Python中实现多态的方法
在编程中,多态是面向对象编程的一个概念。它允许使用具有不同数据类型、不同类或不同数量输入的输入的单个接口。
示例:在这种情况下,函数len()是多态的,因为它在第一种情况下将字符串作为输入,在第二种情况下将列表作为输入。
Python3
# length of string
x = len('Geeks')
print(x)
# length of list
y = len([1, 2, 3, 4])
print(y)
Python3
class Geeks1:
def code (self, ide):
ide.execute()
class Geeks2:
def execute (self):
print("GeeksForGeeks is the best Platform for learning")
# create object of Geeks2
ide = Geeks2()
# create object of class Geeks1
G1 = Geeks1()
# calling the function by giving ide as the argument.
G1.code(ide)
Python3
class GFG:
def sum(self, a = None, b = None, c = None):
s = 0
if a != None and b != None and c != None:
s = a + b + c
elif a != None and b != None:
s = a + b
else:
s = a
return s
s = GFG()
# sum of 1 integer
print(s.sum(1))
# sum of 2 integers
print(s.sum(3, 5))
# sum of 3 integers
print(s.sum(1, 2, 3))
Python3
class Student:
def __init__(self, m1, m2):
self.m1 = m1
self.m2 = m2
S1 = Student (58, 60)
S2 = Student (60, 58)
# this will generate an error
S3 = S1 + S2
Python3
class Student:
# defining init method for class
def __init__(self, m1, m2):
self.m1 = m1
self.m2 = m2
# overloading the + operator
def __add__(self, other):
m1 = self.m1 + other.m1
m2 = self.m2 + other.m2
s3 = Student(m1, m2)
return s3
s1 = Student(58, 59)
s2 = Student(60, 65)
s3 = s1 + s2
print(s3.m1)
Python3
# parent class
class Programming:
# properties
DataStructures = True
Algorithms = True
# function practice
def practice(self):
print("Practice makes a man perfect")
# function consistency
def consistency(self):
print("Hard work along with consistency can defeat Talent")
# child class
class Python(Programming):
# function
def consistency(self):
print("Hard work along with consistency can defeat Talent.")
Py = Python()
Py.consistency()
Py.practice()
输出:
5
4
在Python中,多态是一种让函数接受行为相似的不同类对象的方法。
在Python中有四种实现多态的方法:
1. Duck Typing : Duck Typing是一个概念,它表示对象的“类型”仅在运行时才需要关注,并且在对其执行任何类型的操作之前不需要明确提及对象的类型那个对象,不像普通类型,其中一个对象的适用性是由它的类型决定的。
在Python中,我们有动态类型的概念,即我们稍后可以提及变量/对象的类型。这个想法是,如果在对象上定义了方法,则不需要类型来调用对象上的现有方法,您可以调用它。
蟒蛇3
class Geeks1:
def code (self, ide):
ide.execute()
class Geeks2:
def execute (self):
print("GeeksForGeeks is the best Platform for learning")
# create object of Geeks2
ide = Geeks2()
# create object of class Geeks1
G1 = Geeks1()
# calling the function by giving ide as the argument.
G1.code(ide)
输出:
GeeksForGeeks is the best Platform for learning
2.方法重载: Python默认不支持方法重载,但我们可以通过修改out方法来实现。
给定单个函数sum(),参数个数可以由你指定。这种以不同方式调用相同方法的过程称为方法重载。
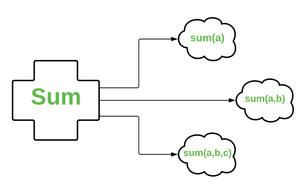
方法重载的概念
蟒蛇3
class GFG:
def sum(self, a = None, b = None, c = None):
s = 0
if a != None and b != None and c != None:
s = a + b + c
elif a != None and b != None:
s = a + b
else:
s = a
return s
s = GFG()
# sum of 1 integer
print(s.sum(1))
# sum of 2 integers
print(s.sum(3, 5))
# sum of 3 integers
print(s.sum(1, 2, 3))
输出:
1
8
6
3. 运算符重载: Python中的运算符重载是单个运算符根据操作数的类(类型)执行多个操作的能力。因此,基本上为运算符定义方法被称为运算符重载。例如:要将 +运算符与自定义对象一起使用,您需要定义一个名为 __add__ 的方法。
我们知道 +运算符用于添加数字并同时连接字符串。这是可能的,因为 +运算符被int class和str class重载。运算符实际上是在各自的类中定义的方法。
因此,如果您想使用 +运算符来添加某个用户定义类的两个对象,那么您必须自己定义该行为并通知Python 。
蟒蛇3
class Student:
def __init__(self, m1, m2):
self.m1 = m1
self.m2 = m2
S1 = Student (58, 60)
S2 = Student (60, 58)
# this will generate an error
S3 = S1 + S2
输出:
TypeError: unsupported operand type(s) for +: 'Student' and 'Student'
所以我们可以看到在用户定义的类中不支持 +运算符。但是我们可以通过为我们的类重载 +运算符来做同样的事情。
蟒蛇3
class Student:
# defining init method for class
def __init__(self, m1, m2):
self.m1 = m1
self.m2 = m2
# overloading the + operator
def __add__(self, other):
m1 = self.m1 + other.m1
m2 = self.m2 + other.m2
s3 = Student(m1, m2)
return s3
s1 = Student(58, 59)
s2 = Student(60, 65)
s3 = s1 + s2
print(s3.m1)
输出:
118
4.方法覆盖:通过使用方法覆盖一个类可以“复制”另一个类,避免重复代码,同时增强或自定义其中的一部分。因此,方法覆盖是继承机制的一部分。
在Python中,方法覆盖通过简单地在与父类中的方法同名的方法中定义子类来实现。
蟒蛇3
# parent class
class Programming:
# properties
DataStructures = True
Algorithms = True
# function practice
def practice(self):
print("Practice makes a man perfect")
# function consistency
def consistency(self):
print("Hard work along with consistency can defeat Talent")
# child class
class Python(Programming):
# function
def consistency(self):
print("Hard work along with consistency can defeat Talent.")
Py = Python()
Py.consistency()
Py.practice()
输出:
Hard work along with consistency can defeat Talent.
Practice makes a man perfect