方阵是其中包含行和列形式的元素的矩阵。以下是5×5矩阵的示例。
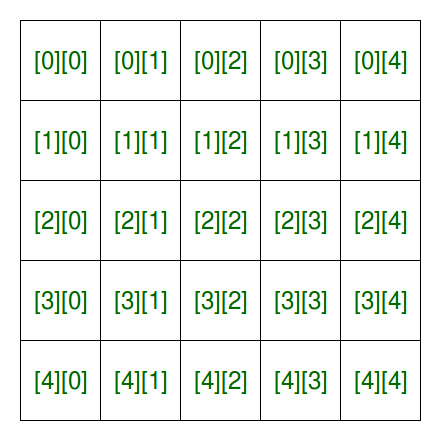
5×5方阵
可通过以下方式访问矩阵: Matrix_Name [row_index] [column_index]
以下是各种以不同形式访问方阵的方法:
- 主对角线上的元素:
方法:
row_index == column_index
C++
// C++ Program to read a square matrix // and print the main diagonal elements #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is" << endl; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the main diagonal elements cout << "\nMain diagonal elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for main diagonal elements if (row_index == column_index) cout << matrix[row_index][column_index] << ", "; } } return 0; } // This code is contributed by SHUBHAMSINGH10
C
// C Program to read a square matrix // and print the main diagonal elements #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the main diagonal elements printf("\nMain diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for main diagonal elements if (row_index == column_index) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the main diagonal elements class GFG { public static void main(String[] args) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the main diagonal elements System.out.printf("\nMain diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for main diagonal elements if (row_index == column_index) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by 29AjayKumar
Python3
# Python Program to read a square matrix # and print the main diagonal elements if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] x, size = 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print( matrix[row_index][column_index],end = "\t"); print(""); # Print the main diagonal elements print("\nMain diagonal elements are:"); for row_index in range(size): for column_index in range(size): # check for main diagonal elements if (row_index == column_index): print(matrix[row_index][column_index], end="\t"); # This code is contributed by 29AjayKumar
C#
//C# Program to read a square matrix // and print the main diagonal elements using System; public class GFG{ public static void Main() { int [,]matrix = new int[5,5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.WriteLine("The matrix is"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write(matrix[row_index,column_index]+"\t"); } Console.WriteLine(); } // Print the main diagonal elements Console.Write("\nMain diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for main diagonal elements if (row_index == column_index) { Console.Write(matrix[row_index,column_index]+","); } } } } } // This code is contributed by 29AjayKumar
C++
// C++ Program to read a square matrix // and print the elements above main diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements above main diagonal cout<<"\nElements above Main diagonal elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) cout << matrix[row_index][column_index] << ", "; } } return 0; } //This code is contributed by shubhamsingh10
C
// C Program to read a square matrix // and print the elements above the main diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements above main diagonal printf("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the elements above the main diagonal class GFG { public static void main(String args[]) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements above the main diagonal System.out.printf("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by PrinciRaj19992
Python3
# Python3 Program to read a square matrix # and print the elements above main diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)]; row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print( matrix[row_index][column_index], end = "\t"); print(""); # Print the elements above main diagonal print("\nElements above Main diagonal elements are:"); for row_index in range(size): for column_index in range(size): # check for elements above main diagonal if (row_index < column_index): print(matrix[row_index][column_index], end=" "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements above main diagonal using System; public class GFG { public static void Main() { int [,] matrix= new int[5,5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write(matrix[row_index,column_index]+"\t"); } Console.Write("\n"); } // Print the elements above the main diagonal Console.Write("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) { Console.Write(matrix[row_index,column_index]+", "); } } } } } // This code is contributed by PrinciRaj19992
C++
// C++ Program to read a square matrix // and print the elements below main diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] <<" "; } cout << endl; } // Print the elements below main diagonal cout << "\nElements below Main diagonal elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) cout << matrix[row_index][column_index]<< ", "; } } return 0; } //This code is contributed by shubhamsingh10
C
// C Program to read a square matrix // and print the elements below the main diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements below main diagonal printf("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
