在 MATLAB 中创建零数组
MATLAB 通常以矩阵形式、数组和向量形式存储其变量。有时,对于某些特定操作,我们经常需要一个零矩阵(或数组或向量)。我们可以手动或借助 MATLAB 的内置函数创建零矩阵。用于创建零数组或矩阵的内置函数称为zeros()函数。我们通常更喜欢这个内置函数来简化任务,而不是手动创建一个零数组。以下部分包含创建零数组或矩阵的两种方法。
1.手动创建一个零数组
如果我们想创建一个零数组,我们可以简单地使用以下代码手动完成:
例子:
Matlab
% MATLAB Code for create
% an array of zeros
X = [0 0 0 0 0];
disp(X)
Matlab
% MATLAB code to create a
% column vector withe zero's
X = [0; 0; 0; 0; 0]
Matlab
% MATLAB code for create an
% (n x m) dimensional matrix
X = [0 0 0 0; 0 0 0 0; 0 0 0 0]
Matlab
% MATLAB Code for Creating scalar zero
X = zeros
Matlab
% MATLAB code for Creating a (n x n) matrix of zeros
matrix = zeros(3)
Matlab
% MATLAB Code for 3-d matrix of zeros
matrix = zeros(2, 3, 4)
Matlab
% MATLAB Code for clone the size of
% another matrix
matrix = zeros([2 3])
Matlab
% MATLAB code for clone the another
% matrix in different way
A = ones(2, 3);
matrix = zeros(size(A))
Matlab
% Code
A = ones(2, 3);
matrix = zeros(size(A), 'uint32')
Matlab
% MATLAB Code for clone complexity
% of an array
p = [2+2i 13i];
X = zeros('like',p)
Matlab
% MATLAB code for clone Size and
% Data Type from Existing Array
p = uint8([4 5 6; 14 15 16; 12 13 14]);
matrix = zeros(size(p),'like',p)
它基本上是一个大小为 1X5 的行向量以及一个包含 5 个零的数组。
输出:
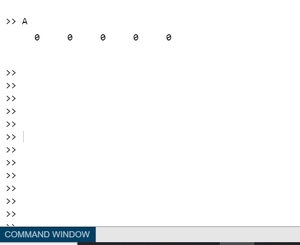
输出截图
手动创建列向量:如果我们想创建一个列向量,我们可以使用以下代码:
MATLAB
% MATLAB code to create a
% column vector withe zero's
X = [0; 0; 0; 0; 0]
它基本上创建了一个大小为 5X1 的列向量。
输出:

输出截图
创建一个 (nxm) 零矩阵:在本节中,我们将创建一个 (nxm) 维矩阵。例如,让 n = 3 和 m = 4。基本上,下面这行代码将生成一个 3 行 4 列的矩阵。
MATLAB
% MATLAB code for create an
% (n x m) dimensional matrix
X = [0 0 0 0; 0 0 0 0; 0 0 0 0]
输出:

输出截图
2. 使用内置函数(zeros()) 创建零数组
我们可以使用zeros()函数创建多个矩阵和数组。我们将在以下部分详细描述它们中的每一个:
创建标量零
Syntax: variable_name = zeros
Return value: In this case, the return value is only scalar zero(‘0’).
例子:
MATLAB
% MATLAB Code for Creating scalar zero
X = zeros
输出:

输出截图
创建一个 (nxn) 零矩阵
syntax: matrix = zeros(n) // Here n is the size of matrix.
Return value: zeros(n) function returns a (n x n) matrix of zeros:
Input Arguments:
- Size of square matrix, specified as an integer value.
- If n is 0 then it returns an empty matrix.
- If n is negative, it also returns an empty matrix.
例子:
MATLAB
% MATLAB code for Creating a (n x n) matrix of zeros
matrix = zeros(3)
此代码返回一个 3 x 3 的零矩阵。
输出:

输出截图
创建一个 (sz1 by sz2 by-…….-szn) 零数组
syntax: matrix = zeros(sz1, sz2,…….,szn)
Return value: This function returns an sz1-by-…-by-szN array of zeros where sz1,…,szN indicate the size of each dimension. For example, zeros(2, 3, 4) returns a 2 X 3 X 4 matrix of zeros.
Input Arguments:
- all the input arguments are specified as an integer value.
- If any of the argument is 0, it returns an empty matrix.
- If any of the argument is negative then it is treated as 0.
- Beyond the second dimension, zeros() ignores trailing dimensions with a size of 1. For example, zeros(4,1,1, 1) produces a 4-by-1 vector of zeros.
例子:
MATLAB
% MATLAB Code for 3-d matrix of zeros
matrix = zeros(2, 3, 4)
上面的代码创建了一个 2×3×4 的零数组。
创建特定大小的矩阵
Syntax: matrix = zeros(sz) // Here sz is the dimension of the matrix in the form [m n].
Return value: It returns an array of zeros where size vector sz defines size(matrix). For example, zeros([2 3]) returns a 2-by-3 matrix.
Input Arguments:
Size of each dimension, specified as a row vector of integer values. The rest of the characteristics of this syntax are as same as the previous one.
例子:
MATLAB
% MATLAB Code for clone the size of
% another matrix
matrix = zeros([2 3])
或者我们也可以克隆另一个矩阵的大小,如下所示:
MATLAB
% MATLAB code for clone the another
% matrix in different way
A = ones(2, 3);
matrix = zeros(size(A))
上面的代码基本上返回一个 2 X 3 的矩阵,每个元素都为零。
输出:

输出截图
创建指定的零数据类型
syntax: matrix = zeros(___,typename) // Here the first argument may be any of the previous types.
Return value: It returns an array of zeros of the specified data type named typename.
Input Arguments:
Data type (class) to create, specified as ‘double’, ‘single’, ‘logical’,’int8′, ‘uint8’, ‘int16’, ‘uint16’, ‘int32’, ‘uint32’, ‘int64’, ‘uint64’, or the name of another class that provides zeros support.
例子:
MATLAB
% Code
A = ones(2, 3);
matrix = zeros(size(A), 'uint32')
此代码创建数据类型为“uint32”的 2×3 矩阵。
输出:

输出截图
使用数组原型创建矩阵
syntax: matrix = zeros(___,’like’,p) // Here the first argument may be any of the previous types.
Return value: It returns an array of zeros like p; i.e, of the same data type (class), sparsity, and complexity (real or complex) as p. The user can specify typename or ‘like’, but not both.
Input Arguments:
Prototype of array to create, specified as an array.
例子
MATLAB
% MATLAB Code for clone complexity
% of an array
p = [2+2i 13i];
X = zeros('like',p)
输出:
例子:
MATLAB
% MATLAB code for clone Size and
% Data Type from Existing Array
p = uint8([4 5 6; 14 15 16; 12 13 14]);
matrix = zeros(size(p),'like',p)
输出: