Bash 脚本 – 如何检查文件是否存在
在本文中,我们将编写一个 bash 脚本来检查文件是否存在。
句法 :
- 测试 [表达式]
- [ 表达 ]
- [[ 表达 ]]
在这里,在表达式中,我们写参数和文件名。让我们看看可以在表达式中使用的一些参数:-
- - f:如果文件作为普通(常规)文件存在,则返回 True。
- -d :如果目录存在则返回 True。
- -e :如果存在任何类型的文件,则返回 True。
- -c :如果字符文件存在则返回 True。
- -r :如果存在可读文件,则返回 True。
- -w :如果存在可写文件,则返回True 。
- -x :如果存在可执行文件,则返回 True。
- -p :如果文件作为管道存在,则返回 True。
- -S :如果文件作为套接字存在,则返回 True。
- -s :如果文件存在且文件大小不为零,则返回 True。
- -L :如果符号链接文件存在则返回True 。
- -g :如果文件存在并且设置了组 ID 标志,则返回 True。
- -G :如果文件存在并且持有与正在处理的相同组 id,则返回 True。
- -k :如果文件存在并且设置了粘性位标志,则返回 True。
现在,还有一些参数可以在两个文件之间进行比较。
- -ef:如果两个文件都存在,则返回True,表示同一个文件。
例子 :
FirstFile -ef SecondFile
- -nt:如果 FirstFile 比 Secondfile 新,则返回 True。
例子 :
FirstFile -nt FileOld
- -ot:如果 FirstFile 早于 SecondFile,则返回 True。
例子:
FirstFile -ot SecondFile
让我们根据语法举一些例子:
- [表达式]:首先,创建一个名为“FirstFile.sh”的文件,并在其上编写以下脚本
#!/bin/bash
# using [ expression ] syntax and in place
# of File.txt you can write your file name
if [ -f "File.txt" ];
then
# if file exist the it will be printed
echo "File is exist"
else
# is it is not exist then it will be printed
echo "File is not exist"
fi
现在使用以下命令保存并运行文件
$ chmod +x ./FirstFile.sh
$ ./FirstFile.sh
输出 :

输出
注意:由于系统中存在“ File.txt ”。所以,它打印了“文件存在”。
- test [表达式]:现在,修改“FirstFile.sh”中的上述脚本如下
#!/bin/bash
# using test expression syntax and in place
# of File2.txt you can write your file name
if test -f "File2.txt" ;
then
# if file exist the it will be printed
echo "File is exist"
else
# is it is not exist then it will be printed
echo "File is not exist"
fi
现在,再次使用以下命令保存并运行文件
$ chmod +x ./FirstFile.sh
$ ./FirstFile.sh
输出 :

输出
注意:由于系统中不存在“ File2.txt ”。所以,它打印了“文件不存在”。
- [[表达式]]:再次修改“FirstFile.sh”中的上述脚本如下
#!/bin/bash
# using [[ expression ]] syntax and in place
# of File3.txt you can write your file name
if test -f "File3.txt" ;
then
# if file exist the it will be printed
echo "File is exist"
else
# is it is not exist then it will be printed
echo "File is not exist"
fi
现在,再次使用以下命令保存并运行文件
$ chmod +x ./FirstFile.sh
$ ./FirstFile.sh
输出 :

输出
注意:由于系统中存在“ File3.txt ”。所以,它打印了“文件存在”。
让我们看一个基于参数的示例:
- 使用 -d 参数:创建一个名为“FirstDir.sh”的文件 ” 并在其中编写以下脚本
!/bin/bash
if [[ -d "GFG_dir" ]] ; # Here GFG_dir
is directory and in place of GFG_dir you can write your Directory name
then
echo "Directory is exist" # If GFG_dir exist then it will be printed
else
echo "Directory is not exist" # If GFG_dir is not exist then it will be printed
fi
现在使用以下命令保存并运行文件
$ chmod +x ./FirstDir.sh
$ ./FirstDir.sh
输出 :
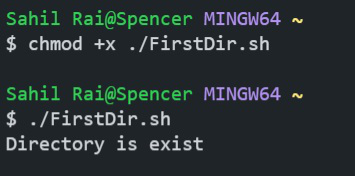
输出
注意:由于系统中存在“ GFG_dir ”。所以,它打印了“Directory is exist”。
同样,您可以使用-f 、 -e 、 -w 、 -r 、 -c等。 (根据他们的用途)代替-d用于检查不同类型文件的存在。
让我们看一个基于两个文件比较的示例:
- 使用-nt参数
创建一个文件名“Comparison_File.sh”并编写以下脚本
#!/bin/bash
# New_file.txt and Old_File.txt are names of two files.
if [[ "New_File.txt" -nt "Old_File.txt" ]] ;
then
# This will be printed if Condition is true
echo "New_File.txt is newer than Old_File.txt"
else
# This will be printed if Condition is False
echo "New_File.txt is not newer than Old_File.txt"
fi
现在使用以下命令保存并运行文件
$ chmod +x ./Comparison_File.sh
$ ./Comparison_File.sh
输出 :
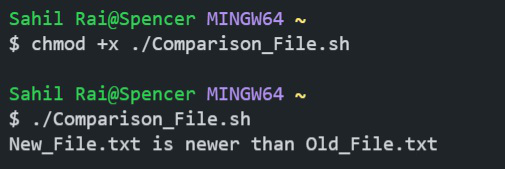
输出
注意:因为这两个文件都存在于系统中,并且“New_File.txt”比“Old_File.txt”更新 “
让我们看一个例子“检查文件是否不存在”:
创建一个名为“Check_Exist.sh”的文件 ” 并在其中编写以下脚本
#!/bin/bash
# using ! before -f parameter to check if
# file does not exist
if [[ ! -f "GFG.txt" ]] ;
then
# This will printed if condition is True
echo "File is not exist"
else
# This will be printed if condition if False
echo "File is exist"
fi
现在使用以下命令保存并运行文件
$ chmod +x ./Check_Exist.sh
$ ./Check_Exist.sh
输出 :

输出
注意:系统中不存在“GFG.txt”。所以,它会打印“文件不存在”
让我们看一个不使用 If-else 条件的示例:
创建一个名为“Geeks_File.sh”的文件并在其中写入以下脚本
#!/bin/bash
# If File exist then first statement will be
# printed and if it is not exist then 2nd
# statement will be printed.
[ -f "GFG_File.txt" ] && echo "File is exist" || echo "File is not exist"
现在使用以下命令保存并运行文件
$ chmod +x ./Geeks_File.sh
$ ./Geeks_File.sh
输出 :
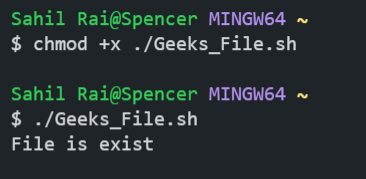
输出
注意:因为系统中存在“GFG_File.txt”。所以,它打印了“文件存在”。