class GFG { // Java Program to read a square matrix // and print the elements below the main diagonal public static void main(String[] args) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements below main diagonal System.out.printf("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by PrinciRaj1992
Python3
# python Program to read a square matrix # and print the elements below main diagonal if __name__ == "__main__": matrix = [[0 for x in range(5)]for y in range(5)] x = 0 size = 5 # Get the square matrix for row_index in range(0, size): for column_index in range(0, size): x += 1 matrix[row_index][column_index] = x # Display the matrix print("The matrix is") for row_index in range(0, size): for column_index in range(0, size): print(matrix[row_index][column_index], end=" ") print() # Print the elements below main diagonal print() print("Elements below Main diagonal elements are:") for row_index in range(0, size): for column_index in range(0, size): # check for elements below main diagonal if (row_index > column_index): print(matrix[row_index][column_index], end=", ")
C#
// C# program of above approach using System; public class GFG { // Java Program to read a suare matrix // and print the elements below main diagonal public static void Main() { int [,]matrix = new int[5,5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index,column_index]); } Console.Write("\n"); } // Print the elements below main diagonal Console.Write("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) { Console.Write("{0}, ", matrix[row_index,column_index]); } } } } } // This code is contributed by Rajput-Ji
C++
// C++ Program to read a square matrix // and print the elements on secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements on secondary diagonal cout << "\nElements on Secondary diagonal:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) cout << matrix[row_index][column_index]<< ", "; } } return 0; } //This code is contributed by shubhamsingh10
C
// C Program to read a square matrix // and print the elements on secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements on secondary diagonal printf("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the elements on secondary diagonal import java.io.*; class GFG { public static void main (String[] args) { int matrix[][]=new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements on secondary diagonal System.out.printf("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } //This code is contributed by ajit.
C#
// C# Program to read a square matrix // and print the elements on secondary diagonal using System; class GFG { public static void Main (String[] args) { int [,]matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements on secondary diagonal Console.Write("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by 29AjayKumar
C++
// C++ Program to read a square matrix // and print the elements above secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements above secondary diagonal cout << "\nElements above Secondary diagonal are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) cout << matrix[row_index][column_index]<< ", "; } } return 0; } // This code is contributed by shivanisinghss2110
C
// C Program to read a square matrix // and print the elements above secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements above secondary diagonal printf("\nElements above Secondary diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the elements above secondary diagonal class GFG { public static void main(String[] args) { int[][] matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements above secondary diagonal System.out.printf("\nElements above Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by Rajput-Ji
Python3
# Python3 Program to read a square matrix # and print the elements above secondary diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)]; row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] += x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print( matrix[row_index][column_index], end = "\t"); print(""); # Print the elements above secondary diagonal print("\nElements above Secondary diagonal are:"); for row_index in range(size): for column_index in range(size): # check for elements above secondary diagonal if ((row_index + column_index) < size - 1): print(matrix[row_index][column_index], end=" "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements above // secondary diagonal using System; class GFG { public static void Main(String[] args) { int[,] matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements above // secondary diagonal Console.Write("\nElements above Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above // secondary diagonal if ((row_index + column_index) < size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by Rajput-Ji
C++
// C Program to read a square matrix // and print the elements below secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << " " << matrix[row_index][column_index] << " "; } cout << endl ; } // Print the elements below secondary diagonal cout << "\nElements below Secondary diagonal are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) cout << matrix[row_index][column_index] << " , "; } } return 0; } //This code is contributed by shivanisinghss2110
