ReactJS CORS 选项
在 ReactJS 中,跨域资源共享 (CORS) 是指允许您向部署在不同域的服务器发出请求的方法。作为参考,如果前端和后端位于两个不同的域,我们需要 CORS。
在前端级别的 React 应用程序中设置 CORS 请求的方法:
- 在 axios 中:axios总是使用基本 URL 来启动请求,并且浏览器在开始时会自行确认 HTTP OPTIONS 请求。很多时候我们需要传递令牌进行身份验证,而我们正在使用的令牌是由 Bearer 识别的。现在,我们需要为 CORS 传递一些名为Access-Control-Allow-Credentials 的附加标头的主要部分。这是必需的,因为浏览器需要确认服务器是否允许访问资源。
- 在 fetch 中:要在 fetch 中使用 CORS,我们需要使用 mode 选项并将其设置为 cors。
const response = await fetch("https://api.request.com/api_resource", {
method: "GET",
mode: "cors",
headers: {
Authorization: `Bearer: ${token}`,
"Content-Type": "application/json",
},
body: JSON.stringify(data),
});
console.log(response.json());
让我们在 react 中创建一个应用程序来演示上述概念:
创建反应应用程序
第 1 步:使用以下命令创建一个 React 应用程序:
npx create-react-app example
第 2 步:创建项目文件夹(即示例)后,使用以下命令移动到该文件夹:
cd example
第 3 步:这里我们使用 Axios 库来获取 API 数据,我们需要使用根目录中的命令来安装它。
npm install axios
项目结构:它看起来像这样。
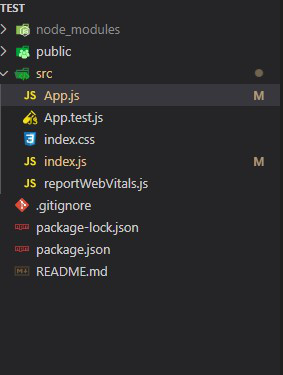
项目结构
示例:演示 ReactJS 中的 CORS 选项。在 index.js 和 App.js 文件中写下以下代码。
index.js
import React from "react";
import ReactDOM from "react-dom";
import "./index.css";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
ReactDOM.render(
,
document.getElementById("root")
);
reportWebVitals();
App.js
import React, { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [isLoading, setLoading] = useState(true);
const [pokemon, setPokemon] = useState();
const baseurl = "https://pokeapi.co/";
useEffect(() => {
axios.get(`${baseurl}api/v2/pokemon/5`).then((response) => {
setPokemon(response.data);
setLoading(false);
});
}, []);
if (isLoading) {
return (
Loading...
);
}
return (
{pokemon.name}
);
}
export default App;
应用程序.js
import React, { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [isLoading, setLoading] = useState(true);
const [pokemon, setPokemon] = useState();
const baseurl = "https://pokeapi.co/";
useEffect(() => {
axios.get(`${baseurl}api/v2/pokemon/5`).then((response) => {
setPokemon(response.data);
setLoading(false);
});
}, []);
if (isLoading) {
return (
Loading...
);
}
return (
{pokemon.name}
);
}
export default App;
运行应用程序的步骤:打开终端并键入以下命令。
npm start
输出:我们将在浏览器屏幕上看到以下输出。
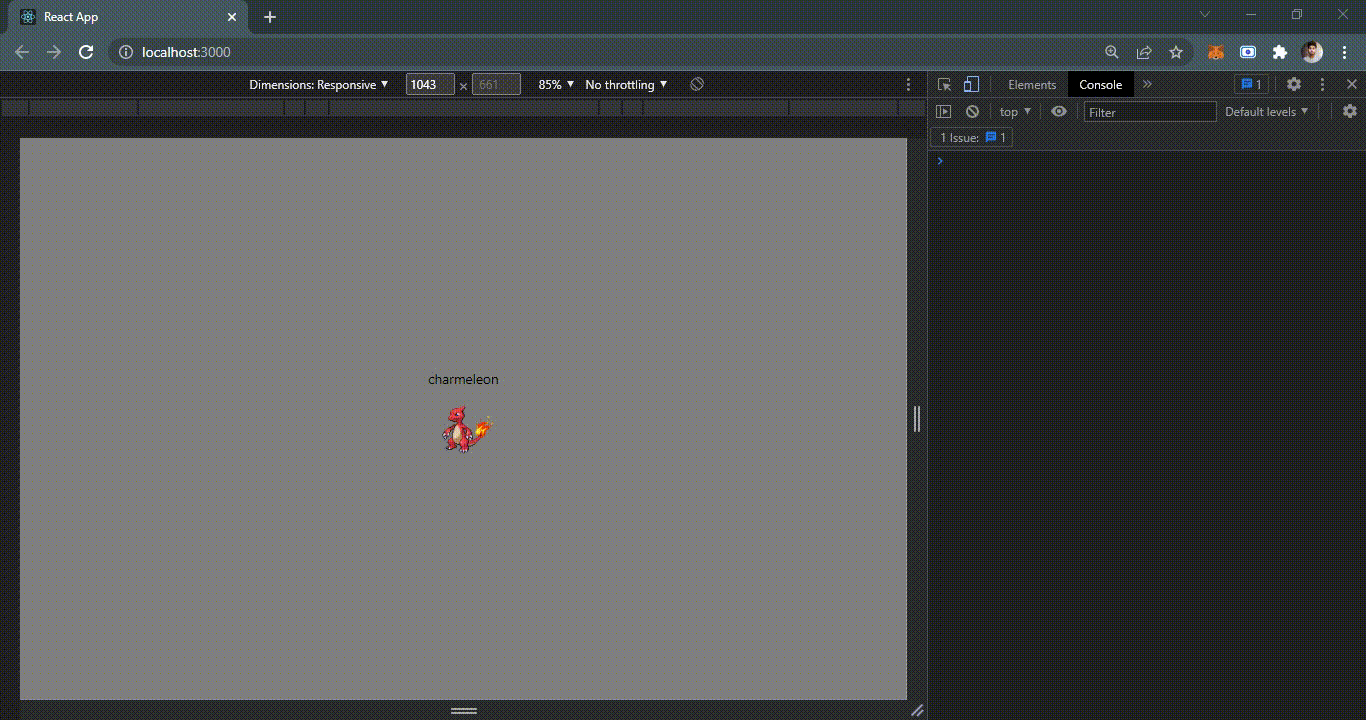
输出
我们可以在控制台中看到没有 CORS 错误。因此,我们在 react 中成功处理了 CORS。