使用Python为图像添加“盐和胡椒”噪声
在本文中,我们将看到如何使用Python向图像添加“椒盐”噪声。
噪声:噪声是指计算机版本中信号中的随机干扰。在我们的例子中,信号是一个图像。图像亮度和颜色的随机干扰称为图像噪声。
Salt-and-pepper:仅在灰度图像(黑白图像)中发现。顾名思义,胡椒(黑色)中的盐(白色)-黑暗区域中的白色斑点或盐(白色)中的胡椒(黑色)-白色区域中的黑色斑点。换句话说,具有椒盐噪声的图像将在明亮区域中具有少量暗像素,在暗区域中具有少量亮像素。椒盐噪声也称为脉冲噪声。它可能由多种原因引起,例如坏点、模数转换错误、位传输错误等。
让我们看看如何在图像中添加椒盐噪声——
- 椒盐噪声只能添加到灰度图像中。因此,读取图像后将其转换为灰度
- 随机选择添加噪声的像素数(number_of_pixels)
- 在图像中随机选取一些将添加噪声的像素。可以通过随机选取 x 和 y 坐标来完成
- 注意生成的随机值必须在图像尺寸范围内。 x 和 y 坐标必须在图像大小的范围内
- 可以使用随机数生成器函数生成随机数,如代码中使用的 random.randint
- 将一些随机选取的像素着色为黑色,将它们的值设置为 0
- 将一些随机选取的像素着色为白色,将它们的值设置为 255
- 保存图像的值
下面是实现:
Python
import random
import cv2
def add_noise(img):
# Getting the dimensions of the image
row , col = img.shape
# Randomly pick some pixels in the
# image for coloring them white
# Pick a random number between 300 and 10000
number_of_pixels = random.randint(300, 10000)
for i in range(number_of_pixels):
# Pick a random y coordinate
y_coord=random.randint(0, row - 1)
# Pick a random x coordinate
x_coord=random.randint(0, col - 1)
# Color that pixel to white
img[y_coord][x_coord] = 255
# Randomly pick some pixels in
# the image for coloring them black
# Pick a random number between 300 and 10000
number_of_pixels = random.randint(300 , 10000)
for i in range(number_of_pixels):
# Pick a random y coordinate
y_coord=random.randint(0, row - 1)
# Pick a random x coordinate
x_coord=random.randint(0, col - 1)
# Color that pixel to black
img[y_coord][x_coord] = 0
return img
# salt-and-pepper noise can
# be applied only to greyscale images
# Reading the color image in greyscale image
img = cv2.imread('lena.jpg',
cv2.IMREAD_GRAYSCALE)
#Storing the image
cv2.imwrite('salt-and-pepper-lena.jpg',
add_noise(img))
输出:
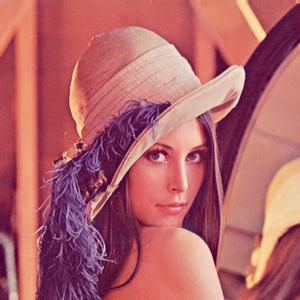
输入图像:“lena.jpg”
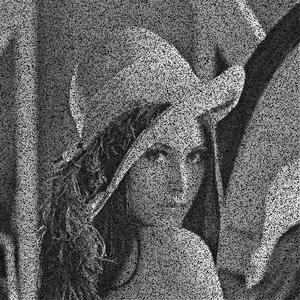
输出图像:“Salt-and-pepper-lena.jpg”