C
// C Program to read a square matrix // and print the elements below secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements below secondary diagonal printf("\nElements below Secondary diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the elements below secondary diagonal class GFG { public static void main(String[] args) { int[][] matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements below secondary diagonal System.out.printf("\nElements below Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by 29AjayKumar
Python3
# Python3 Program to read a square matrix # and print the elements below secondary diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index][column_index], end="\t"); print(""); # Print the elements below secondary diagonal print("\nElements below Secondary diagonal are:"); for row_index in range(size): for column_index in range(size): # check for elements below secondary diagonal if ((row_index + column_index) > size - 1): print(matrix[row_index][column_index], end=", "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements below secondary diagonal using System; class GFG { public static void Main(String[] args) { int[,] matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements below secondary diagonal Console.Write("\nElements below Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by 29AjayKumar
C++
// C++ Program to read a square matrix // and print the Corner Elements #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout <<"The matrix is"<< endl; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << "\t" << matrix[row_index][column_index]; } cout << endl; } // Print the Corner elements cout <<"\nCorner Elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) cout << matrix[row_index][column_index] << ","; } } return 0; } // This code is contributed by shivanisinghss2110
C
// C Program to read a square matrix // and print the Corner Elements #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the Corner elements printf("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
// Java Program to read a square matrix // and print the Corner Elements class GFG { public static void main(String[] args) { int [][]matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the Corner elements System.out.printf("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by Rajput-Ji
Python3
# Python3 Program to read a square matrix # and print the Corner Elements if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index][column_index], end = "\t"); print(""); # Print the Corner elements print("\nCorner Elements are:"); for row_index in range(size): for column_index in range(size): # check for corner elements if ((row_index == 0 or row_index == size - 1) and (column_index == 0 or column_index == size - 1)): print(matrix[row_index][column_index], end="\t"); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the Corner Elements using System; class GFG { public static void Main(String[] args) { int [,]matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the Corner elements Console.Write("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by PrinciRaj1992
C++
// C++ Program to read a square matrix // and print the Boundary Elements #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout <<"\t" << matrix[row_index][column_index]; } cout << endl; } // Print the Boundary elements cout << "\nBoundary Elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) cout << matrix[row_index][column_index] << ", " ; } } return 0; } // This code is contributed by shivanisinghss2110
C
// C Program to read a square matrix // and print the Boundary Elements #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the Boundary elements printf("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) printf("%d, ", matrix[row_index][column_index]); } } return 0; }
Java
//Java Program to read a square matrix // and print the Boundary Elements class GFG { public static void main(String[] args) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the Boundary elements System.out.printf("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by 29AjayKumar
Python3
# Python3 Program to read a square matrix # and print the Boundary Elements if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index] [column_index], end = "\t"); print(""); # Print the Boundary elements print("\nBoundary Elements are:"); for row_index in range(size): for column_index in range(size): # check for boundary elements if ((row_index == 0 or row_index == size - 1 \ or column_index == 0 or column_index == size - 1)): print(matrix[row_index] [column_index], end = ", "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the Boundary Elements using System; class GFG { static public void Main () { int [,]matrix = new int[5,5]; int row_index; int column_index; int x = 0; int size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index,column_index]); } Console.Write("\n"); } // Print the Boundary elements Console.Write("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) { Console.Write("{0},", matrix[row_index,column_index]); } } } } } // This code is contributed by ajit.
