Spring Boot – 开发工具
由于 Spring Framework 提供的丰富功能,它日益流行。它于 2003 年发布。 Spring Framework 基本上是一组子框架或子框架的集合。我们需要编写大量代码来手动设置 Spring 应用程序的配置。为了克服这种乏味和繁琐的工作,基于 Spring Framework 构建的 Spring Boot 于 2014 年发布。Spring Boot 框架的主要功能是其“自动配置”功能。这使开发人员能够更快地构建应用程序。 Spring Boot 的另一个关键特性是它的 DevTools 库。
Pre-requisite: It is important to understand that the ‘DevTools’ is not an IDE plugin, nor does it require that you should use a specific IDE.
Spring Boot – 开发工具
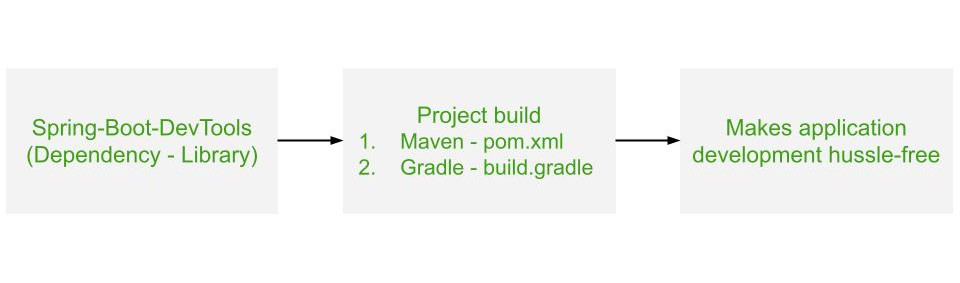
Spring Boot 的工作原理 – DevTools
DevTools – 顾名思义,它为 Spring 开发人员提供了一些开发时间工具,可以简化开发过程。其中一些重要的工作如下 -
1.自动应用重启
- 当我们对Java代码或属性文件进行更改时,使用 DevTools,应用程序会使用新的更改进行更新。
- 它监视更改并自动重新启动应用程序。
- 使用应用程序类路径中的 DevTools,应用程序被加载到Java虚拟机中的两个不同的类加载器中。
- 一个类加载器包含应用程序需要的所有依赖项(库)。这些不太可能经常改变。
- 第二个类加载器包含您的实际Java代码、属性文件等,特别是在 (src/main/path) 中,它们可能会经常更改。
- 在检测到更改时,DevTools 仅在类加载器上方重新加载,并重新启动 Spring 应用程序上下文,并保持另一个类加载器 JVM 完好无损。
- 这将有助于减少启动应用程序的时间。
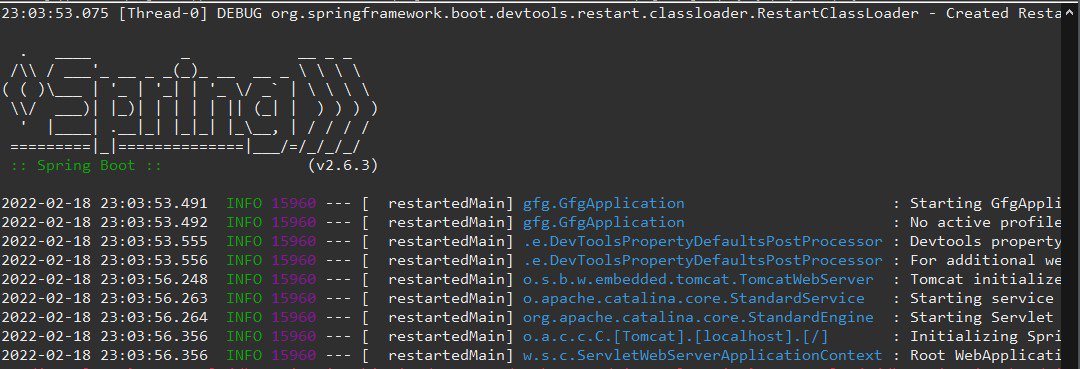
安慰
- Devtools 提供了一种配置不与任何应用程序耦合的全局设置的方法。该文件名为 .spring-boot-devtools.properties,位于 $HOME。
- 如果未手动配置,则 DevTools 将自动使用默认值。
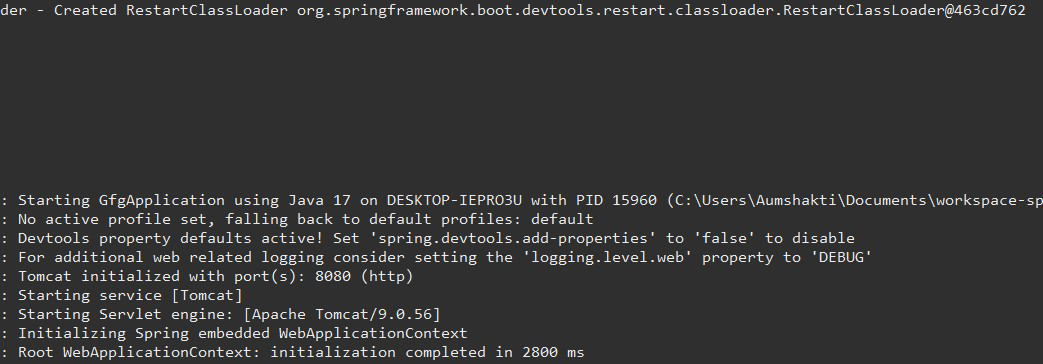
安慰
Note: On automatic application restart, the changes to dependencies won’t be available. Because the respective class loader isn’t automatically reloaded. Therefore, after making the changes to dependencies, you have manually restart the application to get those changes in effect.
