一等函数:如果该语言中的函数被视为其他变量,则该语言被称为具有一等函数。因此,函数可以分配给任何其他变量或作为参数传递或可以由另一个函数返回。
JavaScript 将函数视为一等公民。这意味着函数只是一个值,只是另一种类型的对象。
例子:让我们通过一个例子来更多地了解一等函数。
Javascript
const Arithmetics = {
add:(a, b) => {
return `${a} + ${b} = ${a+b}`;
},
subtract:(a, b) => {
return `${a} - ${b} = ${a-b}`
},
multiply:(a, b) => {
return `${a} * ${b} = ${a*b}`
},
division:(a, b) => {
if(b!=0) return `${a} / ${b} = ${a/b}`;
return `Cannot Divide by Zero!!!`;
}
}
console.log(Arithmetics.add(100, 100));
console.log(Arithmetics.subtract(100, 7));
console.log(Arithmetics.multiply(5, 5));
console.log(Arithmetics.division(100, 5));
Javascript
const greet = function(name){
return function(m){
console.log(`Hi!! ${name}, ${m}`);
}
}
const greet_message = greet('ABC');
greet_message("Welcome To GeeksForGeeks")
Javascript
function greet(name){
return `Hi!! ${name} `;
}
function greet_name(greeting,message,name){
console.log(`${greeting(name)} ${message}`);
}
greet_name(greet,'Welcome To GeeksForGeeks','JavaScript');
输出:在上面的程序中,函数作为变量存储在对象中。
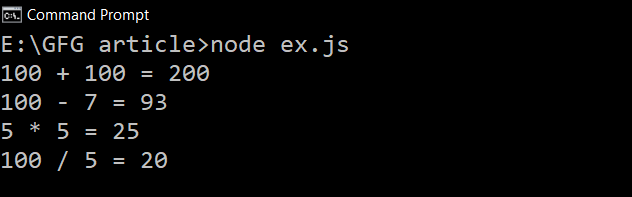
头等舱函数
高阶函数:一个函数接收另一个函数作为参数或者返回一个新的函数或两者被称为高阶函数。高阶函数只有在 First-class 函数 的作用下才有可能。
让我们举一些例子来更好地理解:
示例 1:函数返回另一个函数。
Javascript
const greet = function(name){
return function(m){
console.log(`Hi!! ${name}, ${m}`);
}
}
const greet_message = greet('ABC');
greet_message("Welcome To GeeksForGeeks")
注意:我们也可以像这样调用函数—— greet(‘ABC’)(‘Welcome To GeeksForGeeks’) ,它也会给出相同的输出。
输出:
Hi!! ABC, Welcome To GeeksForGeeks
示例 2:将函数作为参数传递。
Javascript
function greet(name){
return `Hi!! ${name} `;
}
function greet_name(greeting,message,name){
console.log(`${greeting(name)} ${message}`);
}
greet_name(greet,'Welcome To GeeksForGeeks','JavaScript');
注意:我们作为参数传递到另一个函数被调用回调函数的函数。
输出:
Hi!! JavaScript Welcome To GeeksForGeeks
注意: filter()、map()、reduce()、some() 等函数,这些都是高阶函数的例子。
一次函数和高阶函数之间的主要区别: –
First-Order Function | Higher-Order Function |
---|---|
Function are treated as a variable that can be assigned to any other variable or passed as an argument. | Function receives another function as an argument or returns First-order a new function or both. |
The “first-class” concept only has to do with functions in programming languages. | The “higher-order” concept can be applied to functions in general, like functions in the mathematical sense. |
The presence of the First-class function implies the presence of a higher-order function. | The presence of a Higher-order function does not imply the presence of a First-order function. |