Spring – REST JSON 响应
REST API 因其在应用程序开发中提供的优势而变得流行。 REST API 的工作方式类似于客户端-服务器架构。客户端发出请求,服务器(REST API)通过提供某种数据进行响应。客户端可以是任何前端框架,如 Angular、React 等,也可以是 Spring 应用程序(内部/外部)本身。数据可以以各种格式发送,例如纯文本、XML、JSON 等。在这些格式中,JSON(JavaScript Object Notation)是在 Web 应用程序之间传输数据的标准。
Pre-requisites required are as follows:
- JSON string can be stored in it own file with the ‘.json‘ extension.
- It has the MIME type of – ‘application/json‘.
JSON
JSON 是 JavaScript Object Notation 的缩写。它是一种遵循 Javascript 对象语法的基于文本的数据格式。它的语法有点像 Javascript 对象字面量量,可以独立于 Javascript 使用。许多编程环境都具有解析和生成 JSON 的能力。它以字符串形式存在,需要转换为原生 Javascript 对象才能通过 Javascript 的可用全局 JSON 方法访问数据。
JSON的结构
{
"id" : 07,
"framework" : "Spring",
"API" : "REST API",
"Response JSON" : true
}
On sending an array of objects, they are wrapped in the square brackets
[
{
"id" : 07,
"name" : "darshan"
},
{
"id" : 08,
"name" : "DARSHAN"
}
]
- Spring 框架的“Starter Web”依赖项为您提供了 Spring MVC 的两个基本特性——(Spring 的 Web 框架)和 RESTful(REST API)方法。
- 要在 Spring 应用程序中包含“Starter Web”,请添加以下依赖项 -
Maven -> pom.xml
org.springframework.boot
spring-boot-starter-web
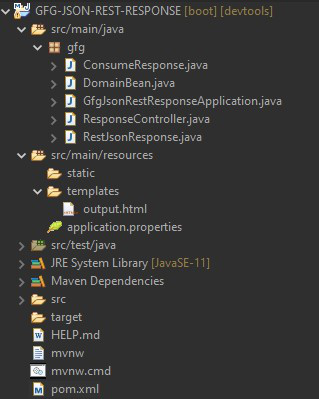
项目结构——Maven
文件: pom.xml(配置)
XML
4.0.0
org.springframework.boot
spring-boot-starter-parent
2.6.3
sia
GFG-JSON-REST-RESPONSE
0.0.1-SNAPSHOT
GFG-JSON-REST-RESPONSE
REST API Response
11
org.springframework.boot
spring-boot-starter-thymeleaf
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-starter
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-devtools
runtime
true
org.springframework.boot
spring-boot-maven-plugin
org.projectlombok
lombok
Java
// Java Program to Illustrate Bootstrapping of an
// Application
package gfg;
// Importing classes
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
// Annotation
@SpringBootApplication
// Main class
public class GfgJsonRestResponseApplication {
// Main driver method
public static void main(String[] args)
{
SpringApplication.run(
GfgJsonRestResponseApplication.class, args);
}
}
Java
// java Program to illustrate DomainBean class
package gfg;
// Importing class
import lombok.Data;
// Annotation
@Data
// Class
public class DomainBean {
String id;
String name;
String data;
}
Java
package gfg;
import java.util.ArrayList;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping(path="/JSON", produces="application/json")
@CrossOrigin(origins="*")
public class RestJsonResponse {
@GetMapping("/data")
public ArrayList get() {
ArrayList arr = new ArrayList<>();
DomainBean userOne = new DomainBean();
userOne.setId("1");
userOne.setName("@geek");
userOne.setData("GeeksforGeeks");
DomainBean userTwo = new DomainBean();
userTwo.setId("2");
userTwo.setName("@drash");
userTwo.setData("Darshan.G.Pawar");
arr.add(userOne);
arr.add(userTwo);
return arr;
}
@GetMapping("/{id}/{name}/{data}")
public ResponseEntity getData(@PathVariable("id") String id,
@PathVariable("name") String name,
@PathVariable("data") String data) {
DomainBean user = new DomainBean();
user.setId(id);
user.setName(name);
user.setData(data);