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Main diagonal elements are: 1, 7, 13, 19, 25,
- 主对角线上方的元素:
方法:
row_index < column_index
C++
// C++ Program to read a square matrix // and print the elements above main diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements above main diagonal cout<<"\nElements above Main diagonal elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) cout << matrix[row_index][column_index] << ", "; } } return 0; } //This code is contributed by shubhamsingh10 C
// C Program to read a square matrix // and print the elements above the main diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements above main diagonal printf("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
// Java Program to read a square matrix // and print the elements above the main diagonal class GFG { public static void main(String args[]) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements above the main diagonal System.out.printf("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by PrinciRaj19992
Python3
# Python3 Program to read a square matrix # and print the elements above main diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)]; row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print( matrix[row_index][column_index], end = "\t"); print(""); # Print the elements above main diagonal print("\nElements above Main diagonal elements are:"); for row_index in range(size): for column_index in range(size): # check for elements above main diagonal if (row_index < column_index): print(matrix[row_index][column_index], end=" "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements above main diagonal using System; public class GFG { public static void Main() { int [,] matrix= new int[5,5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write(matrix[row_index,column_index]+"\t"); } Console.Write("\n"); } // Print the elements above the main diagonal Console.Write("\nElements above Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above main diagonal if (row_index < column_index) { Console.Write(matrix[row_index,column_index]+", "); } } } } } // This code is contributed by PrinciRaj19992
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Elements above Main diagonal elements are: 2, 3, 4, 5, 8, 9, 10, 14, 15, 20,
- 主对角线以下的元素:
方法:
row_index > column_index
C++
// C++ Program to read a square matrix // and print the elements below main diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] <<" "; } cout << endl; } // Print the elements below main diagonal cout << "\nElements below Main diagonal elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) cout << matrix[row_index][column_index]<< ", "; } } return 0; } //This code is contributed by shubhamsingh10 C
// C Program to read a square matrix // and print the elements below the main diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements below main diagonal printf("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
class GFG { // Java Program to read a square matrix // and print the elements below the main diagonal public static void main(String[] args) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements below main diagonal System.out.printf("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by PrinciRaj1992
Python3
# python Program to read a square matrix # and print the elements below main diagonal if __name__ == "__main__": matrix = [[0 for x in range(5)]for y in range(5)] x = 0 size = 5 # Get the square matrix for row_index in range(0, size): for column_index in range(0, size): x += 1 matrix[row_index][column_index] = x # Display the matrix print("The matrix is") for row_index in range(0, size): for column_index in range(0, size): print(matrix[row_index][column_index], end=" ") print() # Print the elements below main diagonal print() print("Elements below Main diagonal elements are:") for row_index in range(0, size): for column_index in range(0, size): # check for elements below main diagonal if (row_index > column_index): print(matrix[row_index][column_index], end=", ")
C#
// C# program of above approach using System; public class GFG { // Java Program to read a suare matrix // and print the elements below main diagonal public static void Main() { int [,]matrix = new int[5,5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index,column_index]); } Console.Write("\n"); } // Print the elements below main diagonal Console.Write("\nElements below Main diagonal elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below main diagonal if (row_index > column_index) { Console.Write("{0}, ", matrix[row_index,column_index]); } } } } } // This code is contributed by Rajput-Ji
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Elements below Main diagonal elements are: 6, 11, 12, 16, 17, 18, 21, 22, 23, 24,
- 次要对角线上的元素:
方法:
(row_index + column_index) == size-1
C++
// C++ Program to read a square matrix // and print the elements on secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements on secondary diagonal cout << "\nElements on Secondary diagonal:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) cout << matrix[row_index][column_index]<< ", "; } } return 0; } //This code is contributed by shubhamsingh10 C
// C Program to read a square matrix // and print the elements on secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements on secondary diagonal printf("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
// Java Program to read a square matrix // and print the elements on secondary diagonal import java.io.*; class GFG { public static void main (String[] args) { int matrix[][]=new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements on secondary diagonal System.out.printf("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } //This code is contributed by ajit.
