Rust – 泛型函数
在 Rust 中,泛型函数非常有用。泛型使代码更灵活,并为函数的调用者提供更多函数。它可以防止代码重复,因为不需要定义不同类型的不同功能。泛型在函数签名中指定,我们实际上指定参数的数据类型和返回类型。
让我们看一个普通函数的例子:
fn sampleFunction1(varX:i32)->i32{
// this function accepts integer and return an integer
……………….
………………..
}
fn sampleFunction2(varX:f64)->f64{
……
……
// accepts float and return float
// same functionality of above function
}
以上是两个功能相同的函数,但由于参数类型和返回类型不同,定义了两个函数。但是泛型函数将帮助我们定义一次函数并将其与多种类型一起使用。
句法:
fn functionName(variable_name1 : T, variable_name2 : T ,...) -> T {
......
......
}
where T represents the generic type. variable_name1,
variable_name2 are parameters of type generic.
The function will return a value of the same type T.
Let's look at the coding part of using a generic function.
让我们看另一个例子,在 Rust 中编写一个程序来将两个相同类型的数字相加。
示例 1:
Rust
// Rust code for addition
fn main() {
println!("Addition between 10 & 20 is {}", sumGeneric(10,20));//30
println!("Addition between 10.1 & 20.3 is {}", sumGeneric(10.1,20.3));//30.4
}
// generic function that add two numbers
use std::ops::Add;
fn sumGeneric + Copy> (a: T, b: T) -> T {
return a + b;
}
Rust
// Rust program to find the largest number among 3 numbers.
fn main() {
println!("Greatest among 1, 2, 3 is {}",greater(1,2,3));
println!("Greatest among 1.2, 7.2, 3.0 is {}",greater(1.2,7.2,3.0));
println!("Greatest among 10, 20, 30 is {}",greater(10,20,30));
}
fn greater(a:T,b:T,c:T)->T{
if a>b && a>c{
return a;
}
else if b>a && b>c{
return b;
}
else{
return c;
}
}
输出:
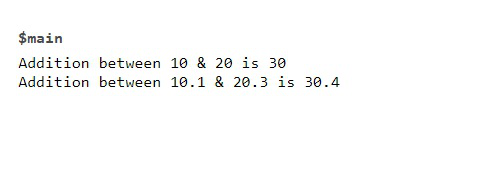
如果我们没有使用泛型函数,那么我们需要编写两个不同的函数,一个函数接受整数并返回一个整数,另一个函数接受浮点数并返回浮点结果。所以这里泛型函数可以帮助我们避免代码冗余。
让我们看一下泛型函数的另一个例子,以便更好地理解。
示例 2:
锈
// Rust program to find the largest number among 3 numbers.
fn main() {
println!("Greatest among 1, 2, 3 is {}",greater(1,2,3));
println!("Greatest among 1.2, 7.2, 3.0 is {}",greater(1.2,7.2,3.0));
println!("Greatest among 10, 20, 30 is {}",greater(10,20,30));
}
fn greater(a:T,b:T,c:T)->T{
if a>b && a>c{
return a;
}
else if b>a && b>c{
return b;
}
else{
return c;
}
}
输出:
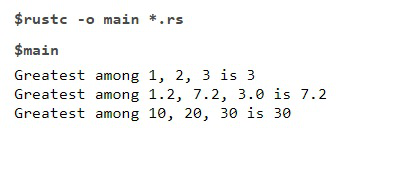
在上面的代码中,在泛型函数内部,我们使用 PartialOrd 表示允许比较函数中的参数的有序特征。使用泛型函数的好处是它有助于防止代码重复并使代码看起来更有条理和简洁。