在 Julia 中使用日期和时间
Julia 提供了一个库来使用 Dates 来处理日期和时间。 Dates 模块是 Julia 内置的,我们只需要导入它就可以使用它。现在我们需要在每个函数前面加上一个明确的Dates类型,例如Dates.Date 。如果您不想在每个函数上添加前缀,只需在代码中添加using Dates ,然后您就可以使用 Dates 的任何函数,而无需使用 Dates 作为前缀。
Dates 模块提供用于处理日期和时间的耗材类。有许多函数可用于在 Julia 中操作日期和时间。
对于使用 Date , DateTime 提供了两个主要模块: Date 和 DateTime are most used 。它们都是抽象 TimeType 的子类型。
获取不同类型的日期
- Dates.Date(year, month, date):它返回带有提供参数的日期。它独立于时区。
- Dates.Date(year):它返回带有提供的年份的日期,月份,日期设置为 01
- Dates.Date(year, month):返回带有提供的年月的日期,日期设置为01。
- Dates.DateTime(year, month, date, hour, min, sec):它返回日期和时间,并指定确切的时间点。精确到毫秒。
- Dates.today():它提供当前日期的 Date 对象
- Dates.now():它及时返回当前实例的 DateTime 对象。
- Dates.now(Dates.UTC):它返回带有协调世界时 (UTC) 时区的日期。
例如:
Julia
# import Dates module
import Dates
# returns date with provided parameter and independent of time zone
d = Dates.Date(2001, 12, 12)
# returns a date with the provided year, and the month and day are set to 01
d = Dates.Date(2001)
# returns a date with the provided year and month, and the day is set to 01
d = Dates.Date(2001, 11)
# returns date and time and it specifies the exact moment in time. The accuracy is to a millisecond.
d = Dates.DateTime(2001, 10, 1, 12, 11, 11)
# returns the Date object for the current date
d = Dates.today()
# returns a DateTime object for the current instance in time
d = Dates.now()
# returns date with Coordinated Universal Time (UTC) time zone
d = Dates.now(Dates.UTC)
Julia
import Dates
# formatting a string into a DateTime()
Dates.DateTime("Tue, 11 Jan 2001 12:10:4", "e, d u y H:M:S")
Julia
import Dates
# Convert a string date to a d/m/yyyy format
Dates.Date("Mon, 12 Jan 2002", "e, d u y")
Julia
import Dates
# getting current time
my_time = Dates.now()
# using format() to format string into time
Dates.format(my_time, "e, dd u yyyy HH:MM:SS")
Julia
import Dates
my_time = Dates.DateTime("Mon, 1 Jun 2001 1:2:4", "e, d u y H:M:S")
# with leading zeros
Dates.format(my_time, "e: dd u yy, HH.MM.SS")
# without leading zero
Dates.format(my_time, "e: d u yy, H.M.S")
Julia
import Dates
# date that needs to be formatted
date = "Mon, 12 Jul 2001 12:13:14"