HttpHeaders headers = new HttpHeaders();
ResponseEntity entity = new ResponseEntity<>(user,headers,HttpStatus.CREATED);
return entity;
}
}
Java
// Java Program to Illustrate Consume REST API response
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
// Class
public class ConsumeResponse {
// Creating an object of ResponseEntity class
RestTemplate rest = new RestTemplate();
public ResponseEntity get()
{
return rest.getForEntity(
"http://localhost:8080/JSON/{id}/{name}/{data}",
DomainBean.class, "007", "geek@drash",
"Darshan.G.Pawar");
}
}
Java
// Java Program to Illustrate Regular Controller
package gfg;
// Importing required classes
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
// Annotation
@Controller
@RequestMapping("/ConsumeResponse")
// Class
public class ResponseController {
@GetMapping("/get") public String get(Model model)
{
// Creating object of ConsumeResponse class
ConsumeResponse data = new ConsumeResponse();
model.addAttribute("response",
data.get().getBody());
model.addAttribute("headers",
data.get().getHeaders());
return "output";
}
}
HTML
GeeksforGeeks
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
HTML
Rest API Response : JSON
{{json.id}}
{{json.name}}
{{json.data}}
CSS
h1, tr{
color : green;
font-size : 25px;
}
文件: GfgJsonRestResponseApplication。 Java (应用程序的引导)
Java
// Java Program to Illustrate Bootstrapping of an
// Application
package gfg;
// Importing classes
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
// Annotation
@SpringBootApplication
// Main class
public class GfgJsonRestResponseApplication {
// Main driver method
public static void main(String[] args)
{
SpringApplication.run(
GfgJsonRestResponseApplication.class, args);
}
}
文件:域豆。 Java (用户属性-要传输的数据)
- Java Beans 需要Getter/Setter 方法。
- 可以使用“Lombok”库的“@Data”注释自动生成它们。
- 依赖关系如下图所示:
Maven -> pom.xml
org.projectlombok
lombok
true
Java
// java Program to illustrate DomainBean class
package gfg;
// Importing class
import lombok.Data;
// Annotation
@Data
// Class
public class DomainBean {
String id;
String name;
String data;
}
文件:RestJsonResponse。 Java ( REST API )
- 此类提供 RESTful 服务。
- 基本注释是——
Annotation | Description |
---|---|
@RestController | Combines @Controller and @ResponseBody |
@RequestMapping | Maps web requests with ‘path’ attribute and response format with ‘produces’ attribute |
@CrossOrigin | Permits cross-origin web requests on the specific handler classes/methods |
@GetMapping | Maps GET request on specific handler method |
@PathVariable | Binds the URI template variables to method parameters |
Java
package gfg;
import java.util.ArrayList;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping(path="/JSON", produces="application/json")
@CrossOrigin(origins="*")
public class RestJsonResponse {
@GetMapping("/data")
public ArrayList get() {
ArrayList arr = new ArrayList<>();
DomainBean userOne = new DomainBean();
userOne.setId("1");
userOne.setName("@geek");
userOne.setData("GeeksforGeeks");
DomainBean userTwo = new DomainBean();
userTwo.setId("2");
