Solidity – 编程 ERC-20 代币
ERC 代币标准 20 代币在遵循以太坊征求意见建议 20 指南的智能合约代币中。我们的程序将具有以下强制性功能:
- 函数totalSupply() 公共视图返回 (uint256);
- 函数balanceOf(address tokenOwner) 公共视图返回(uint);
- 函数津贴(地址令牌所有者,地址支出者)
- 函数转移(地址到,uint 令牌)公共返回(布尔);
- 函数批准(地址支出者,uint 代币)公开回报(布尔);
- 函数transferFrom(address from, address to, uint 令牌) 公共返回 (bool);
以及向外部应用程序发出的两个事件:
- 事件批准(地址索引 tokenOwner,地址索引支出者,uint 令牌);
- 事件传输(地址索引,地址索引,uint令牌);
使用的重要关键字:
- 事件:事件是 Solidity 允许客户端的方式,例如通知您的前端应用程序有关特定事件的发生。
- 视图:视图函数确保它们不会修改状态
- public:可以在合约本身之外访问公共函数
- indexed:最多三个参数可以接收索引的属性,我们可以通过它搜索相应的参数。
步骤 1:表达式 mapping(address => uint256) 定义了一个关联数组,它的键是地址类型,值是 uint256 类型,用于存储余额。
- balances 将持有每个所有者账户的代币余额。
- 允许将包括所有获准从给定账户提款的账户以及每个账户允许的提款金额。
mapping(address => uint256) balances;
mapping(address => mapping (address => uint256)) allowed;
第 2 步:定义代币的总供应量。
步骤3:声明所需的事件并根据指定的签名添加强制功能。
Solidity
// Compatible with version
// of compiler upto 0.6.6
pragma solidity ^0.6.6;
// Creating a Contract
contract GFGToken
{
// Table to map addresses
// to their balance
mapping(address => uint256) balances;
// Mapping owner address to
// those who are allowed to
// use the contract
mapping(address => mapping (
address => uint256)) allowed;
// totalSupply
uint256 _totalSupply = 500;
// owner address
address public owner;
// Triggered whenever
// approve(address _spender, uint256 _value)
// is called.
event Approval(address indexed _owner,
address indexed _spender,
uint256 _value);
// Event triggered when
// tokens are transferred.
event Transfer(address indexed _from,
address indexed _to,
uint256 _value);
// totalSupply function
function totalSupply()
public view returns (
uint256 theTotalSupply)
{
theTotalSupply = _totalSupply;
return theTotalSupply;
}
// balanceOf function
function balanceOf(address _owner)
public view returns (
uint256 balance)
{
return balances[_owner];
}
// function approve
function approve(address _spender,
uint256 _amount)
public returns (bool success)
{
// If the address is allowed
// to spend from this contract
allowed[msg.sender][_spender] = _amount;
// Fire the event "Approval"
// to execute any logic that
// was listening to it
emit Approval(msg.sender,
_spender, _amount);
return true;
}
// transfer function
function transfer(address _to,
uint256 _amount)
public returns (bool success)
{
// transfers the value if
// balance of sender is
// greater than the amount
if (balances[msg.sender] >= _amount)
{
balances[msg.sender] -= _amount;
balances[_to] += _amount;
// Fire a transfer event for
// any logic that is listening
emit Transfer(msg.sender,
_to, _amount);
return true;
}
else
{
return false;
}
}
/* The transferFrom method is used for
a withdraw workflow, allowing
contracts to send tokens on
your behalf, for example to
"deposit" to a contract address
and/or to charge fees in sub-currencies;*/
function transferFrom(address _from,
address _to,
uint256 _amount)
public returns (bool success)
{
if (balances[_from] >= _amount &&
allowed[_from][msg.sender] >=
_amount && _amount > 0 &&
balances[_to] + _amount > balances[_to])
{
balances[_from] -= _amount;
balances[_to] += _amount;
// Fire a Transfer event for
// any logic that is listening
emit Transfer(_from, _to, _amount);
return true;
}
else
{
return false;
}
}
// Check if address is allowed
// to spend on the owner's behalf
function allowance(address _owner,
address _spender)
public view returns (uint256 remaining)
{
return allowed[_owner][_spender];
}
}
输出:
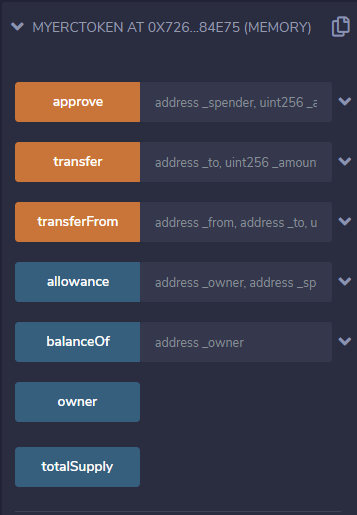
部署后的函数调用