# using DateTime() to obtain DateTime() obj.
temp = Dates.DateTime(date, "e, dd u yyyy HH:MM:SS")
# Using Dates.format()
Dates.format(temp, "dd, U, yyyy HH:MM, e")
Julia
import Dates
# defining general format for dates
dateformat = Dates.DateFormat("y-m-d");
Dates.Date.([
"2001-11-01",
"2002-12-12",
"2003-02-6",
"2004-04-7",
"2005-12-9",
"2006-11-12"
], dateformat)
Julia
import Dates
# ISODateTimeFormat
Dates.Date.([
"2001-11-01",
"2002-12-12",
"2003-02-6",
"2004-04-7",
"2005-12-9",
"2006-11-12"
], Dates.ISODateTimeFormat)
Julia
import Dates
# creating a Dates object
t = Dates.now()
# Provides the current time
Dates.Time(t)
# Provides the hour of date object
Dates.hour(t)
# Provides the second of date object
Dates.second(t)
# Provides the minutes of date object
Dates.minute(t)
Julia
# provides unix time of current second
time()
Julia
# 1 year worth of UNIX time
Libc.strftime(86400 * 365.25 * 1)
Julia
import Dates
# To convert from unix to date/time object
Dates.unix2datetime(time())
以下代码的输出:
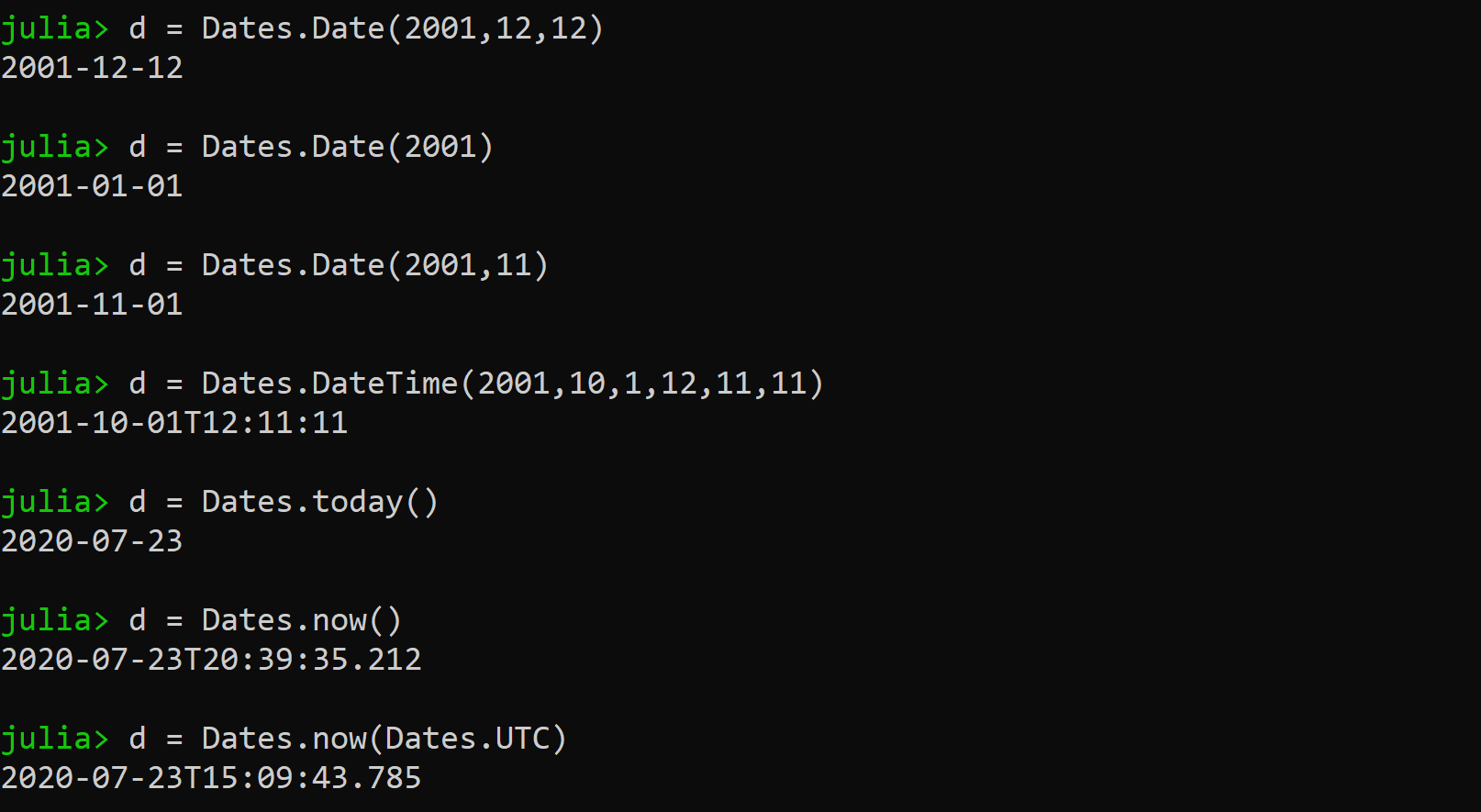
不同的日期类型
日期格式
