Java.awt.image.RescaleOp Java中的类与示例
RescaleOp是Java.awt.image 包中的一个类,它实现了BufferedImageOp和RasterOp接口。此类通过将每个像素的样本值乘以比例因子然后添加偏移量来对源图像中的数据执行逐像素的重新缩放。缩放的样本值被裁剪为目标图像中的最小/最大表示。该类用于图片处理。
- 对于Rasters ,重新缩放对波段进行。缩放常数集的数量可以是一组,在这种情况下,相同的常数将应用于所有波段,或者它必须等于源栅格波段的数量。
- 对于BufferedImages ,重新缩放对颜色进行操作。缩放常数组的数量可以是一组,在这种情况下,相同的常数应用于所有颜色分量。
- 无法重新缩放具有IndexColorModel的图像。
- 如果在构造函数中指定了RenderingHints对象,则在需要进行颜色转换时可以使用颜色渲染提示和抖动提示。
句法:
public class RescaleOp
extends Object
implements BufferedImageOp, RasterOp
构造函数:
- public RescaleOp(float[] scaleFactors, float[] offsets, RenderingHints hints):具有所需 scaleFactors 和偏移量的构造函数。提示可以为空。
- public RescaleOp(float scaleFactor, float offset, RenderingHints hints):具有 singlt scaleFactor 和 offset 的构造函数。提示可以为空。
方法:
- BufferedImage createCompatibleDestImage?(BufferedImage src, ColorModel destCM):此方法创建具有正确大小和带数的归零目标图像。
- WritableRaster createCompatibleDestRaster?(Raster src):在给定源的情况下,此方法创建具有正确大小和波段数的归零目标栅格。
- BufferedImage filter?(BufferedImage src, BufferedImage dst):这个方法重新缩放源BufferedImage。
- WritableRaster filter?(Raster src, WritableRaster dst):此方法重新缩放源 Raster 中的像素数据。
- Rectangle2D getBounds2D?(BufferedImage src):此方法返回重新缩放的目标图像的边界框。
- Rectangle2D getBounds2D?(Raster src):此方法返回重新缩放的目标 Raster 的边界框。
- int getNumFactors?():此方法返回此 RescaleOp 中使用的缩放因子和偏移的数量。
- float[] getOffsets?(float[] offsets):此方法返回给定数组中的偏移量。
- Point2D getPoint2D?(Point2D srcPt, Point2D dstPt):此方法返回给定源中一个点的目标点的位置。
- RenderingHints getRenderingHints?():此方法返回此操作的渲染提示。
- float[] getScaleFactors?(float[] scaleFactors):此方法返回给定数组中的比例因子。
示例 1:在给定的示例中,我们将使用单个比例因子和偏移量设置图片的对比度。下面的代码将亮度降低 25% 并将像素降低 3.6 倍。
Java
import java.awt.image.*;
import java.net.*;
import java.awt.*;
import java.io.*;
import javax.imageio.*;
public class DemoonRescaleop {
public static void main(String[] args) throws Exception
{
// picking the image from the url
URL url
= new URL("https:// media.geeksforgeeks.org"
+ "/wp-content/uploads/geeksforgeeks-9.png");
// Reading the image from url
Image image = ImageIO.read(url);
// Setting up the scaling and
// the offset parameters for processing
RescaleOp rop = new RescaleOp(.75f, 3.6f, null);
// applying the parameters on the image
// by using filter() method, it takes the
// Source and destination objects of buffered reader
// here our destination object is null
BufferedImage bi
= rop.filter((BufferedImage)image, null);
ImageIO.write(bi, "png",
new File("processed.png"));
}
}
Java
import java.awt.image.*;
import java.net.*;
import java.awt.*;
import java.io.*;
import javax.imageio.*;
public class DemoonRescaleop {
