使用 Mobilenet 进行图像识别
介绍:
图像识别在医学疾病分析等许多领域发挥着重要作用。在本文中,我们将主要关注如何识别给定的图像,显示的内容。我们假设对 Tensorflow、Keras、 Python和 MachineLearning 有一定的了解
此外,我们将使用 Colaboratory 作为我们的笔记本来运行Python代码并训练我们的模型。
描述:
我们的目标是使用机器学习来识别给定的图像。我们假设我们的 Tensorflow 中已经有一个预训练的模型,我们将用它来识别图像。因此,我们将使用 Tensorflow 的 Keras 导入架构,这将帮助我们识别图像并使用坐标和索引以更好的方式预测图像,我们将使用 NumPy 作为工具。
脚步:
1)首先,我们必须打开 Colaboratory 并将我们的 Gmail 帐户链接到它。
现在首先我们将导入笔记本中的所有需求,然后加载我们要识别的图像。
import tensorflow as tf
import numpy as np
from tensorflow.keras.preprocessing import image
import matplotlib.pyplot as plt
from tensorflow.keras.applications import imagenet_utils
2)要在笔记本中加载图像,我们必须首先将图像文件添加到文件夹中,然后将其路径传递给任何变量(现在设为 FileName),如下所示:
FileName = ‘Path_to_img’
img = image.load_img(filename,target_size=(224,224))
plt.imshow(img)
现在要显示这个图像,我们必须将它加载到我们的 TensorFlow 模型中,这可以使用tensorflow.keras.preprocessing 中的图像库来完成。该库用于在我们的模型中加载图像,然后我们可以将其打印以显示图像,如下所示:
In the above method image is displayed in RGB and pixels format by default. So we will be using matplotlib.pyplot to plot our image using coordinates and to get a visualised form of our image in a better way.
The method in lib of matplotlib.pyplot is imshow( image_Variable ) which is used to display image clearly.Hence,
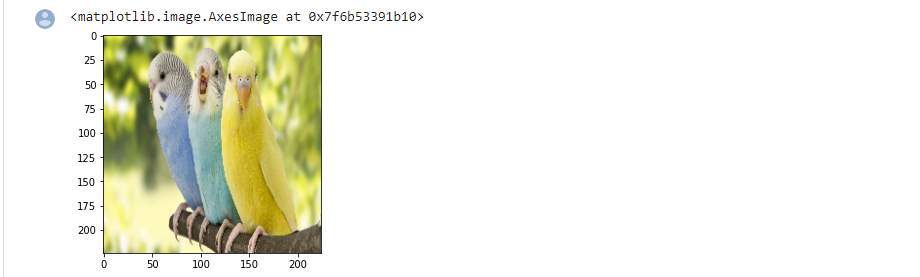
输出图像
因此,我们加载了我们将要识别的图像的一些特征。
3)现在我们将使用一个预训练的模型来测试我们对图像的预测。
由于tensorflow.keras.applications 中有大量模型集合,所以我们可以使用任何模型来预测图像。这里我们将使用mobilenet_v2模型。
Mobilenet_v2是Mobilenet系列的第二个版本模型(虽然还有很多其他版本)。这些模型利用 CNN(卷积神经网络)来预测图像的特征,例如当前物体的形状是什么以及与之匹配的是什么。
How CNN works?
Since the images can be seen as a matrix of pixels and each pixel describes some of features of the image, so these technologies uses filters to filter out certain set of pixels in the images and results in the formation of output predictions about images.
CNN uses lot of pre-defined and stored filters and does a convolution (X) of that filter with the pixels matrix of the image. This results in filtering the image’s objects and comparing them with a large set of pre-defined objects to identify a match between them. Hence in this way these models are able to predict the image.
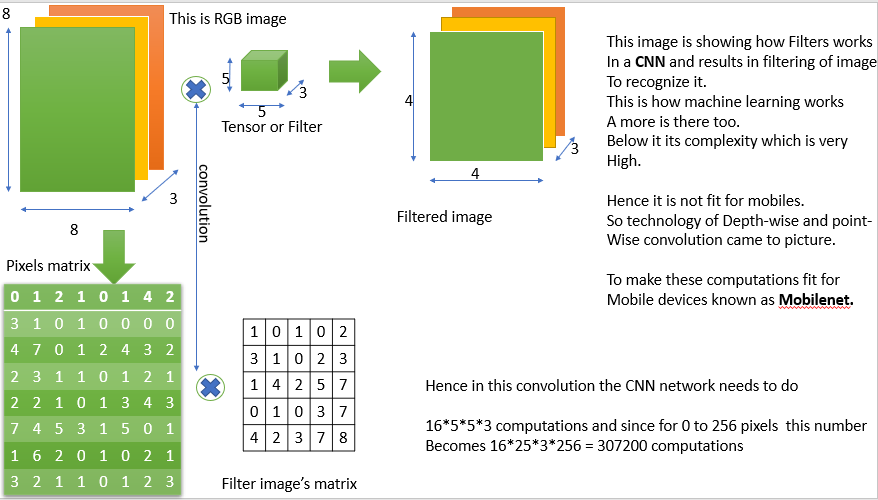
CNN’s working.
