Next.js 中的数据获取方法
Next.js是一个基于 React 的全栈框架,支持网页预渲染等功能。与将整个应用程序加载到客户端的传统 React 应用程序不同,Next.js 允许在服务器上呈现网页,这对性能和 SEO 来说非常有用。您可以在此处了解有关 Next.js 的更多信息。
Next.js 提供了三种数据获取方法,并基于这些方法以不同的方式呈现内容。 (您可以在此处了解不同的渲染方法。
- 获取静态道具
- 获取静态路径
- 获取ServerSideProps
getStaticProps:它预加载给定页面所需的所有数据,并在构建时在用户请求之前呈现页面。为了更快地检索,所有数据都缓存在无头 CMS 上。为了获得更好的 SEO 性能,页面被预渲染和缓存。如果没有指定其他数据获取方法,Next.js 将默认使用此方法。它用于实现静态站点生成和增量站点再生。
getStaticProps 的属性:
- 只能从页面文件导出,不能从组件文件导出。
- 它只在构建时运行。
- 它在开发模式下在每个后续请求上运行。
- 它的代码完全从客户端包中排除。
getStaticPaths:如果页面使用 getStaticProps 并且具有动态路由,则必须声明将静态生成的路径列表。当我们从页面导出名为 getStaticPaths 的函数时,Next.js 将静态预渲染所有由 getStaticPaths 定义的路径。
getStaticPaths 的属性:
- 它只能从页面文件中导出。
- 它适用于动态路由。
- 页面还必须实现 getStaticProps。
- 它仅在生产中的构建时运行。
- 它在开发模式下运行在每个请求上。
getServerSideProps:它将在每个后续请求中预渲染页面。与 getStaticProps 相比,它要慢一些,因为每次请求都会呈现页面。 getServerSideProps props 返回 JSON,它将用于呈现页面所有这些工作将由 Next.js 自动处理。它可用于直接从 getServerSideProps 调用 CMS、数据库或其他 API。它用于实现服务器端渲染。
getServerSideProps 的属性:
- 它在开发和生产模式中的每个后续请求上运行。
- 它的代码被排除在客户端包之外。
- 它只能从页面文件中导出。
何时使用哪种数据获取方法:如果您的页面内容是静态的或不经常更改,那么您应该使用 getStaticProps,因为它会在构建时构建页面,从而提高性能。如果您的页面有动态路由,那么 getStaticPaths 应该与 getStaticProps 一起使用。
但是,如果您的网站包含一个数据更改非常频繁的页面,那么您必须使用 getServerSideProps,因为它会在每次请求时获取新数据。
示例:我们将构建一个简单的 Next Js 应用程序,其中包含三个页面的相册、帖子和一个带有动态路由的用户页面。这三个页面都将实现不同的数据获取方法。对于这个例子,我们将使用 JSONPlaceholder API 来获取随机数据。
运行以下命令创建一个新的 Next Js 应用程序(确保您已安装 NPM 和节点):
npx create-next-app@latest myproject
当我们在代码编辑器中打开我们的项目时,我们会看到一个简单的项目结构。对于本教程的范围,我们将只关注 /pages 目录。我们将首先清理 /pages/index.js 文件。然后我们将创建两个新页面相册、帖子和一个动态路由页面/users/[id]。
项目结构:
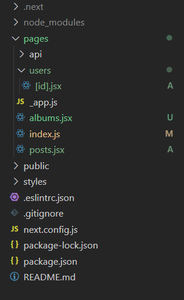
/pages/index.js –我们将首先清理主页(索引),删除所有样板代码并添加指向我们将要实现的所有页面的链接,以便于导航。
Javascript
import React from 'react'
import Link from 'next/link'
const Home = () => {
// This is the home page which will
// contain links to all other pages
return (
<>
Hello Geeks
-
getStaticProps :
About Page
-
getStaticPaths :
User 1
-
getServerSideProps :
Posts Page
>
)
}
export default Home
Javascript
import React from 'react'
export const getStaticProps = async () => {
// Fetching data from jsonplaceholder.
const res = await fetch(
'https://jsonplaceholder.typicode.com/albums');
let allAlbums = await res.json();
// Sending fetched data to the page component via props.
return {
props: {
allAlbums: allAlbums.map((album) => album.title)
}
}
}
const Albums = ({ allAlbums }) => {
return (
All Albums
{allAlbums.map((album, idx) => (
{album}))
}
)
}
export default Albums
Javascript
import React from 'react'
export const getServerSideProps = async (ctx) => {
// ctx is the context object which contains the request,
// response and props passed to the page.
