从 Firebase 检索 Django 中的 PDF 文件
Firebase是 Google 的产品,可帮助开发人员轻松构建、管理和发展他们的应用程序。它可以帮助开发人员以更安全的方式更快地构建他们的应用程序。 firebase 方面不需要编程,这使得更有效地使用其功能变得容易。它提供云存储并使用 NoSQL 来存储数据。
另一方面, Django是一个基于 Python 的 Web 框架,它允许您快速创建 Web 应用程序,而没有您通常会在其他框架中发现的所有安装或依赖问题。
在这里,我们将学习如何实现一个简单的 Django 应用程序,该应用程序可以检索存储在 firebase 中的 PDF 文件。在本文中,我们将主要关注它的 Django 方面。如果您想继续,请在您的Firebase存储中上传一个虚拟 PDF 。如果您是 firebase 新手,请参阅此内容。
在这里,我们将学习如何在Firebase 中使用 Django 检索 PDF。
在 Django 中创建一个项目:
使用以下命令创建一个 Django 项目:
$ django-admin startproject pdffinder
让我们验证您的 Django 项目是否有效。切换到外部项目目录(如果还没有),然后运行以下命令:
$ python manage.py runserver
您将在命令行上看到以下输出:
Performing system checks...
System check identified no issues (0 silenced).
You have unapplied migrations; your app may not work properly until they are applied.
Run 'python manage.py migrate' to apply them.
April 14, 2021 - 15:50:53
Django version 3.2, using settings 'pdffinder.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
将 Firebase 数据库集成到 Django 项目:
现在,我们希望您已经在 Django 中创建了一个项目。如果没有,请参阅如何在 Django 中使用 MVT 创建基本项目?由于我们使用 firebase 作为数据库,我们需要安装pyrebase 。对于这种类型,在终端中输入以下命令:
$pip install pyrebase4
执行:
按照以下步骤从 firebase 检索 Django 中的 PDF 文件:
步骤1:进入pdffinder项目目录。
步骤 2:转到urls.py文件并创建一个路径以移动到网页以搜索数据。
Python
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('search/', views.search),
]
Python
from django.shortcuts import render
from django.views.decorators.http import require_http_methods
from django.views.decorators.csrf import csrf_exempt
from django.contrib.auth.decorators import login_required
import pyrebase
config={
"databaseURL": "YOUR DATABASE URL",
"projectId": "YOUR PROJECT ID",
}
#firebase configuration & authentication
firebase=pyrebase.initialize_app(config)
authe = firebase.auth()
database=firebase.database()
def search(request):
return render(request, "search.html")
HTML
{% load static %}
Search Page
Python
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('searchpdf/', views.searchnotes),
]
Python
from django.shortcuts import render
from django.views.decorators.http import require_http_methods
from django.views.decorators.csrf import csrf_exempt
from django.contrib.auth.decorators import login_required
import pyrebase
config = {
"databaseURL": "********************",
"projectId": "********************",
}
firebase = pyrebase.initialize_app(config)
authe = firebase.auth()
database = firebase.database()
# function to request a query
# to the firebase database
def searchpdf(request):
value = request.POST.get("search")
if value == "":
return render(request, "search.html")
title = request.POST["category"]
if title == "":
return render(request, "search.html")
if value is None or title is None:
print(value, "Value", title)
return render(request, "search.html")
else:
print(value)
if title == "Question-papers":
# search
# for content inside the node Question-papers in firebase
data = database.child("Question-papers").shallow().get().val()
id = []
# if found then append the value of i in id
for i in data:
id.append(i)
for i in id:
val = (
database.child("Question-papers")
.child(i)
.child("filename")
.get()
.val()
)
# if id is equal to what we are
# looking for the get the link or
# return to serach page
if val == value:
requid = i
fileurl = (
database.child("Question-papers")
.child(requid)
.child("fileurl")
.get()
.val()
)
return render(request, "searchNotes.html",
{"fileurl": fileurl})
else:
return render(request, "search.html")
Python
{% load static %}
Questions & Notes
第 3 步:然后移动到views.py文件并编写以下函数以呈现到 HTML 页面。
Python
from django.shortcuts import render
from django.views.decorators.http import require_http_methods
from django.views.decorators.csrf import csrf_exempt
from django.contrib.auth.decorators import login_required
import pyrebase
config={
"databaseURL": "YOUR DATABASE URL",
"projectId": "YOUR PROJECT ID",
}
#firebase configuration & authentication
firebase=pyrebase.initialize_app(config)
authe = firebase.auth()
database=firebase.database()
def search(request):
return render(request, "search.html")
第 4 步:然后我们将移动到search.html页面并编写以下代码来搜索 firebase 中的数据。注释写在里面,以便更好地理解它。
HTML
{% load static %}
Search Page
步骤5:转到urls.py文件并创建一个路径以移动到views.py中的searchnotes函数以搜索数据。
Python
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('searchpdf/', views.searchnotes),
]
第 6 步:然后移动到views.py文件并编写以下函数来搜索具有给定名称的 PDF。
Python
from django.shortcuts import render
from django.views.decorators.http import require_http_methods
from django.views.decorators.csrf import csrf_exempt
from django.contrib.auth.decorators import login_required
import pyrebase
config = {
"databaseURL": "********************",
"projectId": "********************",
}
firebase = pyrebase.initialize_app(config)
authe = firebase.auth()
database = firebase.database()
# function to request a query
# to the firebase database
def searchpdf(request):
value = request.POST.get("search")
if value == "":
return render(request, "search.html")
title = request.POST["category"]
if title == "":
return render(request, "search.html")
if value is None or title is None:
print(value, "Value", title)
return render(request, "search.html")
else:
print(value)
if title == "Question-papers":
# search
# for content inside the node Question-papers in firebase
data = database.child("Question-papers").shallow().get().val()
id = []
# if found then append the value of i in id
for i in data:
id.append(i)
for i in id:
val = (
database.child("Question-papers")
.child(i)
.child("filename")
.get()
.val()
)
# if id is equal to what we are
# looking for the get the link or
# return to serach page
if val == value:
requid = i
fileurl = (
database.child("Question-papers")
.child(requid)
.child("fileurl")
.get()
.val()
)
return render(request, "searchNotes.html",
{"fileurl": fileurl})
else:
return render(request, "search.html")
第 7 步:然后我们将转到searchnotes.html页面,并在我们从firebase获得 PDF 链接时显示 PDF。
Python
{% load static %}
Questions & Notes
输出代码:
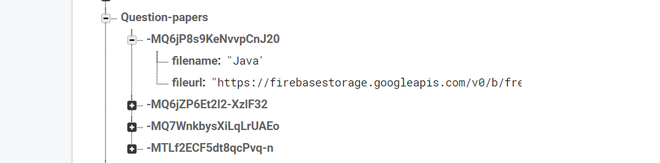