用于打开 Web 浏览器的Python脚本
在本文中,我们将讨论一些可用于打开 Web 浏览器(我们选择)并使用Python脚本访问我们指定的 URL 的方法。
在Python包中,我们有一个名为webbrowser 的模块,其中包含许多方法,我们可以使用这些方法在我们想要的任何指定浏览器中打开所需的 URL。为此,我们只需要将这个模块导入到我们的脚本文件中,然后我们必须使用我们所需的输入调用它的一些函数(在下面声明和定义)。因此,该模块将打开我们想要的浏览器并获得我们想要的页面。
本模块中的方法如下所述:S No. Syntax of Method Description 1 webbrowser.open(url, new = 0, autoraise = true) This is the main method where the web browser with the passed URL is opened and is displayed to the user. If the parameter “new” is 0 then URL is opened in the same browser and if it is 1 then URL is opened in another browser and if it’s 2, then the page is opened in another tab. 2 webbrowser.open_new(url) URL passed is opened in the a new browser if it is possible to do that, else it is opened in default one. 3 webbrowser.open_new_tab(url) Opens new tab of passpage URL passed in the browser which is currently active. 4 webbrowser.get(using=None) This command is used to get the object code for the web browser we want to use. In simple words,, we could use this command to get the code of the web browser (stored in python) and then we could use that code to open that particular web browser. We passes the name of the web browser which we want to use as a string. 5 webbrowser.register(name, constructor, instance=None, preferred=False) This method is used to register the name of the favorite browser in the Python environment if its code was not registered previously. Actually, at the beginning, none of the browsers is registered and only the default one is called each time. Hence we had to register them manually.
现在我们将使用这些方法来查看如何使用传递的 URL 打开浏览器。
下面是实现:
我们在这个实验中使用了 URL = https://www.geeksforgeeks.org。我们的Python脚本存储在一个名为 geeks.py 的文件中。下面是我们在其中编写和实现的代码。
示例 1:在Python脚本中打开 Web 浏览器的基本示例
Python3
# first import the module
import webbrowser
# then make a url variable
url = "https://www.geeksforgeeks.org"
# then call the default open method described above
webbrowser.open(url)
Python3
# first import the module
import webbrowser
# then make a url variable
url = "https://www.geeksforgeeks.org"
# then call the get method to select the code
# for new browser and call open method
# described above
webbrowser.get('chrome').open(url)
# results in error since chrome is not registered initially.
Python3
# first import the module
import webbrowser
# then make a url variable
url = "https://www.geeksforgeeks.org"
# getting path
chrome_path = r"C:\Program Files\Google\Chrome\Application\chrome.exe"
# First registers the new browser
webbrowser.register('chrome', None,
webbrowser.BackgroundBrowser(chrome_path))
# after registering we can open it by getting its code.
webbrowser.get('chrome').open(url)
因此,该网站在默认 Web 浏览器中打开。和我的一样,它在 Microsoft Edge 中打开,如下所示:

输出:- 在 Edge 中打开的站点
示例 2:指定浏览器
Python3
# first import the module
import webbrowser
# then make a url variable
url = "https://www.geeksforgeeks.org"
# then call the get method to select the code
# for new browser and call open method
# described above
webbrowser.get('chrome').open(url)
# results in error since chrome is not registered initially.
通过键入“ Python geeks.py ”在提示符下运行命令,它将给出结果。如果我们在第一次没有注册的情况下尝试打开我们选择的浏览器,那么我们将得到下面给出的响应类型作为输出。
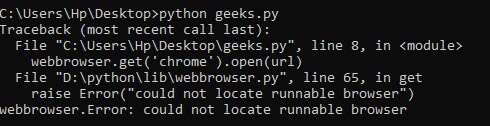
如果我们最初没有注册浏览器,则输出
因此,下面给出的是我们修改的代码,以便它现在注册浏览器,然后在其中打开该 URL。
示例 3:注册新浏览器
Python3
# first import the module
import webbrowser
# then make a url variable
url = "https://www.geeksforgeeks.org"
# getting path
chrome_path = r"C:\Program Files\Google\Chrome\Application\chrome.exe"
# First registers the new browser
webbrowser.register('chrome', None,
webbrowser.BackgroundBrowser(chrome_path))
# after registering we can open it by getting its code.
webbrowser.get('chrome').open(url)
在提示符中使用该命令的输出与上面给出的相同,只是方法不同。
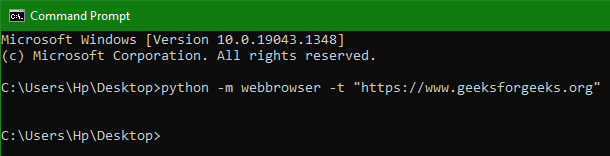
在提示中使用Python脚本。
因此,执行后它将打开 chrome Web 浏览器。
还有另一种方法可以在Python中使用 webbrowser 打开浏览器。在这种方法中,我们不必编写整个脚本并解释它来打开浏览器。我们可以只使用Python shell 执行单个命令(如下所示)以打开具有指定 URL 的浏览器(默认)。
The shell command is given as:-
python -m webbrowser -t “https://www.geeksforgeeks.org”
因此,我们看到了两种不同的方法,并向他们解释了如何使用Python脚本打开 Web 浏览器。