在编程中,循环用于重复代码块。例如,如果要显示一条消息100次,则可以使用循环。这只是一个简单的例子。使用循环可以实现更多目标。
在上一教程中,您了解了JavaScript for循环。在这里,您将学习while
和do...while
循环。
JavaScript while循环
while
循环的语法为:
while (condition) {
// body of loop
}
这里,
-
while
循环评估括号()
的条件 。 - 如果条件评估为
true
,则执行while
循环内的代码。 - 再次评估条件 。
- 这个过程一直持续到条件为
false
。 - 当条件评估为
false
,循环停止。
要了解有关条件的更多信息,请访问JavaScript比较和逻辑运算符。
While循环流程图

示例1:显示从1到5的数字
// program to display numbers from 1 to 5
// initialize the variable
let i = 1, n = 5;
// while loop from i = 1 to 5
while (i <= n) {
console.log(i);
i += 1;
}
输出
1
2
3
4
5
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1 n = 5 |
true |
1 is printed. i is increased to 2. |
2nd | i = 2 n = 5 |
true |
2 is printed. i is increased to 3. |
3rd | i = 3 n = 5 |
true |
3 is printed. i is increased to 4. |
4th | i = 4 n = 5 |
true |
4 is printed. i is increased to 5. |
5th | i = 5 n = 5 |
true |
5 is printed. i is increased to 6. |
6th | i = 6 n = 5 |
false |
The loop is terminated |
示例2:仅正数之和
// program to find the sum of positive numbers
// if the user enters a negative numbers, the loop ends
// the negative number entered is not added to sum
let sum = 0;
// take input from the user
let number = parseInt(prompt('Enter a number: '));
while(number >= 0) {
// add all positive numbers
sum += number;
// take input again if the number is positive
number = parseInt(prompt('Enter a number: '));
}
// display the sum
console.log(`The sum is ${sum}.`);
输出
Enter a number: 2
Enter a number: 5
Enter a number: 7
Enter a number: 0
Enter a number: -3
The sum is 14.
在上面的程序中,提示用户输入数字。
这里, parseInt()
的使用,因为prompt()
需要从所述用户作为字符串输入。当添加数字字符串时,它表现为字符串。例如, '2' + '3' = '23'
。因此parseInt()
将数字字符串转换为数字。
while
循环继续进行,直到用户输入一个负数。在每次迭代期间,将用户输入的数字添加到sum
变量中。
当用户输入负数时,循环终止。最后,显示总和。
JavaScript做… while循环
do...while
循环的语法为:
do {
// body of loop
} while(condition)
这里,
- 循环的主体首先执行。然后评估条件 。
- 如果条件评估为
true
,则将再次执行do
语句内的循环主体。 - 再次评估条件 。
- 如果条件评估为true,则将再次执行
do
语句内的循环主体。 - 这个过程一直持续到条件评估为
false
为止。然后循环停止。
注意 : do...while
循环类似于while
循环。唯一的区别是在do…while
循环中,循环主体至少执行一次。
do … while循环流程图
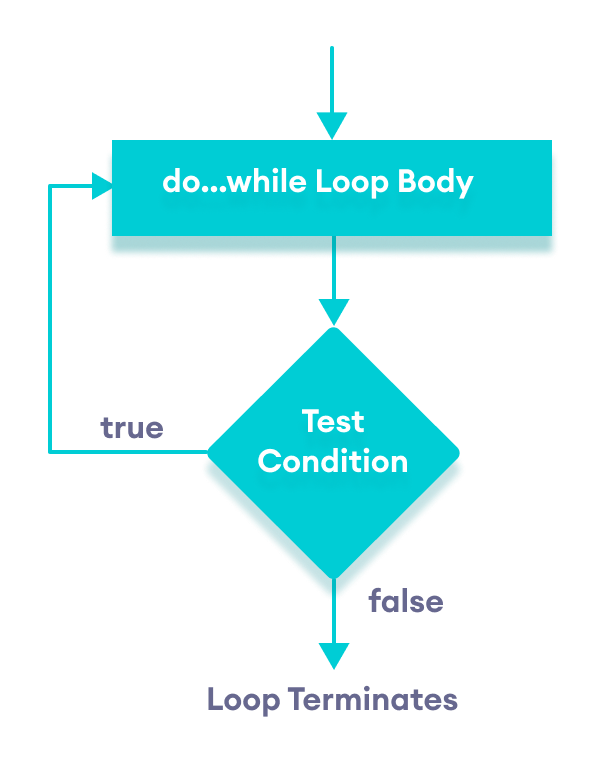
让我们看看do...while
循环的工作原理。
示例3:显示从1到5的数字
// program to display numbers
let i = 1;
let n = 5;
// do...while loop from 1 to 5
do {
console.log(i);
i++;
} while(i <= n);
输出
1
2
3
4
5
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
i = 1 n = 5 |
not checked | 1 is printed. i is increased to 2. | |
1st | i = 2 n = 5 |
true |
2 is printed. i is increased to 3. |
2nd | i = 3 n = 5 |
true |
3 is printed. i is increased to 4. |
3rd | i = 4 n = 5 |
true |
4 is printed. i is increased to 5. |
4th | i = 5 n = 5 |
true |
6 is printed. i is increased to 6. |
5th | i = 6 n = 5 |
false |
The loop is terminated |
示例4:正数之和
// to find the sum of positive numbers
// if the user enters negative number, the loop terminates
// negative number is not added to sum
let sum = 0;
let number = 0;
do {
sum += number;
number = parseInt(prompt('Enter a number: '));
} while(number >= 0)
console.log(`The sum is ${sum}.`);
输出1
Enter a number: 2
Enter a number: 4
Enter a number: -500
The sum is 6.
在这里, do...while
循环继续进行,直到用户输入一个负数。当数字为负数时,循环终止;否则为0。负数不会添加到sum变量中。
输出2
Enter a number: -80
The sum is 0.
如果用户输入负数,则do...while
循环的主体仅运行一次。
无限while循环
如果循环的条件始终为true
,则循环将运行无限次(直到内存已满)。例如,
// infinite while loop
while(true){
// body of loop
}
这是一个无限的do...while
循环的示例。
// infinite do...while loop
let count = 1;
do {
// body of loop
} while(count == 1)
在上述程序中, 条件始终为true
。因此,循环体将运行无限次。
for和while循环
当迭代次数已知时,通常使用for
循环。例如,
// this loop is iterated 5 times
for (let i = 1; i <=5; ++i) {
// body of loop
}
当迭代次数未知时,通常使用while
和do...while
循环。例如,
while (condition) {
// body of loop
}