2.自动禁用模板缓存
- Spring Boot 提供了各种模板选项,例如 Thymeleaf、Groovy、FreeMarker 等。
- 这些模板被配置为自动缓存模板解析的结果。
- 因此,无需针对它们服务的每个请求重新解析它们。
- 缓存模板使得在应用程序运行时很难对模板进行更改,并在刷新浏览器后查看所需的更改。
- 即使在应用更改后,缓存的模板仍将被使用,直到您重新启动应用程序。
- 从开发的角度来看,这使得缓存模板很差。
- DevTools 通过自动禁用所有模板缓存来帮助我们解决这个问题。
- 因此,您应用的更改现在将在刷新浏览器后反映在您的模板上。
Note:
- You can additionally turn on/off caching of templates by specifying ‘spring.thymeleaf.cache=true/false‘ in the ‘application.properties’ file.
- You can also override other properties as well in the same above file.
3. 自动浏览器刷新
- 如果我们对代码做了一些修改并尝试保存,那么我们每次都要点击浏览器上的刷新按钮才能看到它的效果。
- 这个过程会自动变得耗时。在浏览器中见证结果并一次又一次地跳过浏览器的刷新以减少开发时间总是有用的。
- DevTools 通过为您跳过这项繁琐的工作来减轻我们的负担。
- 当我们嵌入和使用 DevTools 时,它会自动启用“LiveReload”服务器以及您的应用程序。
- LiveReload 服务器用处不大,但与相应的 LiveReload 浏览器插件结合使用时很有用。
- 当对浏览器直接或间接提供的文件进行更改时,这会使您的浏览器自动刷新。示例 – 模板引擎、CSS 样式表、JavaScript 等。
- LiveReload 具有适用于 Google Chrome、Firefox 和 Safari 等浏览器的浏览器插件。
- Internet Explorer 和 Edge 浏览器没有 LiveReload 浏览器插件。

安慰
4.H2控制台
- 您的应用程序可能还需要一个数据库。
- Spring Boot 为您提供了嵌入式 H2 数据库的一个非常重要的特性,这对于开发目的非常有帮助。
- 当我们在应用构建中添加 H2 数据库依赖时,DevTools 会自动启用一个 H2 控制台。
- 您可以从 Web 浏览器本身访问此控制台。
- 这有助于我们深入了解或概述应用程序使用或处理的数据。
- 要访问此控制台,您只需将 Web 浏览器指向 - http://localhost:8080/h2-console。
要将 Spring Boot DevTools 嵌入到应用程序中,请在构建的项目中添加以下依赖项 -
摇篮
Gradle -> build.gradle
dependencies {
compile("org.springframework.boot:spring-boot-devtools")
}
OR
dependencies {
developmentOnly("org.springframework.boot:spring-boot-devtools")
}
示例 – build.gradle文件
buildscript {
repositories {
jcenter()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:1.3.8.RELEASE")
}
}
apply plugin: 'java'
apply plugin: 'spring-boot'
repositories {
jcenter()
}
dependencies {
compile("org.springframework.boot:spring-boot-starter-web")
developmentOnly("org.springframework.boot:spring-boot-devtools")
testCompile("org.springframework.boot:spring-boot-starter-test")
}
马文
Maven -> pom.xml
org.springframework.boot
spring-boot-devtools
runtime
示例 – pom.xml文件
XML
4.0.0
org.springframework.boot
spring-boot-starter-parent
2.6.3
sia
GFG
0.0.1-SNAPSHOT
GFG
GeeksforGeeks
11
org.springframework.boot
spring-boot-starter-thymeleaf
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-starter
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-devtools
runtime
true
org.springframework.boot
spring-boot-maven-plugin
org.projectlombok
lombok
在添加 DevTools 依赖项时,“devtools”一词会出现在您的项目名称旁边。
示例 1:项目文件
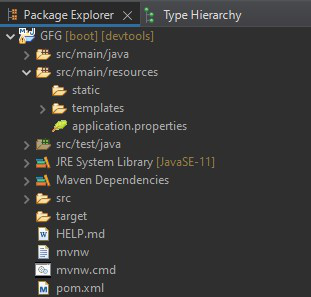
项目——Maven
示例 2:引导仪表板
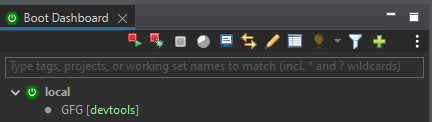
Spring Tool Suite 引导仪表板
Note:
- As the DevTools is neither a plugin nor an IDE-specific functionality, it works equally well in the STS ( Spring Tool Suite ), IntelliJ IDEA, and also Netbeans.
- Also, we now know that the DevTools were built only for development-specific purposes, it is very smart enough that it disables itself when the application is deploying in a production setting.