为了格式化日期,我们需要引用代表/引用日期元素的字符。这是一个用于参考每个字符代表什么的表格。Code Reference Format Example y year digit yyyy or yy 2013 m month digit m 5 or 05 u month name u Feb U month full name U February e day of week e Mon E day of the week full E Monday d day in number d 1 H hour digit HH 11 M minute digit MM 22 S second digit S 00 s millisecond digit s .001
我们可以使用DateTime()、Dates.format()和Date()等函数来格式化这些字符串。下面的示例将展示各种日期格式化方法:
- 使用日期时间()
朱莉娅
import Dates
# formatting a string into a DateTime()
Dates.DateTime("Tue, 11 Jan 2001 12:10:4", "e, d u y H:M:S")

日期时间示例
- 使用日期()
朱莉娅
import Dates
# Convert a string date to a d/m/yyyy format
Dates.Date("Mon, 12 Jan 2002", "e, d u y")

Date_Example
- 使用格式()
朱莉娅
import Dates
# getting current time
my_time = Dates.now()
# using format() to format string into time
Dates.format(my_time, "e, dd u yyyy HH:MM:SS")

格式示例
- 有时我们需要单个日期元素的前导零。这个例子展示了我们如何添加前导零。
朱莉娅
import Dates
my_time = Dates.DateTime("Mon, 1 Jun 2001 1:2:4", "e, d u y H:M:S")
# with leading zeros
Dates.format(my_time, "e: dd u yy, HH.MM.SS")
# without leading zero
Dates.format(my_time, "e: d u yy, H.M.S")
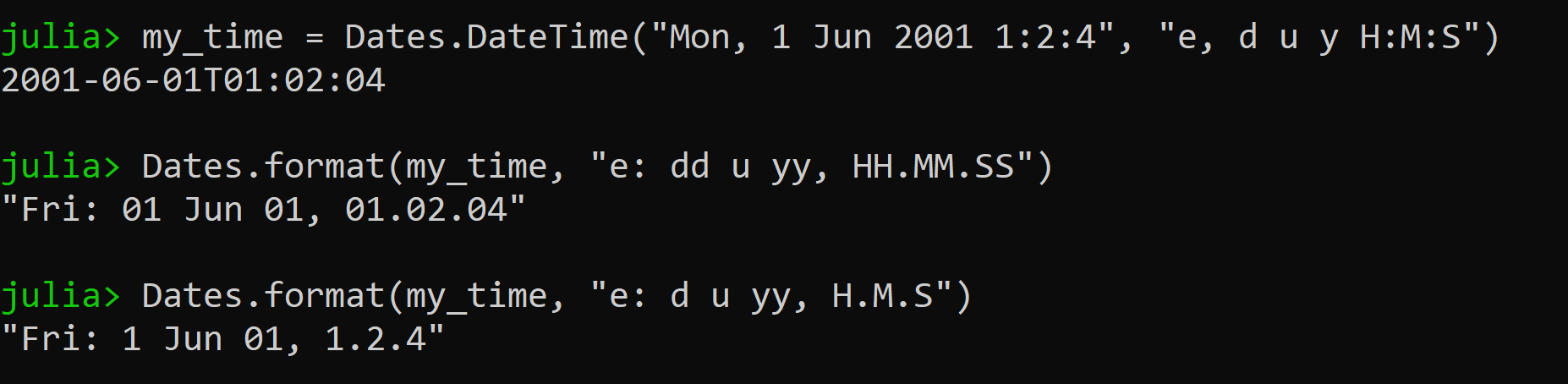
领先的零示例
- 此外,我们可以将日期字符串从一种格式转换为另一种格式,方法是使用DateTime()转换为 DateTime 对象,然后使用DateFormat()显示/输出为不同的格式。
朱莉娅
import Dates
# date that needs to be formatted
date = "Mon, 12 Jul 2001 12:13:14"
