Java中的 PatternSyntaxException 类
Java使用Java.util.regex API 与正则表达式进行模式匹配。为了指示正则表达式模式中的任何语法错误, Java提供了一个名为PatternSyntaxException的类。
Java中的 PatternSyntaxException 类:
在编写正则表达式时,为了识别和抛出模式中的任何语法错误,使用PatternSyntaxException类。这是Java 1.4 版本的Java .util.regex包中可用的未经检查的异常。
句法:
public class PatternSyntaxException extends IllegalArgumentException implements Serializable
PatternSyntaxException 类的层次结构:
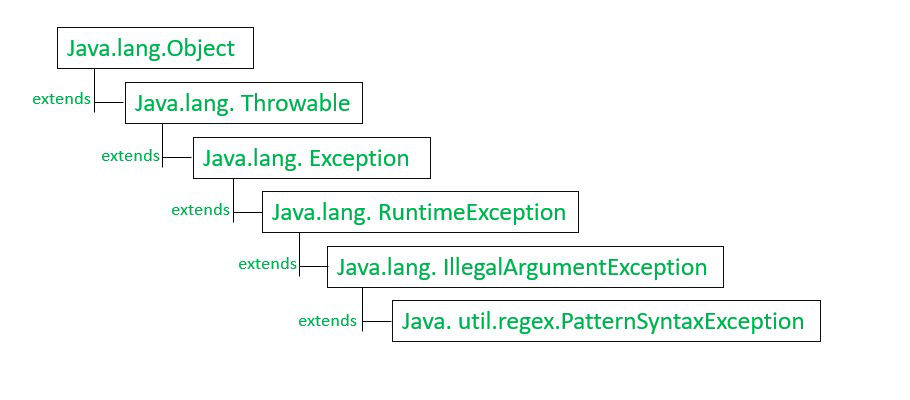
PatternSyntaxException 类的层次结构
PatternSyntaxException 类的构造函数:
构造 PatternSyntaxException 类的新实例。
句法:
PatternSyntaxException(String desc, String regex, int index)
参数:
- desc:错误的描述。
- 正则表达式: 不正确的模式。
- index:正则表达式模式中错误的近似索引,如果索引未知,则为 -1。
PatternSyntaxException 类中的方法
S. No. | Method Name | Modifier | Description |
---|---|---|---|
1 | getIndex() | int | It returns the approximate index of the error in the pattern, or -1 if the index is not known. |
2 | getDescription() | String | It returns the description of the error in the pattern. |
3 | getMessage() | String | It returns the description of the syntax error in the pattern and its index, the incorrect regular-expression pattern, and a visual indication of the error-index within the pattern in a full detailed message. |
4 | getPattern() | String | It returns the regular-expression pattern which is incorrect. |
从Java.lang.Throwable 类继承的方法
由于 PatternSyntaxException 类是从 Throwable 类扩展而来的,所以下面的方法都继承自它。S. No. Method Name Modifier Description 1. addSuppressed(Throwable exception) void This method is thread-safe which appends the specified exception to the exceptions that were suppressed in order to deliver this exception. 2. getCause() Throwable It returns the cause of this throwable or null if the cause is nonexistent or unknown. 3. getStackTrace() StackTraceElement[] It provides programmatic access to the stack trace information printed by printStackTrace() method. 4. initCause(Throwable cause) Throwable This method initializes the cause of this throwable to the specified value. 5. printStackTrace(PrintStream s) void Method prints this throwable and its backtrace to the specified print stream. 6. setStackTrace(StackTraceElement[] stackTrace) void This method is designed for use by RPC frameworks and other advanced systems which allows the client to override the default stack trace. It sets the stack trace elements that will be returned by getStackTrace() and printed by printStackTrace() and related methods. 7. fillInStackTrace() Throwable This method records within this Throwable object information about the current state of the stack frames for the current thread and fills in the execution stack trace. 8. getLocalizedMessage() String This method creates a localized description of this throwable. 9. getSuppressed() Throwable[] It returns an array containing all of the exceptions that were suppressed in order to deliver this exception. 10. printStackTrace() void It prints this throwable and its backtrace to the standard error stream. 11. printStackTrace(PrintWriter s) void It prints this throwable and its backtrace to the specified print writer. 12. toString() String It returns a short description of this throwable with the name of the class of this object, “: ” (a colon and a space), and the result of invoking this object’s getLocalizedMessage() method.
