在计算机编程中,循环用于重复代码块。例如,如果要显示一条消息100次,则可以使用循环。这只是一个简单的例子。使用循环可以实现更多目标。
在上一教程中,您了解了Java for循环。在这里,您将学习while
和do...while
循环。
Java while循环
Java while
循环用于运行特定代码,直到满足特定条件为止。 while
循环的语法为:
while (testExpression) {
// body of loop
}
这里,
-
while
循环评估括号()
内的textExpression 。 - 如果textExpression评估为
true
,则执行while
循环内的代码。 - 再次评估textExpression 。
- 这个过程一直持续到textExpression为
false
为止。 - 当textExpression计算为
false
,循环停止。
要了解有关条件的更多信息,请访问Java关系和逻辑运算符。
While循环流程图
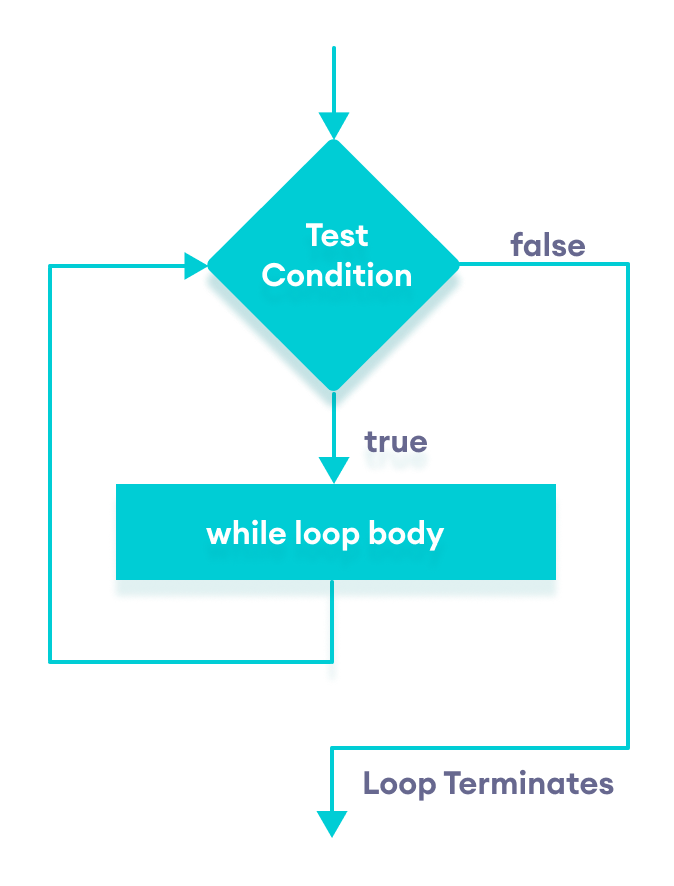
示例1:显示从1到5的数字
// Program to display numbers from 1 to 5
class Main {
public static void main(String[] args) {
// declare variables
int i = 1, n = 5;
// while loop from 1 to 5
while(i <= n) {
System.out.println(i);
i++;
}
}
}
输出
1
2
3
4
5
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
1st | i = 1 n = 5 |
true |
1 is printed. i is increased to 2. |
2nd | i = 2 n = 5 |
true |
2 is printed. i is increased to 3. |
3rd | i = 3 n = 5 |
true |
3 is printed. i is increased to 4. |
4th | i = 4 n = 5 |
true |
4 is printed. i is increased to 5. |
5th | i = 5 n = 5 |
true |
5 is printed. i is increased to 6. |
6th | i = 6 n = 5 |
false |
The loop is terminated |
示例2:仅正数之和
// Java program to find the sum of positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
// create an object of Scanner class
Scanner input = new Scanner(System.in);
// take integer input from the user
System.out.println("Enter a number");
int number = input.nextInt();
// while loop continues
// until entered number is positive
while (number >= 0) {
// add only positive numbers
sum += number;
System.out.println("Enter a number");
number = input.nextInt();
}
System.out.println("Sum = " + sum);
input.close();
}
}
输出
Enter a number
25
Enter a number
9
Enter a number
5
Enter a number
-3
Sum = 39
在上面的程序中,我们使用了Scanner类从用户那里获取输入。在这里, nextInt()
从用户处获取整数输入。
while
循环继续进行,直到用户输入一个负数。在每次迭代期间,将用户输入的数字添加到sum
变量中。
当用户输入负数时,循环终止。最后,显示总和。
Java do … while循环
do...while
循环类似于while循环。但是, do...while
循环的主体在检查测试表达式之前执行一次。例如,
do {
// body of loop
} while(textExpression)
这里,
- 循环的主体首先执行。然后评估textExpression 。
- 如果textExpression评估为
