Python - 列出带有扩展名的目录中的文件
在本文中,我们将讨论不同的用例,在这些用例中,我们希望使用Python列出目录中存在的文件及其扩展名。
使用的模块
- os: Python的 OS 模块提供与操作系统交互的功能。
- glob:在Python,glob 模块用于检索匹配指定模式的文件/路径名。 glob 的模式规则遵循标准的 Unix 路径扩展规则。还预测,根据基准,它比其他方法更快地匹配目录中的路径名。
使用的目录结构:
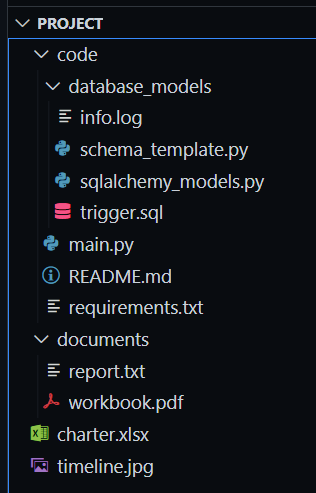
目录结构根视图
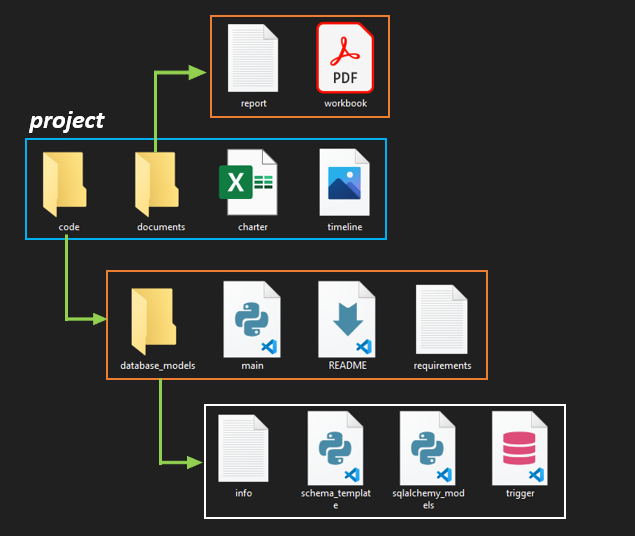
目录文件可视化表示
方法一:使用`os`模块
该模块提供了一种使用操作系统相关功能的可移植方式。方法os.listdir()列出目录中存在的所有文件。如果我们也想使用子目录,我们可以使用os.walk() 。
句法:
os.listdir(path = ‘.’)
返回包含路径给定目录中条目名称的列表。
句法:
os.walk(top, topdown=True, onerror=None, followlinks=False)
通过自顶向下或自底向上遍历树来生成目录树中的文件名。
示例 1:列出root/home/project 中存在的文件和目录
Python
import os
# To get directories as well as files present
# in a path
list_1 = os.listdir(path=r"root/home/project")
print(list_1)
# To get only files present in a path
list_2 = os.listdir(path=r"root/home/project")
# Loop through each value in the list_2
for val in list_2:
# Remove the value from list_2 if the "." is
# not present in value
if "." not in val:
list_2.remove(val)
print(list_2)
Python
import os
all_files = list()
all_dirs = list()
# Iterate for each dict object in os.walk()
for root, dirs, files in os.walk("root/home/project"):
# Add the files list to the the all_files list
all_files.extend(files)
# Add the dirs list to the all_dirs list
all_dirs.extend(dirs)
print(all_files)
print(all_dirs)
Python
import glob
list_ = glob.glob(r"root/home/project/code/*")
print(list_)
Python
import glob
list_ = glob.glob(r"root/home/project/code/database_models/*.py")
print(list_)
输出 :
['documents', 'code', 'charter.xlsx', 'timeline.jpg']
['charter.xlsx', 'timeline.jpg']
示例 2:列出root/home/project 中存在的所有子目录和子文件
Python
import os
all_files = list()
all_dirs = list()
# Iterate for each dict object in os.walk()
for root, dirs, files in os.walk("root/home/project"):
# Add the files list to the the all_files list
all_files.extend(files)
# Add the dirs list to the all_dirs list
all_dirs.extend(dirs)
print(all_files)
print(all_dirs)
输出:
[‘charter.xlsx’, ‘timeline.jpg’, ‘report.txt’, ‘workbook.pdf’, ‘trigger.sql’, ‘schema_template.py’, ‘sqlalchemy_models.py’, ‘info.log’, ‘README.md’, ‘requirements.txt’, ‘main.py’]
[‘documents’, ‘code’, ‘database_models’]
方法 2:使用 `glob` 模块
glob 模块根据 Unix shell 使用的规则查找与指定模式匹配的所有路径名。我们将使用glob.glob()函数来完成我们的任务。 Unix shell-like 背后的想法意味着我们可以提供 Unix shell-like 模式来搜索文件。
句法:
glob.glob(pathname, *, recursive=False)
返回与路径名匹配的路径名列表,该列表必须是包含路径规范的字符串。
' * ' 表示它将匹配类似于os.listdir()方法返回的所有项目。
示例1:获取root/home/project/code中的所有目录和文件
Python
import glob
list_ = glob.glob(r"root/home/project/code/*")
print(list_)
输出:
[‘database_models’, ‘README.md’, ‘requirements.txt’, ‘main.py’]
示例 2:获取root/home/project/code/database_models 中的所有Python (.py) 文件
Python
import glob
list_ = glob.glob(r"root/home/project/code/database_models/*.py")
print(list_)
输出:
[‘schema_template.py’, ‘sqlalchemy_models.py’]