原型和原型之间的区别
在本文中,我们将讨论什么是原型和原型、它们的语法、示例以及两者之间存在哪些差异,以及它们之间的区别以及它们在不同方面的区别。
原型和原型都是有助于创建数组、对象或函数的对象,并提供对这些特定方法或对象的直接访问,而无需占用内存,甚至可以访问其构造函数和所有数组方法,如 push、pop、等等。
Proto:它是一个实际的对象,它提供了一种通过 new 创建的对象来继承 JavaScript 属性的方法。每个具有关联行为的对象都有内部属性 [[prototype]]。
句法:
Object.__proto__ = value
例子:
Javascript
function Student(name,age) {
this.name = name;
this.age = age;
}
var stu1 = new Student("John", 50);
// Ubject have proto property
stu1
// Also if apply strict equal to check
// if both point at the same
// location then it will return true.
Student.prototype === stu1._proto_
Javascript
// Constructor function
function Student(name, age) {
this.name = name;
this.age = age;
}
// Objects
var stu1 = new Student("gfg1", 25);
var stu2 = new Student("gfg2", 42);
// Prototype
Student.prototype.getName = function() { return this.name; }
// Function have property prototype
// Student
// Function call using object
stu1.getName();
// Constructor function
function Student(name, age) {
this.name = name;
this.age = age;
}
// Objects
var stu1 = new Student("gfg1", 25);
var stu2 = new Student("gfg2", 42);
// Prototype
Student.prototype.getName = function() { return this.name; }
// Function have property prototype
// Student
// function call using object
stu1.getName();
// Access prototype
Student.prototype
输出:
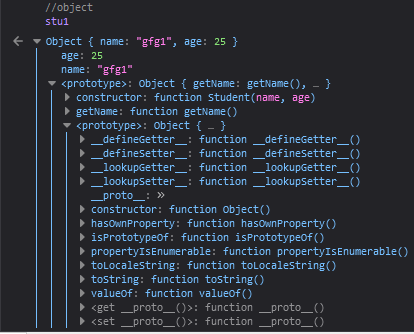
对象具有 proto 属性

对象和函数引用同一个原型
原型:它是一个特殊的对象,这意味着它拥有实例的共享属性和行为。这是一种从 javascript 继承属性的方法,因为它在每个函数声明中都可用。
句法:
objectTypeName.prototype.SharedPropertyName=value;
例子:
Javascript
// Constructor function
function Student(name, age) {
this.name = name;
this.age = age;
}
// Objects
var stu1 = new Student("gfg1", 25);
var stu2 = new Student("gfg2", 42);
// Prototype
Student.prototype.getName = function() { return this.name; }
// Function have property prototype
// Student
// Function call using object
stu1.getName();
// Constructor function
function Student(name, age) {
this.name = name;
this.age = age;
}
// Objects
var stu1 = new Student("gfg1", 25);
var stu2 = new Student("gfg2", 42);
// Prototype
Student.prototype.getName = function() { return this.name; }
// Function have property prototype
// Student
// function call using object
stu1.getName();
// Access prototype
Student.prototype
输出:
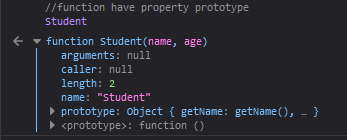
函数有属性原型
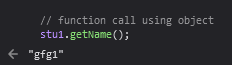
使用对象调用函数
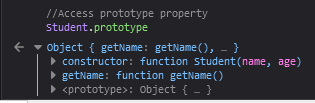
访问原型属性
原型和原型的区别: Prototype proto Prototypes is a simple way to share behavior and data between multiple objects access using .prototype proto is also a way to share behavior and data between multiple objects access using __proto__ The prototype gives access to the prototype of function using function. Syntax: (function.prototype) proto gives access to the prototype of the function using the object. Syntax: (object.__proto__) It is mostly used to resolve issues of memory wastage when creating an object in constructor mode then each object has separate behavior. It is used in the lookup chain to resolve methods, constructors, etc. The prototype property is set to function when it is declared. All the functions have a prototype property. proto property that is set to an object when it is created using a new keyword. All objects behavior newly created have proto properties.All the object constructors (function) have prototype properties. All the objects have proto property. It is the property of the class. It is the property of the instance of that class. It is introduced in EcmaScript 6. It is introduced in ECMAScript 5. It is also called it as .prototype It is also called dunder proto. It is mostly used in javaScript. It is rarely used in JavaScript.