// fetching data from jsonplaceholder.
const res = await fetch(
'https://jsonplaceholder.typicode.com/posts');
let allPosts = await res.json();
// Sending fetched data to the page component via props.
return {
props: {
allPosts: allPosts.map((post) => post.title)
}
}
}
const Posts = ({ allPosts }) => {
return (
All Posts
{allPosts.map((post, idx) => (
{post}))}
)
}
export default Posts
Javascript
import React from "react";
export const getStaticProps = async (ctx) => {
// ctx will contain request parameters
const { params } = ctx;
// We will destructure id from the parameters
const userId = params.id;
// Fetching user data
const res = await fetch(
`https://jsonplaceholder.typicode.com/users/${userId}`
);
const userData = await res.json();
// Sending data to the page via props
return {
props: {
user: userData,
},
};
};
export const getStaticPaths = () => {
// Specifying all the routes to be
// pre-rendered by next js
return {
paths: [
{ params: { id: "1" } },
{ params: { id: "2" } },
{ params: { id: "3" } },
{ params: { id: "4" } },
{ params: { id: "5" } },
{ params: { id: "6" } },
{ params: { id: "7" } },
{ params: { id: "8" } },
{ params: { id: "9" } },
{ params: { id: "10" } },
],
fallback: false,
};
};
const User = ({ user }) => {
return (
<>
User {user.id}
Name : {user.name}
Email : {user.email}
>
);
};
export default User;
/pages/albums.jsx -相册页面将使用getStaticProps实现静态站点生成,我们将与页面组件一起导出数据获取方法。我们可以使用 props 将获取的数据发送到页面组件。 Next Js 将在用户请求之前的构建时获取所有专辑。
Javascript
import React from 'react'
export const getStaticProps = async () => {
// Fetching data from jsonplaceholder.
const res = await fetch(
'https://jsonplaceholder.typicode.com/albums');
let allAlbums = await res.json();
// Sending fetched data to the page component via props.
return {
props: {
allAlbums: allAlbums.map((album) => album.title)
}
}
}
const Albums = ({ allAlbums }) => {
return (
All Albums
{allAlbums.map((album, idx) => (
{album}))
}
)
}
export default Albums
/pages/posts.jsx -帖子页面将使用getServerSideProps实现服务器端渲染。它将在用户提出的每个请求中获取帖子数据并构建页面,并使用道具将获取的数据发送到组件。
Javascript
import React from 'react'
export const getServerSideProps = async (ctx) => {
// ctx is the context object which contains the request,
// response and props passed to the page.
// fetching data from jsonplaceholder.
const res = await fetch(
'https://jsonplaceholder.typicode.com/posts');
let allPosts = await res.json();
// Sending fetched data to the page component via props.