But these technologies requires a high GPU to increase the comparison rate between millions of data which cannot be provided by any mobile device.
Hence, here comes in action what is known as MobileNet.
Mobilenet 是一个模型,它执行与 CNN 相同的卷积来过滤图像,但方式与之前的 CNN 所做的不同。它使用了深度卷积和点卷积的思想,这与普通 CNN 所做的普通卷积不同。这提高了 CNN 预测图像的效率,因此它们也可以在移动系统中竞争。由于这些卷积方式大大减少了比较和识别时间,因此它在很短的时间内提供了更好的响应,因此我们将它们用作我们的图像识别模型。
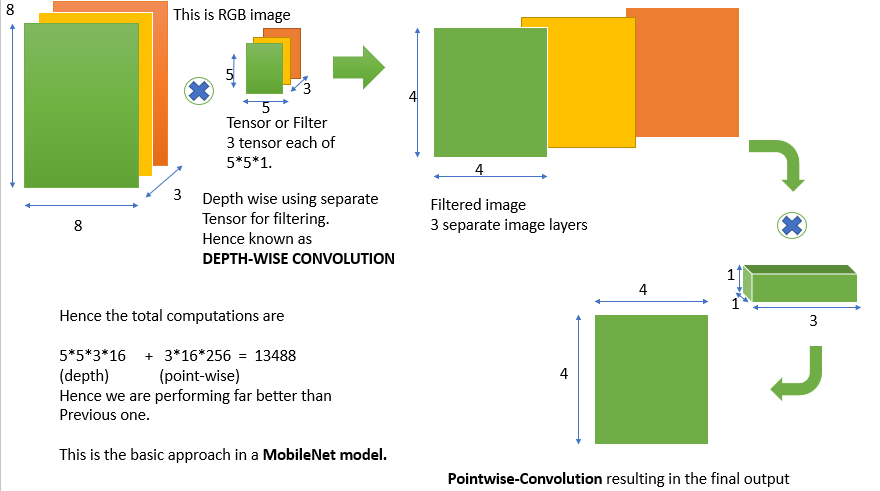
对之前的想法的增强
因此,要将此模型导入模型中的变量,我们将代码编写为:
model = tf.keras.applications.mobilenet_v2.MobileNetV2()
我们现在将以数组的形式将加载的图像提供给它,因此要将图像转换为数组,我们将使用图像库(上面讨论过),其方法名为img_to_array()如下:
现在我们使用我们训练数据集的preprocess_input()和predict()方法来预测图像细节。
4)现在既然做出了预测,所以为了显示它们,我们必须对它们进行解码。为了解码它们,我们将使用imagenet_utils 。该库用于解码数组图像并对其进行许多更改。
一种名为decode_predictions ( ) 的方法用于解码对人类可读格式所做的预测。
results = imagenet_utils.decode_predictions(predictions)# decode_predictions() method is used.
print(results)
因此,预测的整体代码如下所示:
Python3
import tensorflow as tf
import numpy as np
from tensorflow.keras.preprocessing import image
import matplotlib.pyplot as plt
from tensorflow.keras.applications import imagenet_utils
from IPython.display import Image
# importing image
filename = '/content/birds.jpg'
#displaying images
Image(filename,width=224,height=224)
img = image.load_img(filename,target_size=(224,224))
print(img)
plt.imshow(img)
#initializing the model to predict the image details using predefined models.
model = tf.keras.applications.mobilenet_v2.MobileNetV2()
resizedimg = image.img_to_array(img)
finalimg = np.expand_dims(resizedimg,axis=0)
finalimg = tf.keras.applications.mobilenet_v2.preprocess_input(finalimg)
finalimg.shape
predictions = model.predict(finalimg)
# To predict and decode the image details
results = imagenet_utils.decode_predictions(predictions)
print(results)
输出:
我们可以看到输出包含图像中鸟的名称和它所在的像素。
[[('n01558993', 'robin', 0.8600541), ('n04604644', 'worm_fence', 0.005403478),
('n01806567', 'quail', 0.005208329), ('n01530575', 'brambling', 0.00316776),
('n01824575', 'coucal', 0.001805085)]]
因此,我们使用机器学习模型和Python来识别带有笔记本的鸟的图像。