userTwo.setName("@drash");
userTwo.setData("Darshan.G.Pawar");
arr.add(userOne);
arr.add(userTwo);
return arr;
}
@GetMapping("/{id}/{name}/{data}")
public ResponseEntity getData(@PathVariable("id") String id,
@PathVariable("name") String name,
@PathVariable("data") String data) {
DomainBean user = new DomainBean();
user.setId(id);
user.setName(name);
user.setData(data);
HttpHeaders headers = new HttpHeaders();
ResponseEntity entity = new ResponseEntity<>(user,headers,HttpStatus.CREATED);
return entity;
}
}
输出:

JSON 对象字面量数组
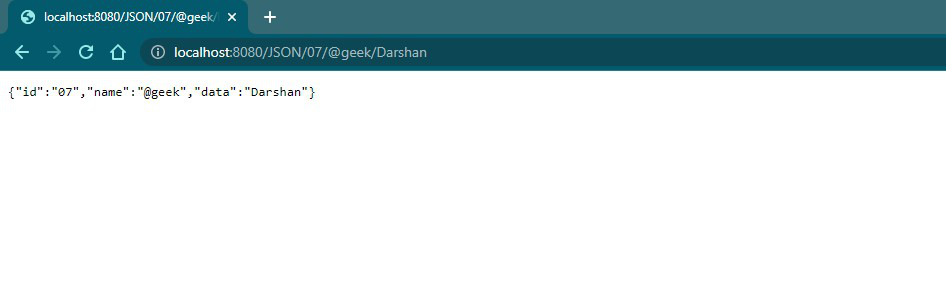
JSON 对象字面量
REST API 的 JSON 响应可以通过以下方式使用:
- Spring 应用程序本身。
- 前端应用程序/框架
A:春季申请
- Spring 提供了“ RestTemplate ”——一种处理 REST 响应的便捷方式。
- 它具有特定于 HTTP 方法的处理程序方法。
文件:消费响应。 Java (使用 REST API 响应)
Java
// Java Program to Illustrate Consume REST API response
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
// Class
public class ConsumeResponse {
// Creating an object of ResponseEntity class
RestTemplate rest = new RestTemplate();
public ResponseEntity get()
{
return rest.getForEntity(
"http://localhost:8080/JSON/{id}/{name}/{data}",
DomainBean.class, "007", "geek@drash",
"Darshan.G.Pawar");
}
}
文件:响应控制器。 Java (常规控制器)
一个普通的 Spring 控制器用于从“RestTemplate”方法中检索响应并返回一个视图。
例子:
Java
// Java Program to Illustrate Regular Controller
package gfg;
// Importing required classes
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
// Annotation
@Controller
@RequestMapping("/ConsumeResponse")
// Class
public class ResponseController {
@GetMapping("/get") public String get(Model model)
{
// Creating object of ConsumeResponse class
ConsumeResponse data = new ConsumeResponse();
model.addAttribute("response",
data.get().getBody());
model.addAttribute("headers",
data.get().getHeaders());
return "output";
}
}
文件: Output.html(API 的输出:Thymeleaf 模板)
HTML
GeeksforGeeks
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
attributeValue will be placed here
输出:
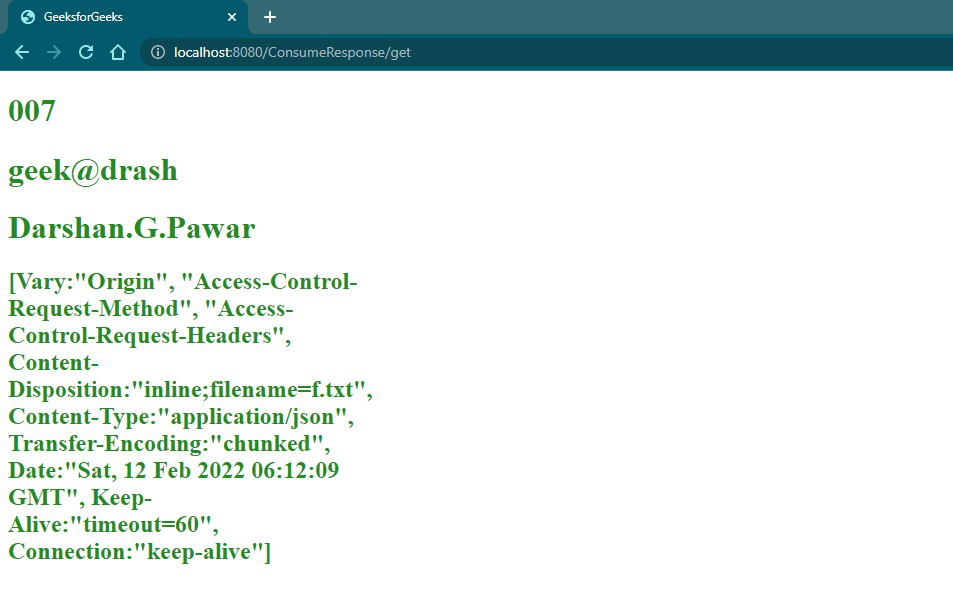
以 JSON 作为内容类型的响应
B:前端应用程序/框架 - Angular
文件: consume-json.component.ts(Angular 组件)
- 此组件从指定的 URL 定位 REST API 检索 JSON 数据。
- 检索到的数据存储在变量中。
import { Component, OnInit, Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-consume-json',
templateUrl: './consume-json.component.html',
styleUrls: ['./consume-json.component.css']
})
export class ConsumeJsonComponent implements OnInit {
restData : any;
constructor(private http:HttpClient) { }
ngOnInit() {
this.http.get('http://localhost:8080/JSON/data')
.subscribe(data => this.restData = data);
}
}
文件: consume-json.component.html(组件视图)
- 通过点 (.) 表示法获取存储的数据。
- 'ngFor' 是一个 Angular 模板指令,用于遍历对象集合。
HTML
Rest API Response : JSON
{{json.id}}
{{json.name}}
{{json.data}}
文件: consume-json.component.css(样式文件)
CSS
h1, tr{
color : green;
font-size : 25px;
}
Add - ' ' in the 'app.component.html' file
输出:
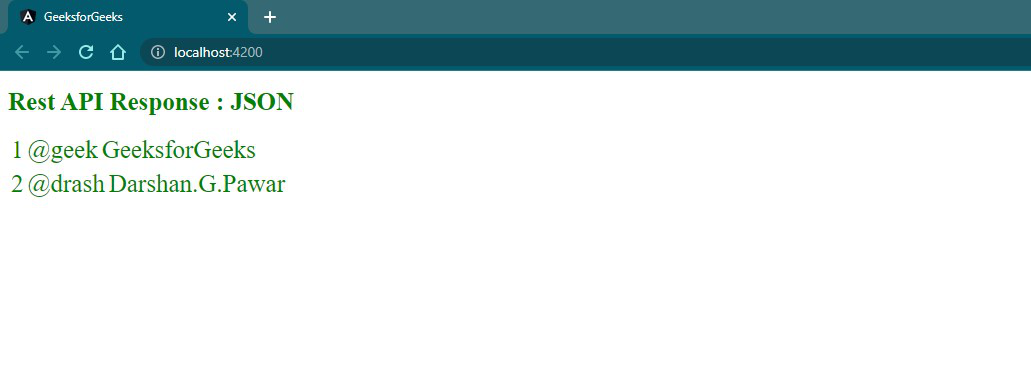
Angular 组件检索到的响应
- 您还可以使用 Jackson API 将对象映射到 JSON 对象字面量。
- 添加以下依赖项:
Maven -> pom.xml
com.fasterxml.jackson.core
jackson-databind
2.5.3
注意:此依赖项还会自动将以下库添加到类路径中:
- 杰克逊注解
- 杰克逊核心