Python SQLAlchemy – 动态获取列名
在本文中,我们将了解如何使用Python在 SQLAlchemy 中动态获取表的列名。
用于演示的数据库:
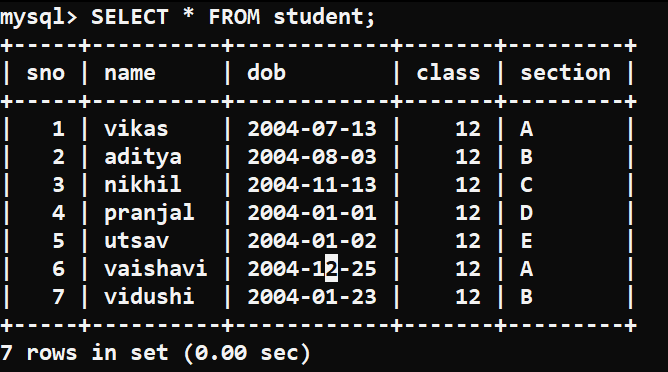
学生桌
因此,我们的学生表有 5 列,即 sno、name、dob、class 和 section,我们的任务是在Python代码中获取所有这些列名。
首先,我们将导入 sqlalchemy 模块,创建引擎,然后创建与数据库的连接。然后我们将对我们想要其列名的表执行查询。
示例 1:
现在使用通过运行查询获得的结果对象的 keys 方法,我们可以动态获取所有列名。
Python3
from sqlalchemy import create_engine
table_name = 'student'
engine = create_engine("mysql+pymysql://root:root123@localhost/geeksforgeeks")
connection = engine.connect()
result = connection.execute(f"SELECT * FROM {table_name}")
print(result.keys())
Python3
from sqlalchemy import create_engine
table_name = 'student'
engine = create_engine("mysql+pymysql://root:root123@localhost/geeksforgeeks")
connection = engine.connect()
result = connection.execute(f"SELECT * FROM {table_name}")
columns = []
for elem in result.cursor.description:
columns.append(elem[0])
print(columns)
输出:

上述代码的输出
示例 2:
我们也可以使用结果对象的result.cursor.description 。 result.cursor.description是一个包含元组的列表,其第一个元素是列的名称。让我们在它上面运行一个 for 循环并将它的第一个元素存储在我们的自定义列变量中。
Python3
from sqlalchemy import create_engine
table_name = 'student'
engine = create_engine("mysql+pymysql://root:root123@localhost/geeksforgeeks")
connection = engine.connect()
result = connection.execute(f"SELECT * FROM {table_name}")
columns = []
for elem in result.cursor.description:
columns.append(elem[0])
print(columns)

上述代码的输出