确。如果数据类型正确,则编译器转换数据类型。如果数据类型不正确,则编译器会出错。为了进行安全的转换,应该从低数据类型到高数据类型进行类型转换。
示例 1:
锈
// Rust program to convert the datatype from i32 to u32
// function to print the type of a variable
fn print_type_of(_: &T) {
println!("{}", std::any::type_name::())
}
fn main() { // main function
// declare x as i32
let x: i32 = 100000;
// explicitly convert the datatype from i32 to u32
let y: u32 = x as u32;
// print the type of x
println!("Before Type Conversion of x ");
print_type_of(&x);
// print the type of y
println!("After Type Conversion of x ");
print_type_of(&y);
}
输出:
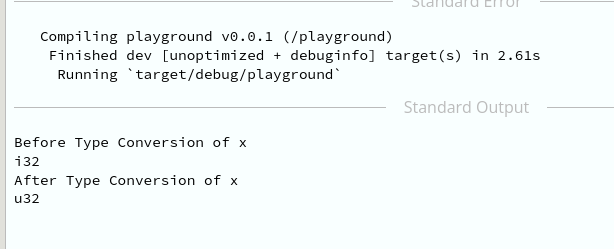
从 i32 到 u32 的类型转换
示例 2:
锈
// Rust program to convert the datatype from i32 to u32
// function to print the type of a variable
fn print_type_of(_: &T) {
println!("{}", std::any::type_name::())
}
// type casting
fn main() {
// declare x as i32
let decimal_value = 65.4321_f32;
// converting to integer and truncating
let integer_value = decimal_value as u8;
// converting integer to character using ascii table
let character_value = integer_value as char;
// printing the values and their datatype
println!("The value of decimal_value is: {} and its datatype is ", decimal_value);
print_type_of(&decimal_value);
println!("The value of integer_value is: {} and its datatype is ", integer_value);
print_type_of(&integer_value);
println!("The value of character_value is: {} and its datatype is", character_value);
print_type_of(&character_value);
// printing the type of the variables
println!("Casting from {} -> {} -> {}", decimal_value, integer_value, character_value);
}
输出:
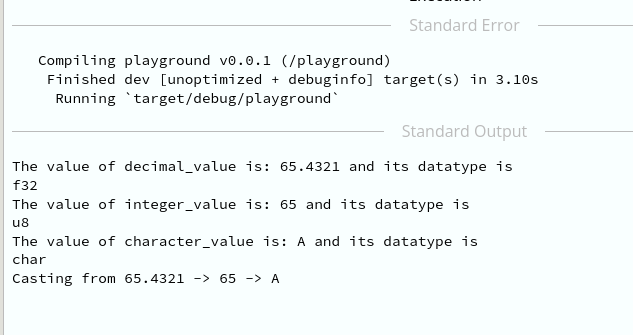
类型转换