在 Pygame 中获取图像的宽度和高度
先决条件: Pygame
为了在Python程序中使用图形,我们使用了一个名为 Pygame 的模块。 Pygame为在Python中开发游戏和图形提供了强大的功能。如今,Pygame 非常流行用于构建简单的 2D 游戏。为了运行使用 Pygame 模块用Python编写的程序,系统必须安装Python和 Pygame 模块。
以下是使用 Pygame 导入图像并获取该图像的高度和宽度所需的示例和步骤。
使用中的图像:链接到图像
尺寸:200×200
方法 1:使用 get_width() 和 get_height() :
函数的名称足以说明它们的用途。
方法
- 导入pygame
- 使用pygame.image.load(“Provide image path here”)创建一个图像对象并将其存储在一个变量中。
- 要获取图像的高度,请使用image.get_height()方法,这里的 image 是存储图像对象的变量。
- 同样,我们使用image.get_width()方法获取图像的宽度,这里的 image 是存储图像对象的变量。
- 打印结果。
例子:
Python3
# import pygame
import pygame
# creating image object
image = pygame.image.load('/home/amninder/Pictures/Wallpapers/download.png')
# get_height method return the height of the surface pixel,
# in our case surface is image
print("Height of image= " + str(image.get_height()))
# get_width method return the width of the surface pixel,
# in our case surface is image
print("Width of image= " + str(image.get_width()))
Python3
import pygame as py
# Initiate pygame and the modules that comes with pygame
py.init()
# setting frame/window/surface with some dimensions
window = py.display.set_mode((300, 300))
# to set title of pygame window
py.display.set_caption("GFG")
# creating image object
image = py.image.load('/home/amninder/Pictures/Wallpapers/download.png')
# to display size of image
print("size of image is (width,height):", image.get_size())
# loop to run window continuously
while True:
window.blit(image, (0, 0))
# loop through the list of Event
for event in py.event.get():
# to end the event/loop
if event.type == py.QUIT:
# it will deactivate the pygame library
py.quit()
quit()
# to display when screen update
py.display.flip()
输出:

方法一
方法 2:使用 get_size() :
此函数能够返回提供给它的图像的尺寸作为元组的参考。
方法
- 导入模块
- 使用display.set_mode()方法创建一个显示对象。
- 使用image.load()方法将图像加载到变量。
- 使用blit()方法在显示表面对象上绘制图像。
- 使用get_size()方法显示图像的宽度和高度,这个 get_size() 方法以元组形式返回宽度和高度。例如。 (200,400)。
- 使用display.flip()来显示内容,即在程序中调用该函数时,在显示表面对象上绘制的任何内容都会显示在窗口中。
例子:
蟒蛇3
import pygame as py
# Initiate pygame and the modules that comes with pygame
py.init()
# setting frame/window/surface with some dimensions
window = py.display.set_mode((300, 300))
# to set title of pygame window
py.display.set_caption("GFG")
# creating image object
image = py.image.load('/home/amninder/Pictures/Wallpapers/download.png')
# to display size of image
print("size of image is (width,height):", image.get_size())
# loop to run window continuously
while True:
window.blit(image, (0, 0))
# loop through the list of Event
for event in py.event.get():
# to end the event/loop
if event.type == py.QUIT:
# it will deactivate the pygame library
py.quit()
quit()
# to display when screen update
py.display.flip()
输出 :
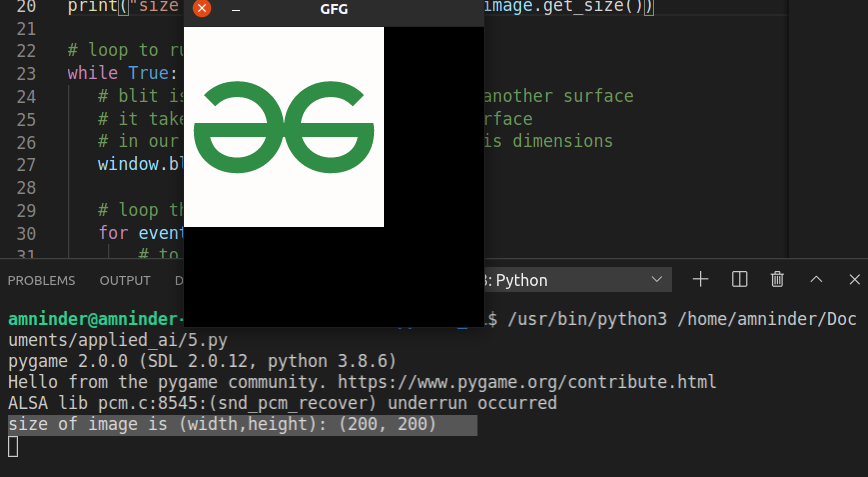
使用方法二