给定数字N。任务是查找从1到N的无序整数互质数的数量。可以有多个查询。
例子:
Input: 3
Output: 4
(1, 1), (1, 2), (1, 3), (2, 3)
Input: 4
Output: 6
(1, 1), (1, 2), (1, 3), (1, 4), (2, 3), (3, 4)
方法:在这里,欧拉的Totient函数将很有帮助。表示为phi(N)的欧拉totient函数是一种算术函数,该函数对小于或等于N的正整数(相对于N而言)进行计数。
这个想法是使用Euler Totient函数的以下属性,即
- 该公式基本上说,对于n的所有素数p,Φ(n)的值等于n乘以(1/1 / p)的乘积。例如Φ(6)= 6 *(1-1 / 2)*(1 – 1/3)= 2。
- 对于素数p,Φ(p)为p-1。例如, Φ(5)是4,Φ(7)是6,Φ(13)是12。这很明显,因为p是质数,所以从1到p-1的所有数字的gcd都是1。
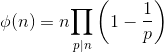
现在,使用前缀求和方法找到介于1到N之间的所有i的所有phi(x)的总和。使用此功能,您可以在o(1)时间内回答。
下面是上述方法的实现。
C++
// C++ program to find number of unordered
// coprime pairs of integers from 1 to N
#include
using namespace std;
#define N 100005
// to store euler's totient function
int phi[N];
// to store required answer
int S[N];
// Computes and prints totient of all numbers
// smaller than or equal to N.
void computeTotient()
{
// Initialise the phi[] with 1
for (int i = 1; i < N; i++)
phi[i] = i;
// Compute other Phi values
for (int p = 2; p < N; p++) {
// If phi[p] is not computed already,
// then number p is prime
if (phi[p] == p) {
// Phi of a prime number p is
// always equal to p-1.
phi[p] = p - 1;
// Update phi values of all
// multiples of p
for (int i = 2 * p; i < N; i += p) {
// Add contribution of p to its
// multiple i by multiplying with
// (1 - 1/p)
phi[i] = (phi[i] / p) * (p - 1);
}
}
}
}
// function to compute number coprime pairs
void CoPrimes()
{
// function call to compute
// euler totient function
computeTotient();
// prefix sum of all euler totient function values
for (int i = 1; i < N; i++)
S[i] = S[i - 1] + phi[i];
}
// Driver code
int main()
{
// function call
CoPrimes();
int q[] = { 3, 4 };
int n = sizeof(q) / sizeof(q[0]);
for (int i = 0; i < n; i++)
cout << "Number of unordered coprime\n"
<< "pairs of integers from 1 to "
<< q[i] << " are " << S[q[i]] << endl;
return 0;
}
Java
// Java program to find number of unordered
// coprime pairs of integers from 1 to N
import java.util.*;
import java.lang.*;
import java.io.*;
class GFG
{
static final int N = 100005;
// to store euler's
// totient function
static int[] phi;
// to store required answer
static int[] S ;
// Computes and prints totient
// of all numbers smaller than
// or equal to N.
static void computeTotient()
{
// Initialise the phi[] with 1
for (int i = 1; i < N; i++)
phi[i] = i;
// Compute other Phi values
for (int p = 2; p < N; p++)
{
// If phi[p] is not computed
// already, then number p is prime
if (phi[p] == p)
{
// Phi of a prime number p
// is always equal to p-1.
phi[p] = p - 1;
// Update phi values of
// all multiples of p
for (int i = 2 * p; i < N; i += p)
{
// Add contribution of p to
// its multiple i by multiplying
// with (1 - 1/p)
phi[i] = (phi[i] / p) * (p - 1);
}
}
}
}
// function to compute
// number coprime pairs
static void CoPrimes()
{
// function call to compute
// euler totient function
computeTotient();
// prefix sum of all euler
// totient function values
for (int i = 1; i < N; i++)
S[i] = S[i - 1] + phi[i];
}
// Driver code
public static void main(String args[])
{
phi = new int[N];
S = new int[N];
// function call
CoPrimes();
int q[] = { 3, 4 };
int n = q.length;
for (int i = 0; i < n; i++)
System.out.println("Number of unordered coprime\n" +
"pairs of integers from 1 to " +
q[i] + " are " + S[q[i]] );
}
}
// This code is contributed
// by Subhadeep
Python 3
# Python3 program to find number
# of unordered coprime pairs of
# integers from 1 to N
N = 100005
# to store euler's totient function
phi = [0] * N
# to store required answer
S = [0] * N
# Computes and prints totient of all
# numbers smaller than or equal to N.
def computeTotient():
# Initialise the phi[] with 1
for i in range(1, N):
phi[i] = i
# Compute other Phi values
for p in range(2, N) :
# If phi[p] is not computed already,
# then number p is prime
if (phi[p] == p) :
# Phi of a prime number p
# is always equal to p-1.
phi[p] = p - 1
# Update phi values of all
# multiples of p
for i in range(2 * p, N, p) :
# Add contribution of p to its
# multiple i by multiplying with
# (1 - 1/p)
phi[i] = (phi[i] // p) * (p - 1)
# function to compute number
# coprime pairs
def CoPrimes():
# function call to compute
# euler totient function
computeTotient()
# prefix sum of all euler
# totient function values
for i in range(1, N):
S[i] = S[i - 1] + phi[i]
# Driver code
if __name__ == "__main__":
# function call
CoPrimes()
q = [ 3, 4 ]
n = len(q)
for i in range(n):
print("Number of unordered coprime\n" +
"pairs of integers from 1 to ",
q[i], " are " , S[q[i]])
# This code is contributed
# by ChitraNayal
C#
// C# program to find number
// of unordered coprime pairs
// of integers from 1 to N
using System;
class GFG
{
static int N = 100005;
// to store euler's
// totient function
static int[] phi;
// to store required answer
static int[] S ;
// Computes and prints totient
// of all numbers smaller than
// or equal to N.
static void computeTotient()
{
// Initialise the phi[] with 1
for (int i = 1; i < N; i++)
phi[i] = i;
// Compute other Phi values
for (int p = 2; p < N; p++)
{
// If phi[p] is not computed
// already, then number p is prime
if (phi[p] == p)
{
// Phi of a prime number p
// is always equal to p-1.
phi[p] = p - 1;
// Update phi values of
// all multiples of p
for (int i = 2 * p;
i < N; i += p)
{
// Add contribution of
// p to its multiple i
// by multiplying
// with (1 - 1/p)
phi[i] = (phi[i] / p) * (p - 1);
}
}
}
}
// function to compute
// number coprime pairs
static void CoPrimes()
{
// function call to compute
// euler totient function
computeTotient();
// prefix sum of all euler
// totient function values
for (int i = 1; i < N; i++)
S[i] = S[i - 1] + phi[i];
}
// Driver code
public static void Main()
{
phi = new int[N];
S = new int[N];
// function call
CoPrimes();
int[] q = { 3, 4 };
int n = q.Length;
for (int i = 0; i < n; i++)
Console.WriteLine("Number of unordered coprime\n" +
"pairs of integers from 1 to " +
q[i] + " are " + S[q[i]] );
}
}
// This code is contributed
// by mits
PHP
输出:
Number of unordered coprime
pairs of integers from 1 to 3 are 4
Number of unordered coprime
pairs of integers from 1 to 4 are 6