true
,则将再次执行do
语句内的循环主体。 - 再次评估textExpression 。
- 如果textExpression评估为
true
,则将再次执行do
语句内的循环主体。 - 这个过程一直持续到textExpression的值为
false
为止。然后循环停止。
do … while循环流程图
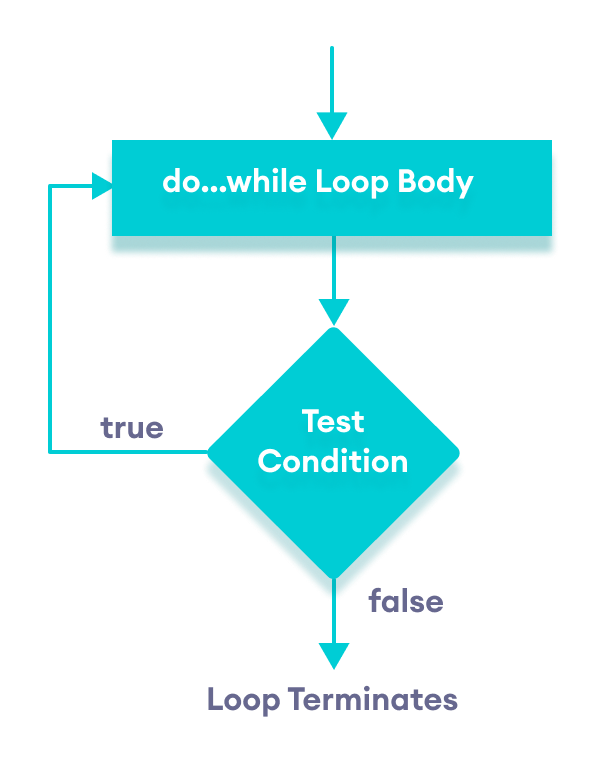
让我们看看do...while
循环的工作原理。
示例3:显示从1到5的数字
// Java Program to display numbers from 1 to 5
import java.util.Scanner;
// Program to find the sum of natural numbers from 1 to 100.
class Main {
public static void main(String[] args) {
int i = 1, n = 5;
// do...while loop from 1 to 5
do {
System.out.println(i);
i++;
} while(i <= n);
}
}
输出
1
2
3
4
5
该程序的工作原理如下。
Iteration | Variable | Condition: i <= n | Action |
---|---|---|---|
i = 1 n = 5 |
not checked | 1 is printed. i is increased to 2. |
|
1st | i = 2 n = 5 |
true |
2 is printed. i is increased to 3. |
2nd | i = 3 n = 5 |
true |
3 is printed. i is increased to 4. |
3rd | i = 4 n = 5 |
true |
4 is printed. i is increased to 5. |
4th | i = 5 n = 5 |
true |
6 is printed. i is increased to 6. |
5th | i = 6 n = 5 |
false |
The loop is terminated |
示例4:正数之和
// Java program to find the sum of positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
int number = 0;
// create an object of Scanner class
Scanner input = new Scanner(System.in);
// do...while loop continues
// until entered number is positive
do {
// add only positive numbers
sum += number;
System.out.println("Enter a number");
number = input.nextInt();
} while(number >= 0);
System.out.println("Sum = " + sum);
input.close();
}
}
输出1
Enter a number
25
Enter a number
9
Enter a number
5
Enter a number
-3
Sum = 39
在此,用户输入一个正数,该数将添加到sum变量中。这个过程一直持续到负数为止。当数字为负数时,循环终止并显示总和,而不添加负数。
输出2
Enter a number
-8
Sum is 0
用户在此处输入一个负数。测试条件将为false
但是循环内部的代码将执行一次。
无限while循环
如果循环的条件始终为true
,则循环将运行无限次(直到内存已满)。例如,
// infinite while loop
while(true){
// body of loop
}
这是一个无限的do...while
循环的示例。
// infinite do...while loop
int count = 1;
do {
// body of loop
} while(count == 1)
在上述程序中, textExpression始终为true
。因此,循环体将运行无限次。
for和while循环
当迭代次数已知时,使用for
循环。例如,
for (let i = 1; i <=5; ++i) {
// body of loop
}
当迭代次数未知时,通常使用while
和do...while
循环。例如,
while (condition) {
// body of loop
}