给定一个仅由小写英文字母组成的字符串S。任务是找到输入给定字符串的最小手指移动次数。当您按下不在键盘上当前按下的键的行中的键时,将考虑移动。
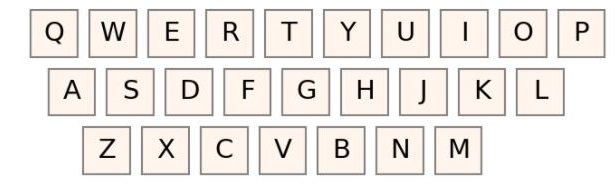
QWERTY 键盘仅由英文字母组成。
例子:
Input: S = “geeksforgeeks”
Output: 7
Explanation:
move 1 >> g
move 2 >> e
move 2 >> e
move 3 >> k
move 3 >> s
move 3 >> f
move 4 >> o
move 4 >> r
move 5 >> g
move 6 >> e
move 6 >> e
move 7 >> k
move 7 >> s
Input: S = “radhamohan”
Output: 6
方法:这可以通过初始设置QWERTY键盘中每个字符的行号来完成。以下是步骤:
- 将每个字符的行存储在数组row[] 中。
- 初始化move = 1 。
- 遍历给定的字符串,并检查字符串中当前字符的行等于前一个字符或没有。
- 如果当前字符不相等,则增加移动,因为我们需要在打印字符时更改当前行。
- 否则检查接下来的字符对。
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function that calculates the moves
// required to print the current string
int numberMoves(string s)
{
// row1 has qwertyuiop, row2 has
// asdfghjkl, and row3 has zxcvbnm
// Store the row number of
// each character
int row[] = { 2, 3, 3, 2, 1, 2, 2,
2, 1, 2, 2, 2, 3, 3,
1, 1, 1, 1, 2, 1, 1,
3, 1, 3, 1, 3 };
// String length
int n = s.length();
// Initialise move to 1
int move = 1;
// Traverse the string
for (int i = 1; i < n; i++) {
// If current row number is
// not equal to previous row
// then increment the moves
if (row[s[i] - 'a']
!= row[s[i - 1] - 'a']) {
move++;
}
}
// Return the moves
return move;
}
// Driver Code
int main()
{
// Given String str
string str = "geeksforgeeks";
// Function Call
cout << numberMoves(str);
return 0;
}
Java
// Java program for the above approach
import java.util.*;
class GFG{
// Function that calculates the moves
// required to print the current String
static int numberMoves(String s)
{
// row1 has qwertyuiop, row2 has
// asdfghjkl, and row3 has zxcvbnm
// Store the row number of
// each character
int row[] = { 2, 3, 3, 2, 1, 2, 2,
2, 1, 2, 2, 2, 3, 3,
1, 1, 1, 1, 2, 1, 1,
3, 1, 3, 1, 3 };
// String length
int n = s.length();
// Initialise move to 1
int move = 1;
// Traverse the String
for (int i = 1; i < n; i++)
{
// If current row number is
// not equal to previous row
// then increment the moves
if (row[s.charAt(i) - 'a'] !=
row[s.charAt(i-1) - 'a'])
{
move++;
}
}
// Return the moves
return move;
}
// Driver Code
public static void main(String[] args)
{
// Given String str
String str = "geeksforgeeks";
// Function Call
System.out.print(numberMoves(str));
}
}
// This code is contributed by sapnasingh4991
Python3
# Python3 program for the above approach
# Function that calculates the moves
# required to prthe current String
def numberMoves(s):
# row1 has qwertyuiop, row2 has
# asdfghjkl, and row3 has zxcvbnm
# Store the row number of
# each character
row = [2, 3, 3, 2, 1, 2,
2, 2, 1, 2, 2, 2, 3,
3, 1, 1, 1, 1, 2, 1,
1, 3, 1, 3, 1, 3];
# String length
n = len(s);
# Initialise move to 1
move = 1;
# Traverse the String
for i in range(1, n):
# If current row number is
# not equal to previous row
# then increment the moves
if(row[ord(s[i]) -
ord('a')] != row[ord(s[i - 1]) -
ord('a')]):
move += 1;
# Return the moves
return move;
# Driver Code
if __name__ == '__main__':
# Given String str
str = "geeksforgeeks";
# Function Call
print(numberMoves(str));
# This code is contributed by Rajput-Ji Add
C#
// C# program for the above approach
using System;
class GFG{
// Function that calculates the moves
// required to print the current String
static int numberMoves(String s)
{
// row1 has qwertyuiop, row2 has
// asdfghjkl, and row3 has zxcvbnm
// Store the row number of
// each character
int []row = { 2, 3, 3, 2, 1, 2, 2,
2, 1, 2, 2, 2, 3, 3,
1, 1, 1, 1, 2, 1, 1,
3, 1, 3, 1, 3 };
// String length
int n = s.Length;
// Initialise move to 1
int move = 1;
// Traverse the String
for (int i = 1; i < n; i++)
{
// If current row number is
// not equal to previous row
// then increment the moves
if (row[s[i] - 'a'] !=
row[s[i - 1] - 'a'])
{
move++;
}
}
// Return the moves
return move;
}
// Driver Code
public static void Main(String[] args)
{
// Given String str
String str = "geeksforgeeks";
// Function Call
Console.Write(numberMoves(str));
}
}
// This code is contributed by sapnasingh4991
输出:
7
时间复杂度: O(N),其中 N 是字符串的长度。
空间复杂度: O(1)
如果您想与行业专家一起参加直播课程,请参阅Geeks Classes Live