# using DateTime() to obtain DateTime() obj.
temp = Dates.DateTime(date, "e, dd u yyyy HH:MM:SS")
# Using Dates.format()
Dates.format(temp, "dd, U, yyyy HH:MM, e")
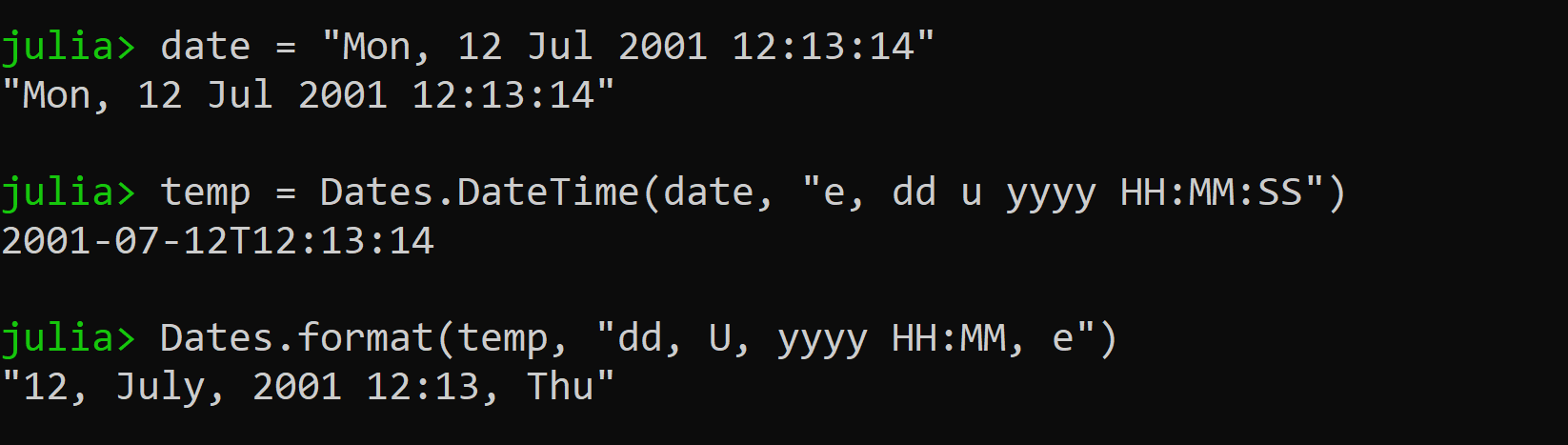
one_form_to_another
- 当我们有很多日期要处理时,我们使用DateFormat对象来处理字符串日期数组
朱莉娅
import Dates
# defining general format for dates
dateformat = Dates.DateFormat("y-m-d");
Dates.Date.([
"2001-11-01",
"2002-12-12",
"2003-02-6",
"2004-04-7",
"2005-12-9",
"2006-11-12"
], dateformat)
