使用 Express.js 的 BMI 计算器
体重指数(BMI)以个体的体重和身高表示。它是体重与身高平方的比值,分别以公斤和米为单位。
BMI = (weight of body) / (height of body)2
Unit of weight: Kilogram(Kg);
Unit of height: Meter(m);
Unit of BMI is kg/m2
方法:
- 首先,我们编写HTML 代码来创建一个表单,我们将从用户那里获取姓名、身高(m)、体重(kg)作为输入。
- 导入 require 模块并将其存储到 app 变量中
- 使用此发送 html 并在特定路由上发布数据
app.post("/bmicalculator", function (req, res) {
heigh = parseFloat(req.body.Height);
weigh = parseFloat(req.body.Weight);
bmi = weigh / (heigh * heigh);
- 使用公式检查 BMI 的条件。
第1步:
HTML
BMI-CALCULATOR
BMI-CALCULATOR
Javascript
//importing modules
const express = require("express");
const bodyparser = require("body-parser");
// stores the express module into the app variable!
const app = express();
app.use(bodyparser.urlencoded({ extended: true }));
//sends index.html
app.get("/bmicalculator", function (req, res) {
res.sendFile(__dirname + "/" + "index.html");
});
//this is used to post the data on the specific route
app.post("/bmicalculator", function (req, res) {
heigh = parseFloat(req.body.Height);
weigh = parseFloat(req.body.Weight);
bmi = weigh / (heigh * heigh);
//number to string format
bmi = bmi.toFixed();
req_name = req.body.Name;
// CONDITION FOR BMI
if (bmi < 19) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Underweight!");
} else if (19 <= bmi && bmi < 25) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Normalweight!");
} else if (25 <= bmi && bmi < 30) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Overweight!");
} else {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Obese!");
}
});
//this is used to listen a specific port!
app.listen(7777, function () {
console.log("port active at 7777");
});
输出:
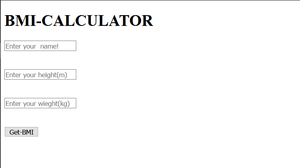
简单的形式
现在让我们为我们的计算和功能编写代码,这是我们 BMI-CALCULATOR 的主要部分。
第2步
Dependencies:
express: npm install express
bodyparser: npm install body-parser
Javascript
//importing modules
const express = require("express");
const bodyparser = require("body-parser");
// stores the express module into the app variable!
const app = express();
app.use(bodyparser.urlencoded({ extended: true }));
//sends index.html
app.get("/bmicalculator", function (req, res) {
res.sendFile(__dirname + "/" + "index.html");
});
//this is used to post the data on the specific route
app.post("/bmicalculator", function (req, res) {
heigh = parseFloat(req.body.Height);
weigh = parseFloat(req.body.Weight);
bmi = weigh / (heigh * heigh);
//number to string format
bmi = bmi.toFixed();
req_name = req.body.Name;
// CONDITION FOR BMI
if (bmi < 19) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Underweight!");
} else if (19 <= bmi && bmi < 25) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Normalweight!");
} else if (25 <= bmi && bmi < 30) {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Overweight!");
} else {
res.send("hey! " + req_name +
" your BMI is around: " + bmi +
"You are Obese!");
}
});
//this is used to listen a specific port!
app.listen(7777, function () {
console.log("port active at 7777");
});
输出:
最终输出:
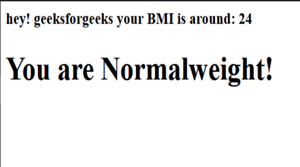
输出