C#
// C# Program to read a square matrix // and print the elements on secondary diagonal using System; class GFG { public static void Main (String[] args) { int [,]matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements on secondary diagonal Console.Write("\nElements on Secondary diagonal:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements on secondary diagonal if ((row_index + column_index) == size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by 29AjayKumar
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Elements on Secondary diagonal: 5, 9, 13, 17, 21,
- 次要对角线上方元素的地址:
方法:
(row_index + column_index) < size-1
C++
// C++ Program to read a square matrix // and print the elements above secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << matrix[row_index][column_index] << " "; } cout << endl; } // Print the elements above secondary diagonal cout << "\nElements above Secondary diagonal are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) cout << matrix[row_index][column_index]<< ", "; } } return 0; } // This code is contributed by shivanisinghss2110 C
// C Program to read a square matrix // and print the elements above secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements above secondary diagonal printf("\nElements above Secondary diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
// Java Program to read a square matrix // and print the elements above secondary diagonal class GFG { public static void main(String[] args) { int[][] matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements above secondary diagonal System.out.printf("\nElements above Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above secondary diagonal if ((row_index + column_index) < size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by Rajput-Ji
Python3
# Python3 Program to read a square matrix # and print the elements above secondary diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)]; row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] += x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print( matrix[row_index][column_index], end = "\t"); print(""); # Print the elements above secondary diagonal print("\nElements above Secondary diagonal are:"); for row_index in range(size): for column_index in range(size): # check for elements above secondary diagonal if ((row_index + column_index) < size - 1): print(matrix[row_index][column_index], end=" "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements above // secondary diagonal using System; class GFG { public static void Main(String[] args) { int[,] matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements above // secondary diagonal Console.Write("\nElements above Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements above // secondary diagonal if ((row_index + column_index) < size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by Rajput-Ji
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Elements above Secondary diagonal are: 1, 2, 3, 4, 6, 7, 8, 11, 12, 16,
- 次要对角线以下的元素:
方法:
(row_index + column_index) > size-1
C++
// C Program to read a square matrix // and print the elements below secondary diagonal #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << " " << matrix[row_index][column_index] << " "; } cout << endl ; } // Print the elements below secondary diagonal cout << "\nElements below Secondary diagonal are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) cout << matrix[row_index][column_index] << " , "; } } return 0; } //This code is contributed by shivanisinghss2110 C
// C Program to read a square matrix // and print the elements below secondary diagonal #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the elements below secondary diagonal printf("\nElements below Secondary diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
// Java Program to read a square matrix // and print the elements below secondary diagonal class GFG { public static void main(String[] args) { int[][] matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the elements below secondary diagonal System.out.printf("\nElements below Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by 29AjayKumar
Python3
# Python3 Program to read a square matrix # and print the elements below secondary diagonal if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index][column_index], end="\t"); print(""); # Print the elements below secondary diagonal print("\nElements below Secondary diagonal are:"); for row_index in range(size): for column_index in range(size): # check for elements below secondary diagonal if ((row_index + column_index) > size - 1): print(matrix[row_index][column_index], end=", "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the elements below secondary diagonal using System; class GFG { public static void Main(String[] args) { int[,] matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the elements below secondary diagonal Console.Write("\nElements below Secondary" + " diagonal are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for elements below secondary diagonal if ((row_index + column_index) > size - 1) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by 29AjayKumar
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Elements below Secondary diagonal are: 10, 14, 15, 18, 19, 20, 22, 23, 24, 25,
- 角元素:
方法:
( row_index == 0 || row_index == size-1 ) && ( column_index == 0 || column_index == size-1 )
C++
// C++ Program to read a square matrix // and print the Corner Elements #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout <<"The matrix is"<< endl; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout << "\t" << matrix[row_index][column_index]; } cout << endl; } // Print the Corner elements cout <<"\nCorner Elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) cout << matrix[row_index][column_index] << ","; } } return 0; } // This code is contributed by shivanisinghss2110 C
