Shell 脚本 - 测试命令
测试命令是用于测试命令有效性的命令。它检查命令/表达式是真还是假。它用于检查文件的类型和与文件相关的权限。如果命令/表达式为真,则测试命令返回 0 作为成功退出状态,如果命令/表达式为假,则返回 1。
句法:
test [expression]
例子:
test "variable1' operator "variable2"
在这里,表达式可以是任何可以由 shell 计算的命令或表达式。并且建议始终将测试变量括在双引号中。
下面是一些可以与test命令一起使用的运算符标志,以及它们的含义:
文件和目录的标志:
- test -e filename:检查文件是否存在。如果文件存在则返回 0,如果文件不存在则返回 1。
- test -d filename:检查文件是否为目录。如果文件是目录则返回 0,如果文件不是目录则返回 1。
- test -f filename:检查文件是否为普通文件。如果文件是常规文件则返回 0,如果文件不是常规文件则返回 1。
- test -s filename:检查文件是否为空。如果文件不为空,则返回 0,如果文件为空,则返回 1。
- test -r filename:检查文件是否可读。如果文件可读则返回 0,如果文件不可读则返回 1。
- test -w filename:检查文件是否可写。如果文件可写,则返回 0,如果文件不可写,则返回 1。
- test -x filename:检查文件是否可执行。如果文件可执行则返回 0,如果文件不可执行则返回 1。
文本字符串的标志
- string1 = string2:检查两个字符串是否相等。如果两个字符串相等则返回 0,如果两个字符串不相等则返回 1。
- string1 != string2:检查两个字符串是否不相等。如果两个字符串不相等则返回 0,如果两个字符串相等则返回 1。
- -n 字符串:检查字符串是否为空。如果字符串为空则返回 1,如果字符串不为空则返回 0。
- -z 字符串:检查字符串是否为空。如果字符串为空则返回 0,如果字符串不为空则返回 1。
用于比较数字的标志
- num1 -eq num2:检查两个数是否相等。如果两个数相等则返回 0,如果两个数不相等则返回 1。
- num1 -ne num2:检查两个数是否不相等。如果两个数不相等则返回 0,如果两个数相等则返回 1。
- num1 -gt num2:检查第一个数字是否大于第二个数字。如果第一个数字大于第二个数字,则返回 0,如果第一个数字不大于第二个数字,则返回 1。
- num1 -ge num2:检查第一个数字是否大于或等于第二个数字。如果第一个数字大于或等于第二个数字,则返回 0,如果第一个数字不大于或等于第二个数字,则返回 1。
- num1 -lt num2:检查第一个数字是否小于第二个数字。如果第一个数字小于第二个数字,则返回 0,如果第一个数字不小于第二个数字,则返回 1。
- num1 -le num2 : 检查第一个数字是否小于或等于第二个数字。如果第一个数小于或等于第二个数,则返回 0,如果第一个数不小于或等于第二个数,则返回 1。
条件标志
- condition1 -a condition2:检查两个条件是否为真。如果两个条件都为真,则返回 0,如果其中一个条件为假,则返回 1。
- condition1 -o condition2:检查两个条件是否为真。如果其中一个条件为真,则返回 0,如果两个条件都为假,则返回 1。
- !expression:检查表达式是否为真。如果表达式为假则返回 0,如果表达式为真则返回 1。
因此,让我们举几个例子来更好地理解测试命令。
1. 数值比较
下面是一个检查两个数字是否相等的示例。
#!/bin/bash
# Example to check if two numbers are equal
# or not
# first number
a=20
# second number
b=20
# using test command to check if numbers
# are equal
if test "$a" -eq "$b"
then
echo "a is equal to b"
else
echo "a is not equal to b"
fi
输出:
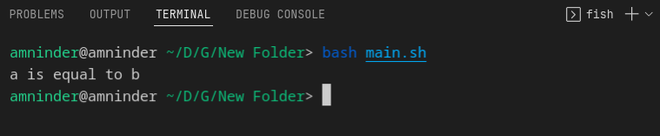
数值比较
2.字符串比较
测试命令也允许我们比较字符串。我们可以检查两个字符串是否相等,一个字符串是否大于另一个,如果一个字符串小于另一个,等等。根据字符串的大小,我们可以进行比较。
3.字符串相等
下面是一个使用 test 命令检查两个字符串是否相等的简单示例
#!/bin/bash
# Example to check if two strings are equal or not
# first string
a="Hello"
b="Hello"
if test "$a" = "$b"
then
echo "a is equal to b"
else
echo "a is not equal to b"
fi
输出:
