在 SQLAlchemy 中选择 NULL 值
在本文中,我们将看到如何使用Python中的 SQLAlchemy 将 NULL 值选择到 PostgreSQL 数据库中。
首先出于演示目的,让我们在 PostgreSQL 中使用 SQLAlchemy 创建一个示例表,如下所示
在 PostgreSQL 中使用 SQLAlchemy 创建表:
- 从 SQLAlchemy 包中导入必要的函数。
- 使用 create_engine()函数与 PostgreSQL 数据库建立连接,如下所示
- 创建一个名为 book_publisher 的表,其中包含 publisher_id、publisher_name 和 publisher_estd 列
- 如图所示,使用 insert() 和 values()函数将记录插入表中。
Syntax: engine = create_engine(dialect+driver://username:password@host:port/database_name)
Parameters:
- dialect – Name of the DBMS. The dialect is the system SQLAlchemy uses to communicate with various types of DBAPIs and databases like PostgreSQL, MySQL, MS SQL, etc.
- driver – Name of the DB API that moves information between SQLAlchemy and the database.
- Username – Name of the admin
- Password – Password of the admin
- host – Name of the host in which the database is hosted
- port – port number through which the database can be accessed
- database_name– Name of the database
创建演示表:
Python3
# import necessary packages
from sqlalchemy import create_engine, MetaData,/
Table, Column, Integer, String
# establish connection
engine = create_engine(
"postgresql+psycopg2://\
postgres:Saibaba97%40@127.0.0.1:5432/test")
# store engine objects
meta = MetaData()
# create a table
book_publisher = Table(
'book_publisher', meta,
Column('publisherId', Integer, primary_key=True),
Column('publisherName', String),
Column('publisherEstd', Integer),
)
# use create_all() function to create a
# table using objects stored in meta.
meta.create_all(engine)
# insert values
statement1 = book_publisher.insert().values(
publisherId=1, publisherName="Oxford", publisherEstd=1900)
statement2 = book_publisher.insert().values(
publisherId=2, publisherName='Stanford', publisherEstd=1910)
statement3 = book_publisher.insert().values(
publisherId=3, publisherName="MIT", publisherEstd=1920)
statement4 = book_publisher.insert().values(
publisherId=4, publisherName="Springer", publisherEstd=1930)
statement5 = book_publisher.insert().values(
publisherId=5, publisherName="Packt", publisherEstd=1940)
statement6 = book_publisher.insert().values(
publisherId=6, publisherName=None, publisherEstd=None)
engine.execute(statement1)
engine.execute(statement2)
engine.execute(statement3)
engine.execute(statement4)
engine.execute(statement5)
engine.execute(statement6)
Python3
# write a conventional SQL query
# with NULL equivalent as None
s = book_publisher.select().where(
book_publisher.c.publisherName == None)
# output get stored in result object
result = engine.execute(s)
# iteratte through the result object
# to get all rows of the output
for row in result:
print(row)
输出:
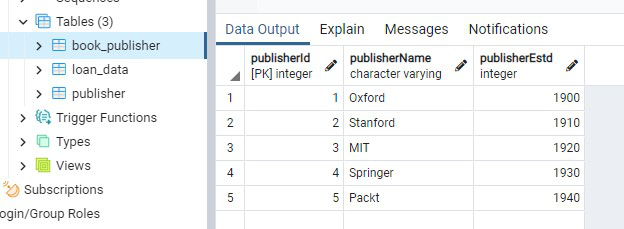
使用 SQLAlchemy 创建的示例表
在 PostgreSQL 中使用 SQLAlchemy 选择 NULL 值
使用 select() 和 where函数,我们可以从数据库中查询数据。 Python中的NULL等效项是None 。
其他事情保持不变,例如传统的 SQL 查询。查询被传递给execute()语句,输出存储在结果对象中,并且可以迭代行以获取所有行
代码:
Python3
# write a conventional SQL query
# with NULL equivalent as None
s = book_publisher.select().where(
book_publisher.c.publisherName == None)
# output get stored in result object
result = engine.execute(s)
# iteratte through the result object
# to get all rows of the output
for row in result:
print(row)
输出:
正如我们从上面显示的输出中知道的那样,第 6 行有 None 值,并且在下面的输出中正确返回
(6, None, None)