Python Pillow – ImageDraw 模块
Python's Pillow 是已停产的Python Imaging Library (PIL) 的一个分支,它是一个强大的库,能够为您的Python代码添加图像处理功能。 Pillow 提供了许多模块,可以简化工作和修改图像的过程。
在本文中,我们将看看这个库的 ImageDraw 模块。顾名思义,ImageDraw 提供了多种在图像上绘制的方法。借助此模块,我们可以在图像上绘制线条、圆形、矩形,甚至书写和格式化文本。
在图像上绘制常见形状
我们将使用的图像可以使用 PIL 显示如下:
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to
# be used and displaying it
img = Image.open('img_path.png')
img.show()
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green rectangle
# in the middle of the image
draw.rectangle(xy = (50, 50, 150, 150),
fill = (0, 127, 0),
outline = (255, 255, 255),
width = 5)
# Method to display the modified image
img.show()
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green circle on the image
draw.circle(xy = (50, 50, 150, 150),
fill = (0, 127, 0),
outline = (255, 255, 255),
width = 5)
# Method to display the modified image
img.show()
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green vertical
# line in the middle image
draw.line(xy=(50, 150, 150, 50),
fill=(0, 128, 0), width = 5)
# Method to display the modified image
img.show()
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green diamond-shaped
# polygon in the middle of the image
draw.polygon(xy=(50, 150, 150, 50),
fill=(0, 128, 0),
outline=(255, 255, 255))
# Method to display the modified image
img.show()
Python
# Importing Image, ImageDraw and ImageFont
# from PIL
from PIL import Image, ImageDraw, ImageFont
# Opening the image to be used
img = Image.open('img_path.png')
# Creating an instance for
# the font to be used using ImageFont
# Here we pass the font name and
# the font size as arguments
fnt = ImageFont.truetype("Pillow/Tests/fonts/FreeMono.ttf", 20)
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing the text on the image
draw.text(xy=(25, 160),
text="Hello, Geeks!",
font=fnt,
fill=(0, 127, 0))
img.show()
输出
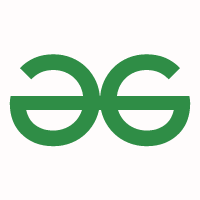
输出图像如下
我们可以通过首先创建一个 Draw 对象,使用 Draw 方法在 Image 上绘制形状和图形。
在图像上绘制一个矩形:
对于绘制矩形,我们使用 ImageDraw 模块的矩形绘制方法:
Syntax: ImageDraw.rectangle(xy, fill, outline, width)
The paramaters for this method are:
- xy : Corresponds to the tuple of set of points in the upper left corner and lower right coordinates enclosing your shape. The points are passed in a tuple as follows : (upper left x-coordinate, upper left y-coordinate, lower right x-coordinate, lower right y-coordinate)
- fill : Corresponds to the tuple of RGB colour values to fill the shape with.
- outline : Corresponds to the tuple of RGB colour values assigned for the shape’s boundary.
- width : Integer value corresponding to the thickness of the boundary of the shape.NOTE: The parameters are similar across various shape drawing methods.
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green rectangle
# in the middle of the image
draw.rectangle(xy = (50, 50, 150, 150),
fill = (0, 127, 0),
outline = (255, 255, 255),
width = 5)
# Method to display the modified image
img.show()
输出:
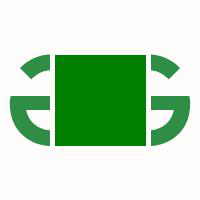
矩形法的输出图像
在图像上绘制椭圆(圆):
为了绘制椭圆形状,我们使用 ImageDraw 方法的椭圆方法:
Syntax: ImageDraw.ellipse(xy, fill, outline, width)
您将在 xy 中提供的坐标将充当一个框,其中将包含圆圈。
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green circle on the image
draw.circle(xy = (50, 50, 150, 150),
fill = (0, 127, 0),
outline = (255, 255, 255),
width = 5)
# Method to display the modified image
img.show()
输出:
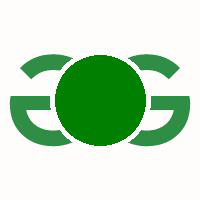
椭圆法的输出图像
在图像上画一条线:
为了绘制一条线,我们使用 ImageDraw 方法的 line 方法:
Syntax: lImageDraw.ine(xy, fill, width)
这里不考虑轮廓参数,宽度将决定线条的长度。
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green vertical
# line in the middle image
draw.line(xy=(50, 150, 150, 50),
fill=(0, 128, 0), width = 5)
# Method to display the modified image
img.show()
输出:
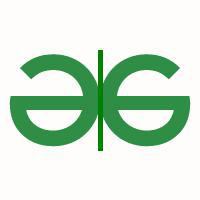
线法的输出图像
在图像上绘制多边形:
我们可以使用 ImageDraw 方法的多边形方法绘制所需形状的多边形:
Syntax: ImageDraw.polygon(xy, fill, outline)
xy 元组参数将包含基于您想要的形状边数的坐标。这里,宽度参数无效。
Python
# Importing Image and ImageDraw from PIL
from PIL import Image, ImageDraw
# Opening the image to be used
img = Image.open('img_path.png')
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing a green diamond-shaped
# polygon in the middle of the image
draw.polygon(xy=(50, 150, 150, 50),
fill=(0, 128, 0),
outline=(255, 255, 255))
# Method to display the modified image
img.show()
输出:
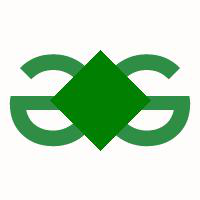
多边形法的输出图像
同样,我们可以使用这些方法绘制其他一些形状:
- 弧: ImageDraw.arc(xy,开始,结束,填充,宽度)
- 和弦(弓形): ImageDraw.chord(xy,开始,结束,填充,轮廓,宽度)
- 点: ImageDraw.point(xy,填充)
- Pieslice: ImageDraw.pieslice(xy,开始,结束,填充,轮廓,宽度)
start 和 end 参数对应顺时针方向的角度度数,将用一条线连接。
在图像上书写文字:
使用我们的 Draw 对象,我们还可以在图像上书写文本。可以使用 Text 方法完成:
Syntax: ImageDraw.text(xy, text, fill, font, anchor, spacing, align, direction, features, language, stroke_width, stroke_fill, embedded_color)
我们还将使用 PIL 中的 ImageFont 为我们的文本使用所需的字体。
Python
# Importing Image, ImageDraw and ImageFont
# from PIL
from PIL import Image, ImageDraw, ImageFont
# Opening the image to be used
img = Image.open('img_path.png')
# Creating an instance for
# the font to be used using ImageFont
# Here we pass the font name and
# the font size as arguments
fnt = ImageFont.truetype("Pillow/Tests/fonts/FreeMono.ttf", 20)
# Creating a Draw object
draw = ImageDraw.Draw(img)
# Drawing the text on the image
draw.text(xy=(25, 160),
text="Hello, Geeks!",
font=fnt,
fill=(0, 127, 0))
img.show()
输出:

文本方法的输出图像