Python SQLite——创建一个新的数据库
在本文中,我们将讨论如何使用Python在 SQLite 中创建数据库。
创建数据库
创建数据库不需要任何特殊权限。用于创建数据库的 sqlite3 命令具有以下基本语法
Syntax: $ sqlite3
数据库名称在 RDBMS 中必须始终是唯一的。
例子:
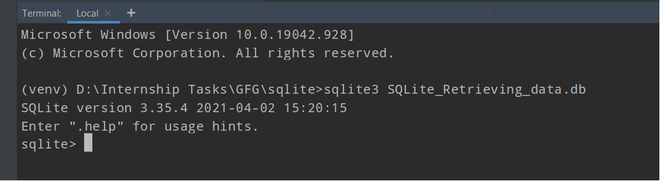
当我们创建一个sqlite数据库时。
同样,我们可以使用 SQlite3 模块在Python创建这个数据库。
Python3
import sqlite3
# filename to form database
file = "Sqlite3.db"
try:
conn = sqlite3.connect(file)
print("Database Sqlite3.db formed.")
except:
print("Database Sqlite3.db not formed.")
Python3
# Importing Sqlite3 Module
import sqlite3
try:
# Making a connection between sqlite3 database and Python Program
sqliteConnection = sqlite3.connect('SQLite_Retrieving_data.db')
# If sqlite3 makes a connection with python program then it will print "Connected to SQLite"
# Otherwise it will show errors
print("Connected to SQLite")
except sqlite3.Error as error:
print("Failed to connect with sqlite3 database", error)
finally:
# Inside Finally Block, If connection is open, we need to close it
if sqliteConnection:
# using close() method, we will close the connection
sqliteConnection.close()
# After closing connection object, we will print "the sqlite connection is closed"
print("the sqlite connection is closed")
输出:
Database Sqlite3.db formed.
连接数据库:
创建一个全新的 SQLite 数据库就像在Python首选库中建立与 sqlite3 模块使用的连接一样简单。要建立连接,您所要做的就是将文件路径传递给 sqlite3 模块中的 connect(...) 方法。如果文件所代表的数据库不存在,则会在此路径下创建。
import sqlite3
connection = sqlite3.connect()
让我们看看有关 connect() 方法的一些描述,
Syntax: sqlite3.connect(database [, timeout , other optional arguments])
使用此 API 会打开与 SQLite 数据库文件的连接。使用“:memory:”建立一个连接到位于 RAM 中而不是硬盘中的数据库。当数据库正确打开时,将返回一个 Connection 对象。
可以通过多个连接访问数据库,其中一个过程是修改数据库。 SQLite 数据库将挂起,直到事务提交。 timeout 参数指定连接在抛出异常之前应等待解锁的时间。超时参数 5.0(五秒)。如果指定的数据库名称不存在,则此调用将创建数据库。
如果我们想在当前目录以外的位置创建数据库数据,我们也可以使用所需的路径来指定文件名。
执行:
1.创建sqlite3数据库与Python程序的连接
sqliteConnection = sqlite3.connect('SQLite_Retrieving_data.db')
2.如果sqlite3与Python程序建立连接,则打印“Connected to SQLite”,否则显示错误
print("Connected to SQLite")
3.如果连接是打开的,我们需要关闭它。关闭代码存在于最终块中。我们将使用 close() 方法关闭连接对象。关闭连接对象后,我们将打印“SQLite connection is closed”
if sqliteConnection:
sqliteConnection.close()
print("the sqlite connection is closed")
蟒蛇3
# Importing Sqlite3 Module
import sqlite3
try:
# Making a connection between sqlite3 database and Python Program
sqliteConnection = sqlite3.connect('SQLite_Retrieving_data.db')
# If sqlite3 makes a connection with python program then it will print "Connected to SQLite"
# Otherwise it will show errors
print("Connected to SQLite")
except sqlite3.Error as error:
print("Failed to connect with sqlite3 database", error)
finally:
# Inside Finally Block, If connection is open, we need to close it
if sqliteConnection:
# using close() method, we will close the connection
sqliteConnection.close()
# After closing connection object, we will print "the sqlite connection is closed"
print("the sqlite connection is closed")
输出:

以上Python程序的输出