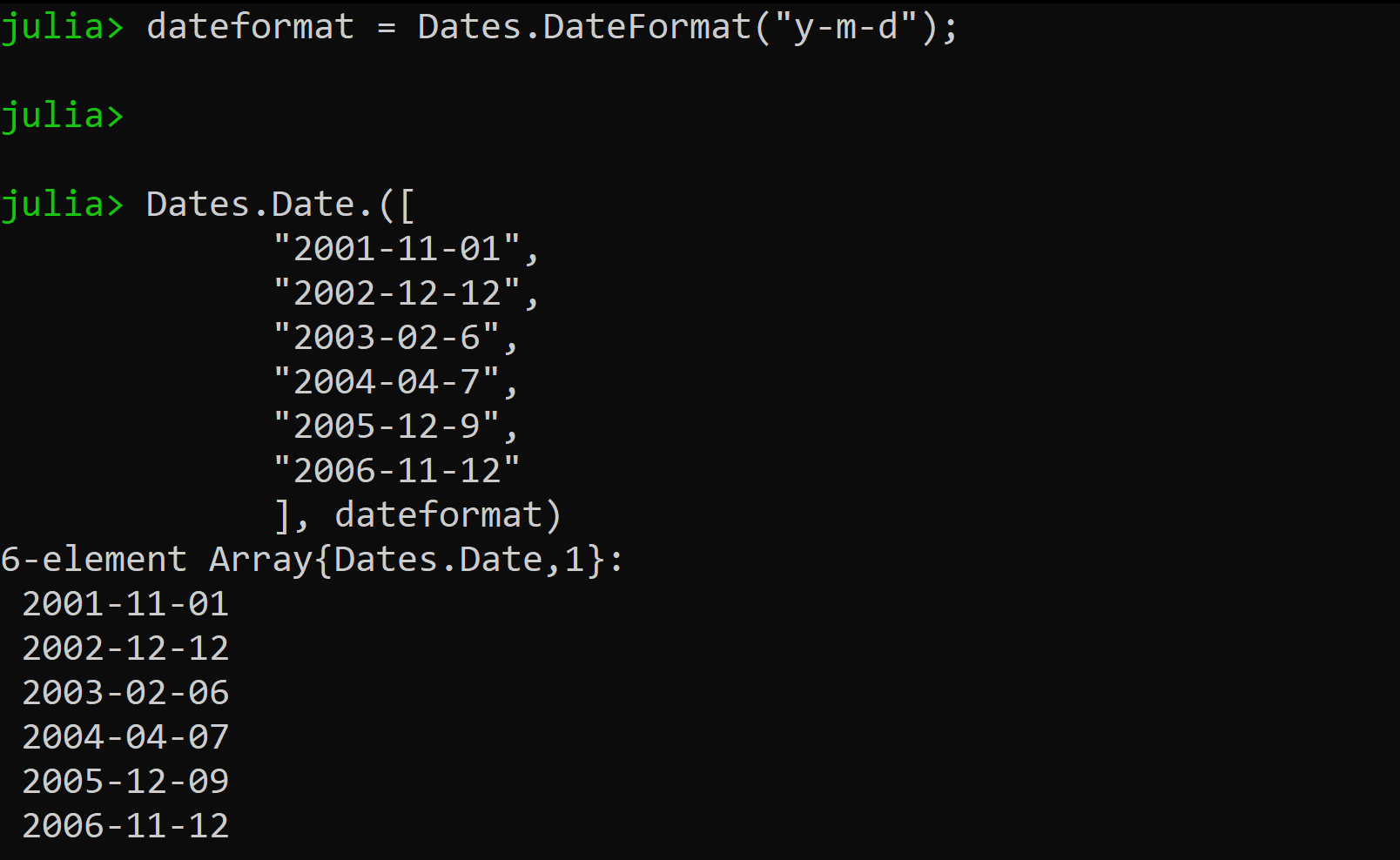
array_of_dates
- 我们还可以使用提供 ISO8601 格式的Dates.ISODateTimeFormat 。示例如下:
朱莉娅
import Dates
# ISODateTimeFormat
Dates.Date.([
"2001-11-01",
"2002-12-12",
"2003-02-6",
"2004-04-7",
"2005-12-9",
"2006-11-12"
], Dates.ISODateTimeFormat)
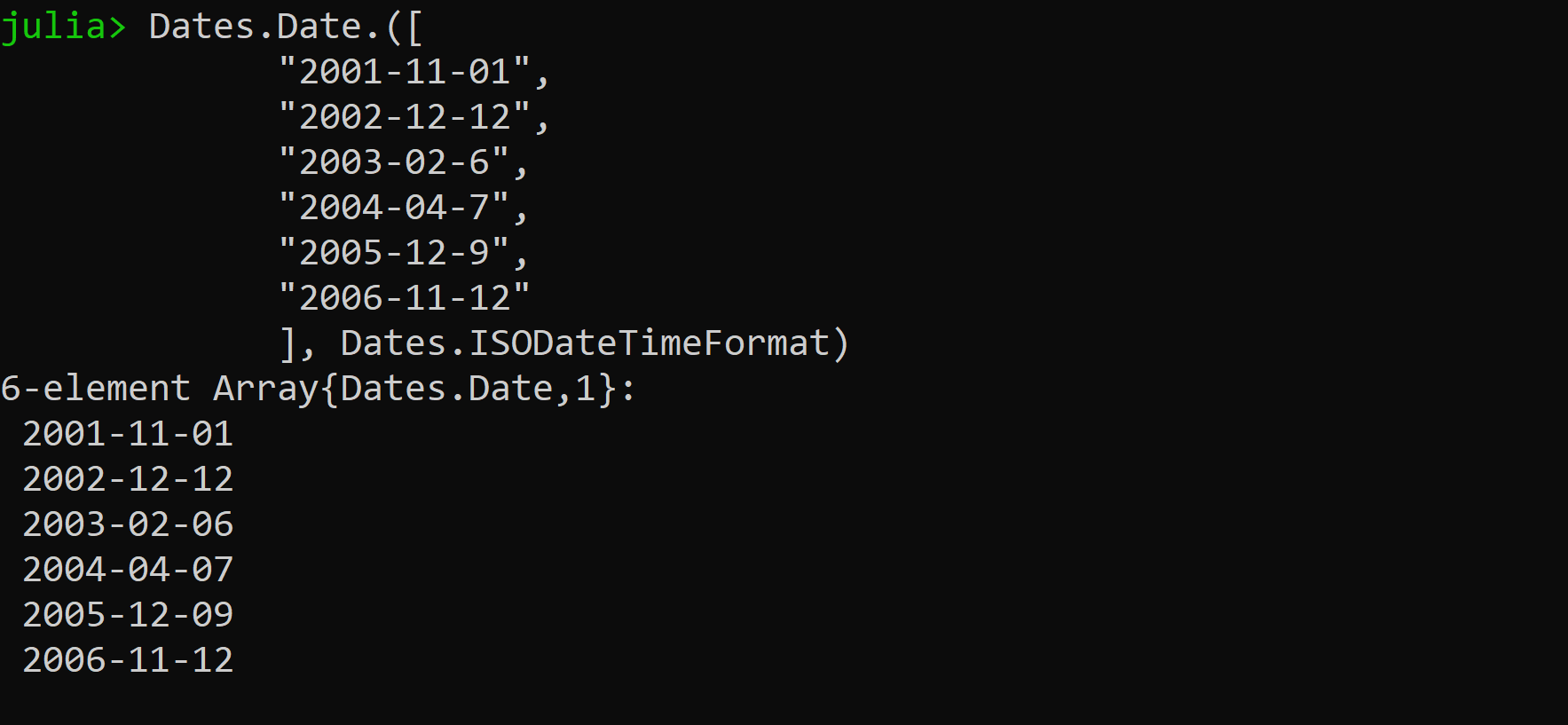
ISOFORMAT_format_example
获得不同类型的时间
为了在 Julia 中获取时间,我们使用Dates.Time()函数 大多。它遵循预测的公历。不同类型的时间如下:
- Dates.Time(Dates.now())它以 HH:MM:SS:ss 形式返回一个时间,并采用 Date 对象。