从Java.lang.Object 类继承的方法
由于 PatternSyntaxException 类是从 Object 类扩展而来的,所以下面的方法都继承自它。S. No. Method Name Modifier Description 1. equals(Object obj) boolean Indicates whether some other object is “equal to” this one or not. 2. wait() void It causes the current thread to wait until another thread invokes the notify() method or the notifyAll() method for this object. 3. wait(long timeout) void It causes the current thread to wait until either another thread invokes the notify() method or the notifyAll() method for this object, or the amount of time that is specified in the parameter has elapsed. 4. notifyAll() void It wakes up all the threads that are waiting on this object’s monitor. 5. hashCode() int It returns a hash code value for this object. 6. clone() protected Object It creates and returns a copy of this object. 7. finalize() protected void It is called by the garbage collector on an object when garbage collection determines that there are no more references to the object. We can perform cleanup actions before the object is irrevocably discarded. 8. wait(long timeout, int nanos) void It causes the current thread to wait until another thread invokes the notify() method or the notifyAll() method for this object, or some other thread interrupts the current thread, or a certain amount of real time that is specified has elapsed. 9. notify() void It wakes up a single thread that is waiting on this object’s monitor. 10. getClass() Class> It returns the runtime class of this Object. The returned Class object is the object that is locked by static synchronized methods of the represented class.
示例 1:创建一个类“Demo1 ”,使用正则表达式将给定消息中的所有非字母数字字符替换为空格。
Java
// Java program to demonstrate the working
// of PatternSyntaxException class methods
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
public class Demo1 {
private static String REGEX = "?[^a-zA-Z0-9]";
private static String MSG
= "Learn/ java? in GeeksforGeeks!!";
private static String REPLACE = " ";
public static void main(String[] args)
{
try {
// Get Pattern class object to compile the
// regular expression.
Pattern pattern = Pattern.compile(REGEX);
// Get a matcher object
Matcher matcher = pattern.matcher(MSG);
// Using matcher object, replace the string
MSG = matcher.replaceAll(REPLACE);
// catch block to handle PatternSyntaxException.
}
catch (PatternSyntaxException pse) {
System.out.println("PatternSyntaxException: ");
System.out.println("Description: "
+ pse.getDescription());
System.out.println("Index: " + pse.getIndex());
System.out.println("Message: "
+ pse.getMessage());
System.out.println("Pattern: "
+ pse.getPattern());
System.exit(0);
}
System.out.println("Output: " + MSG);
}
}
Java
// Java program to demonstrate the working
// of PatternSyntaxException class methods
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
public class Demo2 {
private static String REGEX = "[Geek";
private static String MSG
= "Hi Geek, Welcome to GeeksforGeeks!!";
public static void main(String[] args)
{
Pattern pattern = null;
Matcher matcher = null;
try {
pattern = Pattern.compile(REGEX);
matcher = pattern.matcher(MSG);
}
catch (PatternSyntaxException pse) {
System.out.println("PatternSyntaxException: ");
System.out.println("Pattern: "
+ pse.getPattern());
System.out.println("Description: "
+ pse.getDescription());
System.out.println("Message: "
+ pse.getMessage());
System.out.println("Index: " + pse.getIndex());
System.exit(0);
}
boolean found = false;
while (matcher.find()) {
System.out.println("Found the text at "
+ matcher.start());
found = true;
}
if (!found) {
System.out.println("No match found!");
}
}
}
PatternSyntaxException:
Description: Dangling meta character '?'
Index: 0
Message: Dangling meta character '?' near index 0
?[^a-zA-Z0-9]
^
Pattern: ?[^a-zA-Z0-9]
- 在此示例中,我们尝试将所有非字母数字字符替换为空格。
- 但在正则表达式中,有 ' ? ' 这是导致错误的原因。每当我们使用像 ' + ',' * ',' 这样的元字符时? ',我们需要更加小心,应该使用转义字符。
- 由于它导致了错误,我们可以在输出中看到带有索引和正则表达式模式的异常详细信息。
示例 2:创建一个类“ Demo2 ”以在给定消息中查找匹配模式。
Java
// Java program to demonstrate the working
// of PatternSyntaxException class methods
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
public class Demo2 {
private static String REGEX = "[Geek";
private static String MSG
= "Hi Geek, Welcome to GeeksforGeeks!!";
public static void main(String[] args)
{
Pattern pattern = null;
Matcher matcher = null;
try {
pattern = Pattern.compile(REGEX);
matcher = pattern.matcher(MSG);
}
catch (PatternSyntaxException pse) {
System.out.println("PatternSyntaxException: ");
System.out.println("Pattern: "
+ pse.getPattern());
System.out.println("Description: "
+ pse.getDescription());
System.out.println("Message: "
+ pse.getMessage());
System.out.println("Index: " + pse.getIndex());
System.exit(0);
}
boolean found = false;
while (matcher.find()) {
System.out.println("Found the text at "
+ matcher.start());
found = true;
}
if (!found) {
System.out.println("No match found!");
}
}
}
PatternSyntaxException:
Pattern: [Geek
Description: Unclosed character class
Message: Unclosed character class near index 4
[Geek
^
Index: 4
Note:
- This is a common mistake in which the programmer has forgotten the closing parenthesis in the regular expression.
- So, the output is showing the complete details with the error message and the index of it.