使用PythonSOAP API 调用
SOAP 代表简单对象访问协议,顾名思义,它只是一种用于在节点之间交换结构化数据的协议。它使用 XML 而不是 JSON。
在本文中,我们将了解如何使用Python进行 SOAP API 调用。如果您想测试负载和响应究竟是什么样子,您可以使用以下 curl 命令:
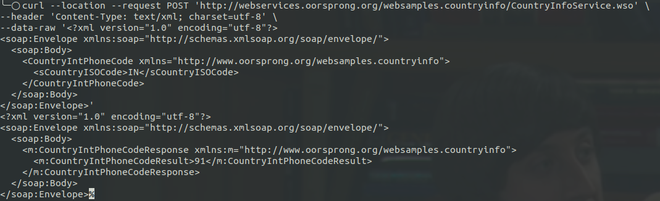
卷曲输出
方法一:使用请求
首先,我们导入requests库,然后定义 SOAP URL。
下一个也是最重要的步骤是根据 SOAP URL 中提供的结构格式化 XML 主体。要了解格式,只需访问 SOAP URL 并单击CountryISOCode链接并相应地格式化 XML。
然后,您只需准备标头并进行 POST 调用。
代码:
Python3
import requests
# SOAP request URL
url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso"
# structured XML
payload = """
IN
"""
# headers
headers = {
'Content-Type': 'text/xml; charset=utf-8'
}
# POST request
response = requests.request("POST", url, headers=headers, data=payload)
# prints the response
print(response.text)
print(response)
Python3
import zeep
# set the WSDL URL
wsdl_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL"
# set method URL
method_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryIntPhoneCode"
# set service URL
service_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso"
# create the header element
header = zeep.xsd.Element(
"Header",
zeep.xsd.ComplexType(
[
zeep.xsd.Element(
"{http://www.w3.org/2005/08/addressing}Action", zeep.xsd.String()
),
zeep.xsd.Element(
"{http://www.w3.org/2005/08/addressing}To", zeep.xsd.String()
),
]
),
)
# set the header value from header element
header_value = header(Action=method_url, To=service_url)
# initialize zeep client
client = zeep.Client(wsdl=wsdl_url)
# set country code for India
country_code = "IN"
# make the service call
result = client.service.CountryIntPhoneCode(
sCountryISOCode=country_code,
_soapheaders=[header_value]
)
# print the result
print(f"Phone Code for {country_code} is {result}")
# set country code for United States
country_code = "US"
# make the service call
result = client.service.CountryIntPhoneCode(
sCountryISOCode=country_code,
_soapheaders=[header_value]
)
# print the result
print(f"Phone Code for {country_code} is {result}")
print(response)
输出:
91
方法 2:使用 Zeep
现在我们已经了解了如何使用请求进行 SOAP 调用,我们将看到通过 Zeep 进行调用是多么容易。首先,您需要安装zeep 。
pip3 install zeep
方法:
- 首先,设置 WSDL URL。您可以通过访问基本 URL 并单击Service Description来获取 WSDL URL。这将带您到 WSDL URL。基本 URL 将是service_url并在基本 URL 后附加服务名称。
- 接下来,您需要创建一个标题元素。现在,您需要使用method_url和service_url设置标题元素。
- 现在,使用 WSDL URL 初始化 zeep 客户端。
- 所有设置都完成了,现在您只需要使用服务名称调用 zeep 服务,这里的服务名称是CountryIntPhoneCode 。您需要使用country_code传递参数,并将标头作为列表传递给_soapheaders 。
- 现在,这将直接返回该国家/地区的电话代码。
代码:
蟒蛇3
import zeep
# set the WSDL URL
wsdl_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL"
# set method URL
method_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryIntPhoneCode"
# set service URL
service_url = "http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso"
# create the header element
header = zeep.xsd.Element(
"Header",
zeep.xsd.ComplexType(
[
zeep.xsd.Element(
"{http://www.w3.org/2005/08/addressing}Action", zeep.xsd.String()
),
zeep.xsd.Element(
"{http://www.w3.org/2005/08/addressing}To", zeep.xsd.String()
),
]
),
)
# set the header value from header element
header_value = header(Action=method_url, To=service_url)
# initialize zeep client
client = zeep.Client(wsdl=wsdl_url)
# set country code for India
country_code = "IN"
# make the service call
result = client.service.CountryIntPhoneCode(
sCountryISOCode=country_code,
_soapheaders=[header_value]
)
# print the result
print(f"Phone Code for {country_code} is {result}")
# set country code for United States
country_code = "US"
# make the service call
result = client.service.CountryIntPhoneCode(
sCountryISOCode=country_code,
_soapheaders=[header_value]
)
# print the result
print(f"Phone Code for {country_code} is {result}")
print(response)
输出:
Phone Code for IN is 91
Phone Code for US is 1