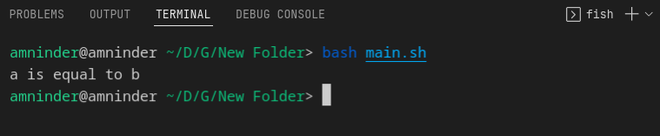
字符串相等比较
4.字符串顺序
字符串顺序基本上意味着检查一个字符串是否大于另一个字符串。下面是检查一个字符串是否大于另一个的示例。
脚本:
#!/bin/bash
# Example to check if one string is greater than
# the other
# first string
a="Hello"
b="Geek"
if test "$a" \> "$b"
then
echo "$a is greater than $b"
else
echo "$a is not greater than $b"
fi
输出:
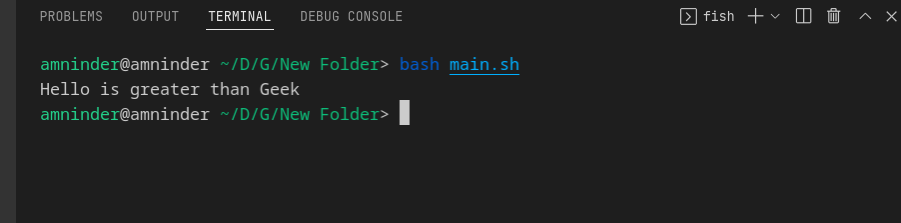
字符串顺序比较
我们必须使用 '\>' 而不是 '>' 因为 '>' 是一个重定向运算符。为了让它工作,我们必须使用'\>'而不是'>'。 '\' 是转义字符。
5.字符串大小
我们还可以使用 test 命令根据字符串的大小来比较字符串。以下是一些执行比较的示例。
#!/bin/bash
# Example to check if string contains some data
# or not
# first string
a="Hello"
# second string
b=""
# using test command on first string to check if
# string is empty or not
# -n will return true if string is not empty
if test -n "$a"
then
echo "String a is not empty"
else
echo "String a is empty"
fi
# -z will return true if string is empty
if test -z "$b"
then
echo "String b is empty"
else
echo "String b is not empty"
fi
输出:
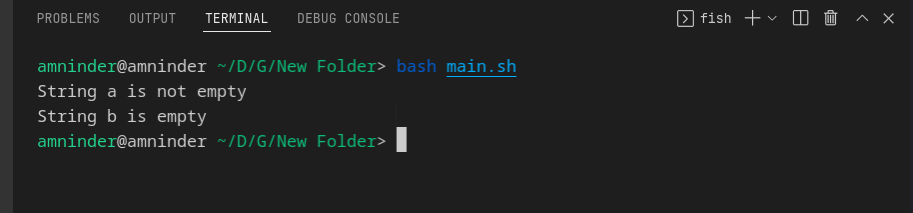
字符串大小比较
6.文件比较
测试命令还可以帮助我们测试 Linux 文件系统上文件和文件夹的状态。下面是一个检查文件是否为空的示例。为此,我们可以使用 -s 标志,如果文件大小大于 0,则返回 true,表示文件不为空,如果文件为空,则返回 false。
#!/bin/bash
# Example to check if file is empty or not
# test command with -s flag to check if file
# is empty or not
# creating new file with the given name
filename="I_LOVE_GEEKSFORGEEKS.txt"
# touch command is used to create empty file
touch $filename
# checking if file is empty or not
if test -s $filename
then
echo "File is not empty"
else
echo "File is empty"
fi
# adding to the newly created text file
echo "I love GeeksforGeeks" >> $filename
# checking again if file is empty or not
if test -s $filename
then
echo "File is not empty now"
else
echo "File is empty"
fi
输出:
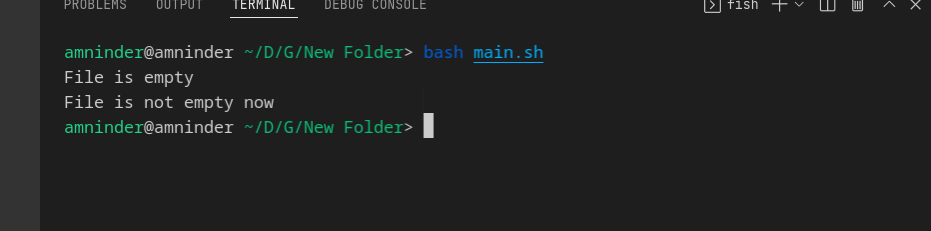
文件比较
例子 :
使用 test 命令检查脚本是否以 root 身份运行的脚本。
# check if the script is run as root or not
if test "$(id -u)" == "0"
then
echo "This script is running as root"
else
echo "This script is not running as root"1>&2
exit 1
fi
输出:
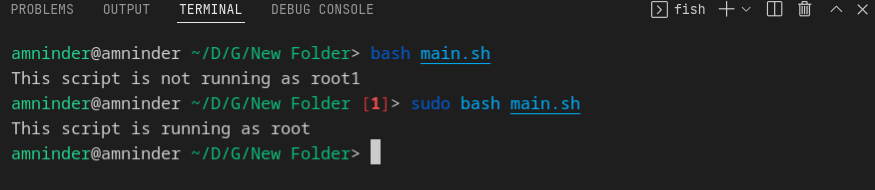
使用 test 命令检查脚本是否以 root 身份运行的脚本
在这里, -id u标志 用于检查正在运行脚本的用户的用户 ID。如果用户 id 不为 0,则表示用户不是 root,脚本将打印 else 语句。这 1>&2 用于将输出重定向到标准错误。 Exit 1 将以状态码 1 退出脚本,即失败,如果我们不使用 exit 1,脚本将以状态码 0 退出,即成功。
注意:这里,红色突出显示的 1 用于表示我们之前运行的脚本以状态码 1 退出。因为它不是以 root 身份运行的。