return {
props: {
allPosts: allPosts.map((post) => post.title)
}
}
}
const Posts = ({ allPosts }) => {
return (
All Posts
{allPosts.map((post, idx) => (
{post}))}
)
}
export default Posts
/pages/users/[id].jsx -因为这是一个动态页面,我们必须预先定义所有用户 ID,以便 Next Js 可以在构建时检索他们的数据。因此,我们使用getStaticPaths并定义了十个用户 ID。
Javascript
import React from "react";
export const getStaticProps = async (ctx) => {
// ctx will contain request parameters
const { params } = ctx;
// We will destructure id from the parameters
const userId = params.id;
// Fetching user data
const res = await fetch(
`https://jsonplaceholder.typicode.com/users/${userId}`
);
const userData = await res.json();
// Sending data to the page via props
return {
props: {
user: userData,
},
};
};
export const getStaticPaths = () => {
// Specifying all the routes to be
// pre-rendered by next js
return {
paths: [
{ params: { id: "1" } },
{ params: { id: "2" } },
{ params: { id: "3" } },
{ params: { id: "4" } },
{ params: { id: "5" } },
{ params: { id: "6" } },
{ params: { id: "7" } },
{ params: { id: "8" } },
{ params: { id: "9" } },
{ params: { id: "10" } },
],
fallback: false,
};
};
const User = ({ user }) => {
return (
<>
User {user.id}
Name : {user.name}
Email : {user.email}
>
);
};
export default User;
运行应用程序:打开终端并输入以下命令。
npm run dev
输出:
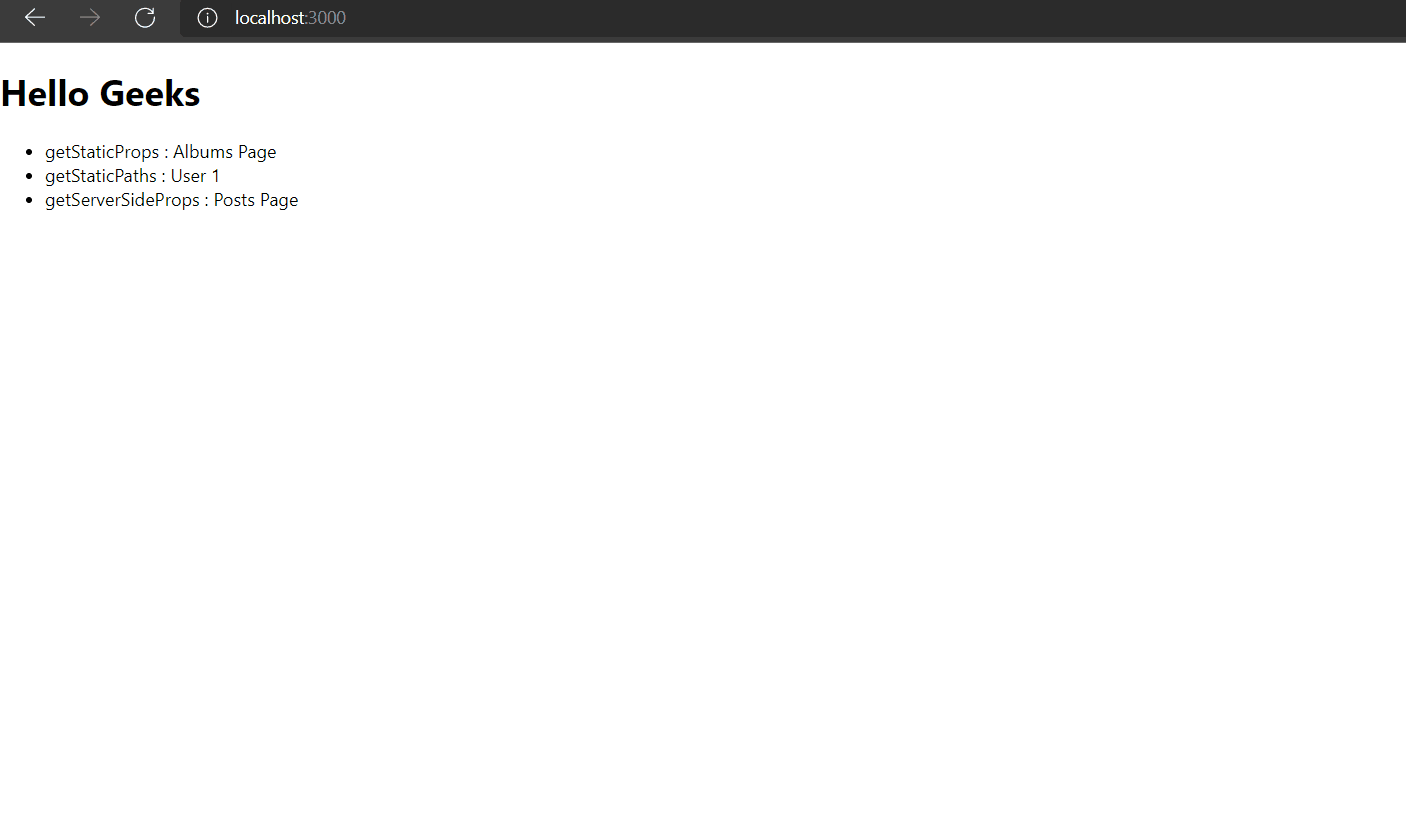