// C Program to read a square matrix // and print the Corner Elements #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the Corner elements printf("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
// Java Program to read a square matrix // and print the Corner Elements class GFG { public static void main(String[] args) { int [][]matrix = new int[5][5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the Corner elements System.out.printf("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) System.out.printf("%d, ", matrix[row_index][column_index]); } } } } // This code is contributed by Rajput-Ji
Python3
# Python3 Program to read a square matrix # and print the Corner Elements if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index][column_index], end = "\t"); print(""); # Print the Corner elements print("\nCorner Elements are:"); for row_index in range(size): for column_index in range(size): # check for corner elements if ((row_index == 0 or row_index == size - 1) and (column_index == 0 or column_index == size - 1)): print(matrix[row_index][column_index], end="\t"); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the Corner Elements using System; class GFG { public static void Main(String[] args) { int [,]matrix = new int[5, 5]; int row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index, column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index, column_index]); } Console.Write("\n"); } // Print the Corner elements Console.Write("\nCorner Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for corner elements if ((row_index == 0 || row_index == size - 1) && (column_index == 0 || column_index == size - 1)) Console.Write("{0}, ", matrix[row_index, column_index]); } } } } // This code is contributed by PrinciRaj1992
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Corner Elements are: 1, 5, 21, 25,
- 边界元素:
方法:
( row_index == 0 || row_index == size-1 || column_index == 0 || column_index == size-1 )
C++
// C++ Program to read a square matrix // and print the Boundary Elements #include
using namespace std; int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix cout << "The matrix is\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { cout <<"\t" << matrix[row_index][column_index]; } cout << endl; } // Print the Boundary elements cout << "\nBoundary Elements are:\n"; for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) cout << matrix[row_index][column_index] << ", " ; } } return 0; } // This code is contributed by shivanisinghss2110 C
// C Program to read a square matrix // and print the Boundary Elements #include
int main() { int matrix[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { printf("%d\t", matrix[row_index][column_index]); } printf("\n"); } // Print the Boundary elements printf("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) printf("%d, ", matrix[row_index][column_index]); } } return 0; } Java
//Java Program to read a square matrix // and print the Boundary Elements class GFG { public static void main(String[] args) { int matrix[][] = new int[5][5], row_index, column_index, x = 0, size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index][column_index] = ++x; } } // Display the matrix System.out.printf("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { System.out.printf("%d\t", matrix[row_index][column_index]); } System.out.printf("\n"); } // Print the Boundary elements System.out.printf("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) { System.out.printf("%d, ", matrix[row_index][column_index]); } } } } } // This code is contributed by 29AjayKumar
Python3
# Python3 Program to read a square matrix # and print the Boundary Elements if __name__ == '__main__': matrix = [[0 for i in range(5)] for j in range(5)] row_index, column_index, x, size = 0, 0, 0, 5; # Get the square matrix for row_index in range(size): for column_index in range(size): x += 1; matrix[row_index][column_index] = x; # Display the matrix print("The matrix is"); for row_index in range(size): for column_index in range(size): print(matrix[row_index] [column_index], end = "\t"); print(""); # Print the Boundary elements print("\nBoundary Elements are:"); for row_index in range(size): for column_index in range(size): # check for boundary elements if ((row_index == 0 or row_index == size - 1 \ or column_index == 0 or column_index == size - 1)): print(matrix[row_index] [column_index], end = ", "); # This code is contributed by 29AjayKumar
C#
// C# Program to read a square matrix // and print the Boundary Elements using System; class GFG { static public void Main () { int [,]matrix = new int[5,5]; int row_index; int column_index; int x = 0; int size = 5; // Get the square matrix for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { matrix[row_index,column_index] = ++x; } } // Display the matrix Console.Write("The matrix is\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { Console.Write("{0}\t", matrix[row_index,column_index]); } Console.Write("\n"); } // Print the Boundary elements Console.Write("\nBoundary Elements are:\n"); for (row_index = 0; row_index < size; row_index++) { for (column_index = 0; column_index < size; column_index++) { // check for boundary elements if ((row_index == 0 || row_index == size - 1 || column_index == 0 || column_index == size - 1)) { Console.Write("{0},", matrix[row_index,column_index]); } } } } } // This code is contributed by ajit.
输出:The matrix is 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Boundary Elements are: 1, 2, 3, 4, 5, 6, 10, 11, 15, 16, 20, 21, 22, 23, 24, 25,