JSwing |在Java中创建半透明和形状的窗口
Per – Pixel Translucency 允许程序员控制特定像素相对于其他像素的半透明度。我们将使用这个属性来创建一个形状的窗口。
在本文中,我们将尝试通过创建透明窗口然后绘制面板然后将面板添加到窗口中来在Java中实现形状窗口。
- setBackground(Color c) 方法用于将背景颜色设置为颜色 c。
- 颜色类用于创建所需透明度的颜色。
方法 :
- setBackground(Color c) :将背景颜色设置为颜色 c 的方法
- color(int r, int g, int b, int alpha) :创建具有指定红色、绿色、蓝色和 alpha 值的新颜色。其中 alpha 是半透明的值,其中 255 是不透明的,0 是透明的。
- getRGB(int x, int y) :返回坐标 x, y 的 RGB 值
- setColor(Color c) :设置图形颜色为 c。
例子:
- 示例 1
输入 :
输出:
- 示例 2
输入 :
输出:
- 示例 3:
输入 :
输出 3:
解释:在这里,在输入中我们可以看到背景是透明的,而
GeeksforGeeks 的标志保持原样。通过透明背景我们可以看到下面的窗口。我们已将徽标与其背景分开,并将其绘制在透明窗口上。
下面的程序将说明如何使用每像素半透明度创建一个形状窗口。
Java
// Java Program to implement the shaped window
import javax.swing.*;
import java.awt.*;
import java.awt.image.*;
import java.io.*;
import javax.imageio.*;
class solve extends JFrame {
// main class
public static void main(String[] args)
{
// try block
try {
// create a window
JWindow w = new JWindow();
// set a transparent background of the window
w.setBackground(new Color(0, 0, 0, 0));
// read the image
BufferedImage i = ImageIO.read(new File("f:/gfg.png"));
// create a panel
JPanel p = new JPanel() {
// paint the panel
public void paintComponent(Graphics g)
{
// extract the pixel of the image
for (int ii = 1; ii < i.getHeight(); ii++)
for (int j = 1; j < i.getWidth(); j++) {
// get the color of pixel
Color ty = new Color(i.getRGB(j, ii));
// if the color is more than 78 % white ignore it keep it transparent
if (ty.getRed() > 200 && ty.getGreen() > 200 && ty.getBlue() > 200)
g.setColor(new Color(0, 0, 0, 0));
// else set the color
else
g.setColor(new Color(i.getRGB(j, ii)));
// draw a pixel using a line.
g.drawLine(j, ii, j, ii);
}
}
};
// add panel
w.add(p);
// set the location
w.setLocation(350, 300);
// set the size of the window
w.setSize(900, 900);
// set the visibility of the window
w.setVisible(true);
}
// catch any exception
catch (Exception e) {
// show the error
System.err.println(e.getMessage());
}
}
}
输入 1:
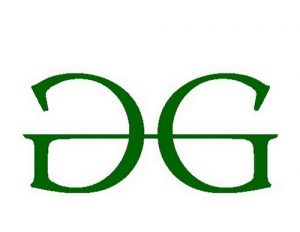
输出 1:
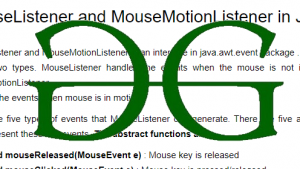
输入 2:
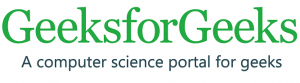
输出 2:
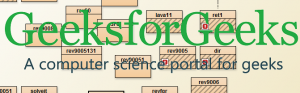
输入 3:
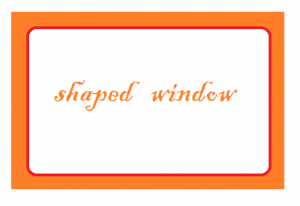
输出 3:
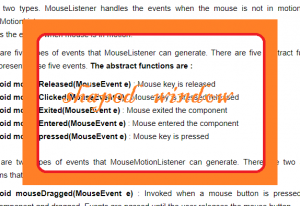
笔记:
我选择了白色作为背景,所以我将它与图像分离并使其透明。由程序员自行选择图像的背景。注意:以上程序可能无法在在线编译器中运行,请使用离线 IDE。
建议使用最新版本的Java来运行上述程序,如果使用旧版本的Java ,用户可能会遇到问题。