public static void main(String[] args) throws Exception
{
// picking the image from the URL
URL url
= new URL("https:// media.geeksforgeeks.org"
+ "/wp-content/uploads/geeksforgeeks-9.png");
// Reading the image from url
Image image = ImageIO.read(url);
// Setting up the scaling and
// the offset parameters for processing
float[] factors = new float[] {
// RGB each value for 1 color
1.45f, 1.45f, 1.45f
};
float[] offsets = new float[] {
0.0f, 150.0f, 0.0f
};
RescaleOp rop
= new RescaleOp(factors, offsets, null);
// applying the parameters on the image
// by using filter() method, it takes the
// Source and destination objects of buffered reader
// here our destination object is null
BufferedImage bi
= rop.filter((BufferedImage)image, null);
ImageIO.write(bi, "png",
new File("processed.png"));
}
}
输入:
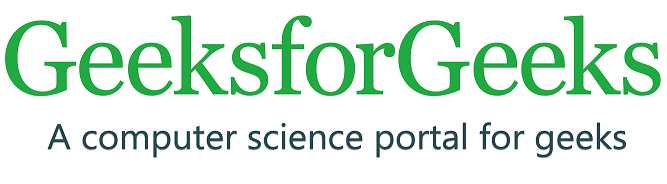
原始图像
输出:
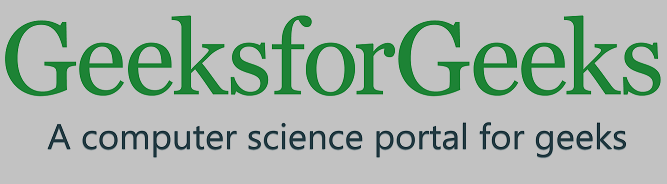
已处理.png
使用的方法filter(BufferedImage src, BufferedImage dst)将源 BufferedImage 重新缩放到目标 BufferedImage 并返回相同的值。在这里,我们没有提到任何目标图像,而是用 null 代替它。在这里,我们为 BufferedImage 对象分配了重新缩放的结果。
示例 2:在给定的示例中,我们将使用比例因子和偏移量数组设置图片的对比度。
每个数组的大小为 3,表示每个像素的红色、绿色和蓝色分量。在下面的代码中,整体亮度增加了 45%,并且所有像素颜色都向绿色移动。 150 的偏移量将每个像素的绿色分量增加了 58.6% (150/256)。请记住,偏移量会添加到颜色值,因此必须是 0 到 255 之间的值,而不是比例因子,它作为百分比。
Java
import java.awt.image.*;
import java.net.*;
import java.awt.*;
import java.io.*;
import javax.imageio.*;
public class DemoonRescaleop {
public static void main(String[] args) throws Exception
{
// picking the image from the URL
URL url
= new URL("https:// media.geeksforgeeks.org"
+ "/wp-content/uploads/geeksforgeeks-9.png");
// Reading the image from url
Image image = ImageIO.read(url);
// Setting up the scaling and
// the offset parameters for processing
float[] factors = new float[] {
// RGB each value for 1 color
1.45f, 1.45f, 1.45f
};
float[] offsets = new float[] {
0.0f, 150.0f, 0.0f
};
RescaleOp rop
= new RescaleOp(factors, offsets, null);
// applying the parameters on the image
// by using filter() method, it takes the
// Source and destination objects of buffered reader
// here our destination object is null
BufferedImage bi
= rop.filter((BufferedImage)image, null);
ImageIO.write(bi, "png",
new File("processed.png"));
}
}
输入:
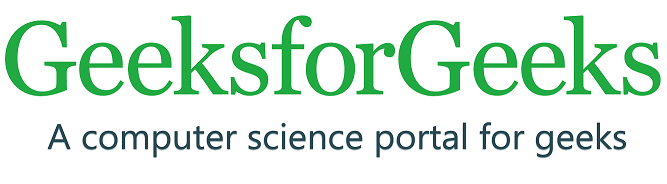
原始图像
输出:
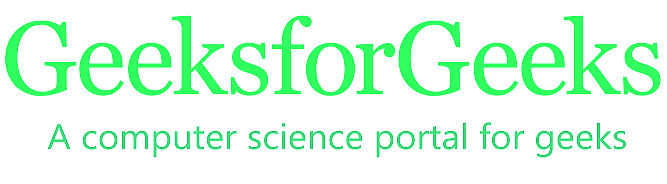
已处理.png
使用的方法filter(BufferedImage src, BufferedImage dst)将源 BufferedImage 重新缩放到目标 BufferedImage 并返回相同的值。在这里,我们没有提到任何目标图像,而是用 null 代替它。在这里,我们为 BufferedImage 对象分配了重新缩放的结果。
参考: https://docs.oracle.com/javase/9/docs/api/ Java/awt/image/RescaleOp.html