- Dates.minute(t)它给出了 Dates.Time 对象传递的分钟。
- Dates.hour(t)它给出了 Dates.Time 对象传递的时间。
- Dates.second(t)它给出了传递的 Dates.Time 对象的第二个。
朱莉娅
import Dates
# creating a Dates object
t = Dates.now()
# Provides the current time
Dates.Time(t)
# Provides the hour of date object
Dates.hour(t)
# Provides the second of date object
Dates.second(t)
# Provides the minutes of date object
Dates.minute(t)
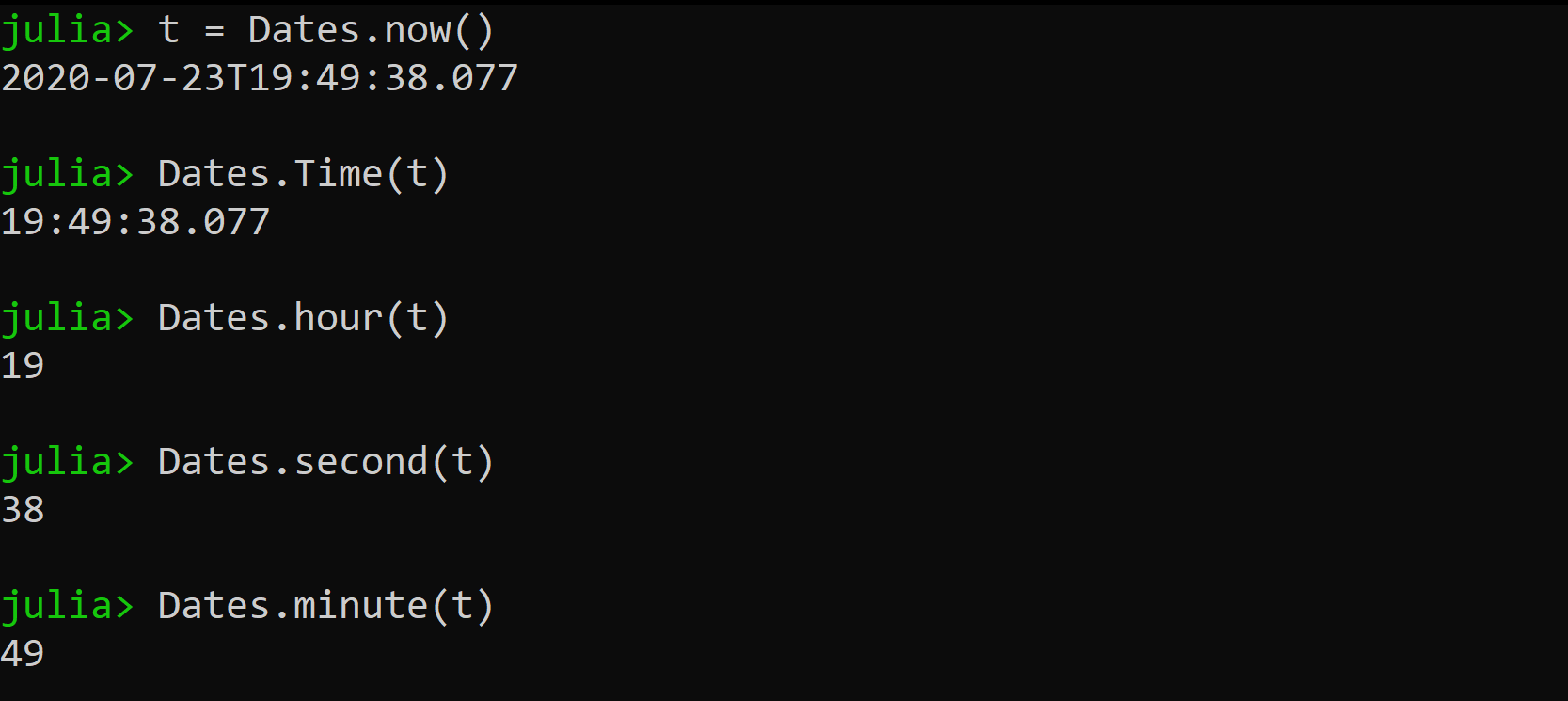
time_module_example
纪元时间
它也称为UNIX时间。它用于处理计时。它是自 1970 年初以来经过的秒数。
- time()函数在不带任何参数使用时,返回当前秒的 Unix/Epoch 时间值。
朱莉娅
# provides unix time of current second
time()

时间()_输出
- 为了将 UNIX 时间转换为更易读的形式,我们使用strftime() (“字符串格式时间”)函数。
朱莉娅
# 1 year worth of UNIX time
Libc.strftime(86400 * 365.25 * 1)

strftime() 示例
- 函数unix2datetime() 将 UNIX 时间转换为日期/时间对象。
朱莉娅
import Dates
# To convert from unix to date/time object
Dates.unix2datetime(time())

unix2datetime() 示例
还有许多其他函数可用于操作日期和时间。请参阅官方文档以了解有关不同实现的更多信息。