从给定的 N 个索引的非循环图构造一棵素数二叉树
给定具有(N-1) 条边的非循环无向图的1到N个顶点。任务是为这些边分配值,以便构造的树是Prime Tree 。素数树是二叉树的一种,其中图的两个连续边之和是一个素数,每条边也是一个素数。如果可以从给定的图中构造 Prime Tree,则返回边的权重,否则返回 -1。如果可能有多个答案,请打印其中任何一个。
例子:
Input: N = 5, edges = [[1, 2], [3, 5], [3, 1], [4, 2]]
Output: 3, 3, 2, 2
Explanation: Refer to the diagram.
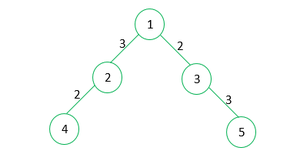
Undirected Graph which is also a Prime Tree
Input: N = 4, edges = [[1, 2], [4, 2], [2, 3]]
Output: -1
方法:这个问题是基于图的概念。之后按照以下步骤在邻接列表的帮助下实现图形:
- 如果任何顶点有两个以上的邻居,那么构造 Prime Tree 是不可能的,返回 -1 。
- 将源src指定给只有一个邻居的那个顶点。
- 如果没有访问过,则开始访问邻居,并为边指定 2 和 3 个值,或者指定两个和为素数的素数。
- 在添加值时,在哈希表的帮助下维护顶点记录。
- 在哈希表的帮助下返回边的值。
下面是上述方法的实现。
Java
// Java code for the above approach
import java.util.*;
class GFG {
// Edge class for Adjacency List
public static class Edge {
int u;
int v;
Edge(int u, int v)
{
this.u = u;
this.v = v;
}
}
// Function to construct a Prime Tree
public static void
constructPrimeTree(int N,
int[][] edges)
{
// ArrayList for adjacency list
@SuppressWarnings("unchecked")
ArrayList[] graph
= new ArrayList[N];
for (int i = 0; i < N; i++) {
graph[i] = new ArrayList<>();
}
// Array which stores source
// and destination
String[] record = new String[N - 1];
// Constructing graph using
// adjacency List
for (int i = 0; i < edges.length;
i++) {
int u = edges[i][0];
int v = edges[i][1];
graph[u - 1].add(new Edge(u - 1,
v - 1));
graph[v - 1].add(new Edge(v - 1,
u - 1));
record[i] = (u - 1) + " "
+ (v - 1);
}
// Selecting source src
int src = 0;
for (int i = 0; i < N; i++) {
// If neighour is more than 2
// then Prime Tree construction
// is not possible
if (graph[i].size() > 2) {
System.out.println(-1);
return;
}
// Appointing src to the vertex
// which has only 1 neighbour
else if (graph[i].size() == 1)
src = i;
}
// Initializing Hash Map which
// keeps records of source and
// destination along with the
// weight of the edge
Map vertices
= new HashMap<>();
int count = 0;
int weight = 2;
// Iterating N-1 times(no. of edges)
while (count < N) {
List ed = graph[src];
int i = 0;
// Finding unvisited neighbour
while (i < ed.size()
&& vertices.containsKey(
src
+ " "
+ ed.get(i).v))
i++;
// Assigning weight to its edge
if (i < ed.size()) {
int nbr = ed.get(i).v;
vertices.put(src + " "
+ nbr,
weight);
vertices.put(nbr + " "
+ src,
weight);
weight = 5 - weight;
src = nbr;
}
count++;
}
// Printing edge weights
for (int i = 0; i < record.length;
i++) {
System.out.print(vertices.get(
record[i])
+ " ");
}
}
// Driver Code
public static void main(String[] args)
{
int N = 5;
int[][] edges
= { { 1, 2 }, { 3, 5 }, { 3, 1 }, { 4, 2 } };
constructPrimeTree(N, edges);
}
}
Javascript
输出
2 2 3 3
时间复杂度: 在)
辅助空间: 在)